How to add multiple columns to pandas dataframe in one assignment
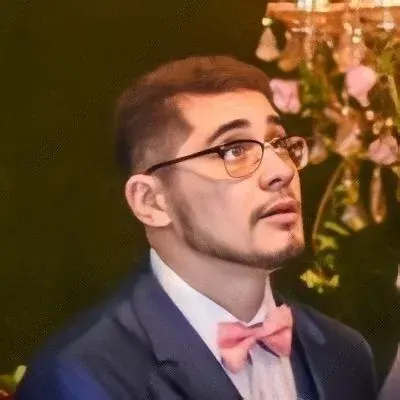
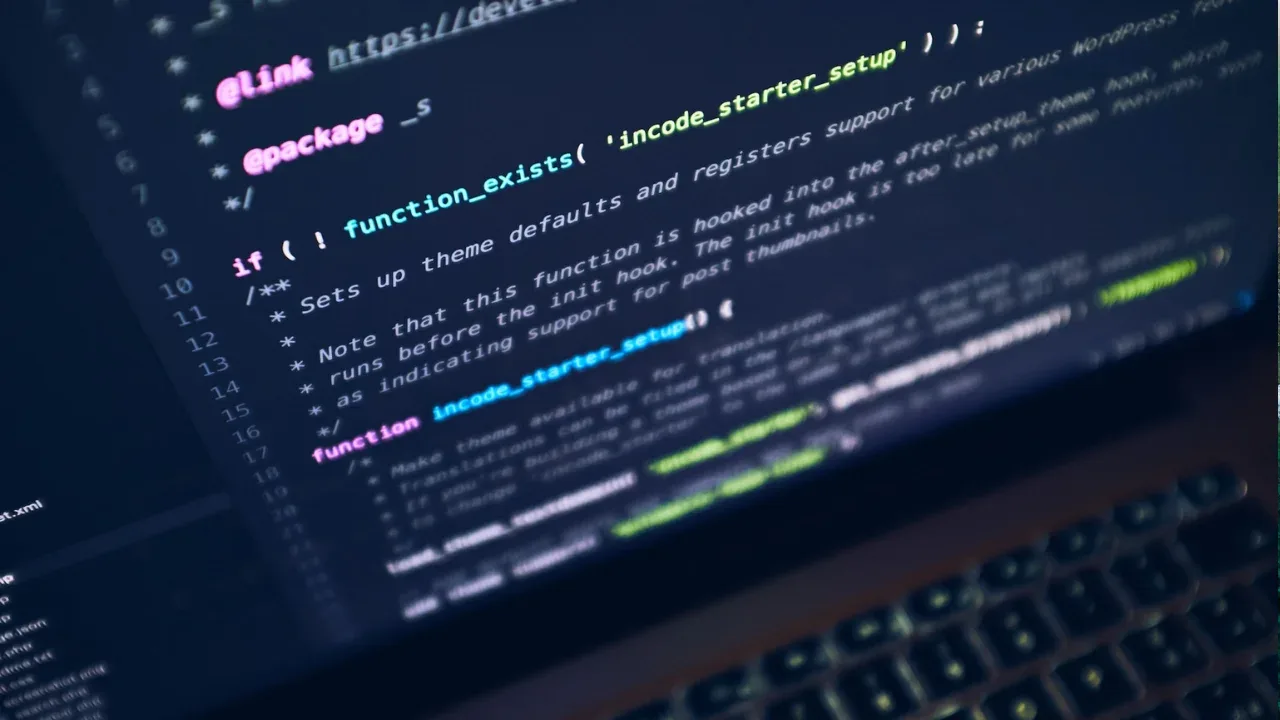
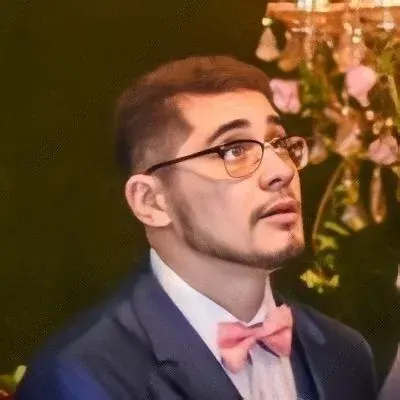
How to Add Multiple Columns to Pandas DataFrame in One Assignment ππͺ
So you want to add multiple columns to a Pandas DataFrame in just one assignment, huh? I feel you! π Doing it in one step can save you time and make your code cleaner. In this guide, I'll show you how to achieve this powerful feat and tackle any common issues that may arise. Let's dive right in! π
The Scenario π
Here's the context: You have a DataFrame with existing columns col_1
and col_2
and you want to add new columns column_new_1
, column_new_2
, and column_new_3
all at once. Here's the initial code:
import pandas as pd
data = {'col_1': [0, 1, 2, 3],
'col_2': [4, 5, 6, 7]}
df = pd.DataFrame(data)
You then attempted to add the new columns in one go using the following line of code:
df[['column_new_1', 'column_new_2', 'column_new_3']] = [np.nan, 'dogs', 3]
But, alas, it didn't work as expected! π Let's dig into the issue and find a solution!
The Pitfall β οΈ
The reason the above code didn't work as expected is that you assigned a list to multiple columns simultaneously. Pandas tries to match each element in the list to the corresponding column, but since the list you provided has three elements while you have only two columns, you get a ValueError
stating that the number of items doesn't match the number of columns.
The Solution π‘
To add multiple columns in one assignment, you need to provide a DataFrame with the desired new columns and their values. Here's the correct way to achieve this:
import pandas as pd
import numpy as np
data = {'col_1': [0, 1, 2, 3],
'col_2': [4, 5, 6, 7]}
df = pd.DataFrame(data)
# Create a new DataFrame with the new columns and their values
new_data = {'column_new_1': [np.nan, np.nan, np.nan, np.nan],
'column_new_2': ['dogs', 'dogs', 'dogs', 'dogs'],
'column_new_3': [3, 3, 3, 3]}
new_columns_df = pd.DataFrame(new_data)
# Concatenate the original DataFrame with the new columns DataFrame
df = pd.concat([df, new_columns_df], axis=1)
By using the pd.concat()
function, we merged the original DataFrame df
with the new columns DataFrame new_columns_df
along the columns (axis=1
). This seamlessly adds the new columns to the existing DataFrame, all in one assignment. Brilliant! π
One Step Closer to DataFrame Mastery! π©βπ»π
You've just learned how to add multiple columns to a Pandas DataFrame in one go! Now you can save time and write cleaner code by eliminating those repeated steps. So go forth and conquer your data manipulation tasks with confidence! πͺ
If you have any questions or face any issues, feel free to drop a comment below. Let's rock the Pandas world together! πΌπ
Remember, sharing is caring! If you found this guide helpful, don't hesitate to share it with your friends or colleagues who might be struggling with the same issue. Everyone deserves the power of one-step column additions! π
Happy coding! β¨π