How to add header row to a pandas DataFrame
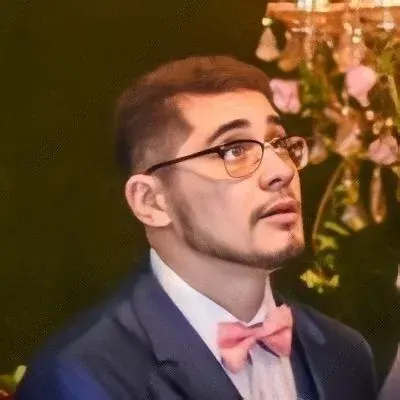
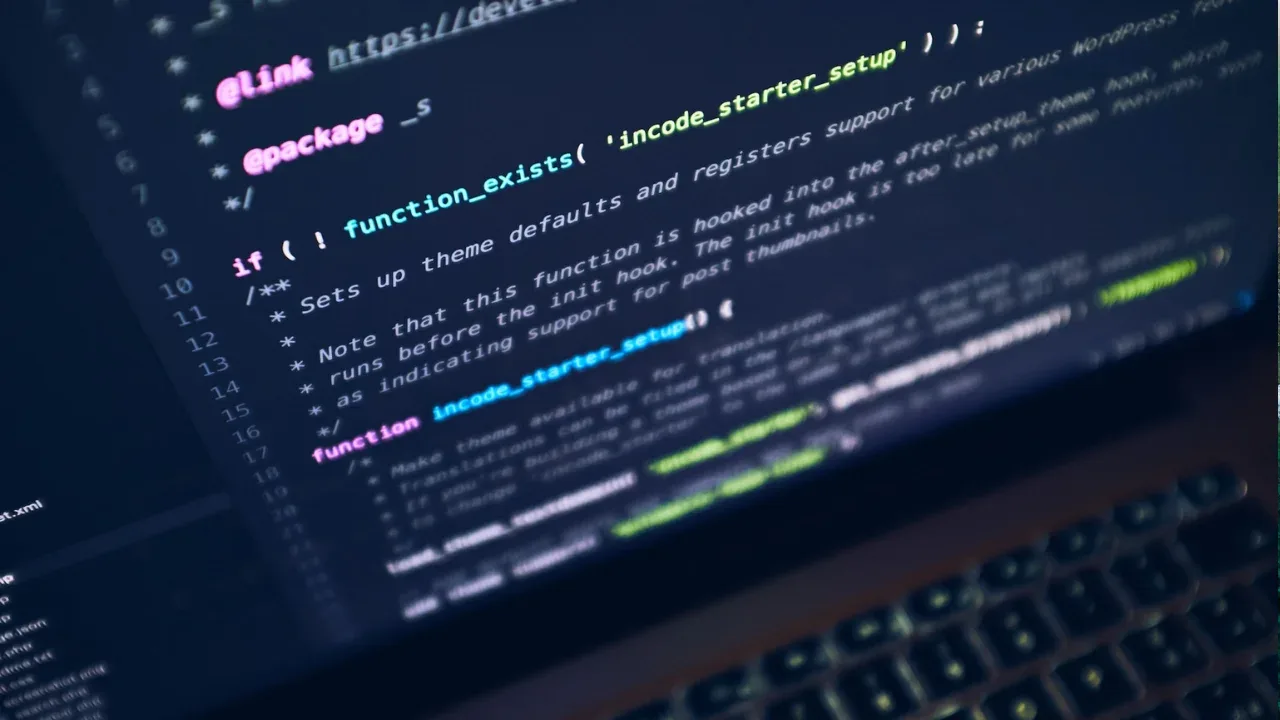
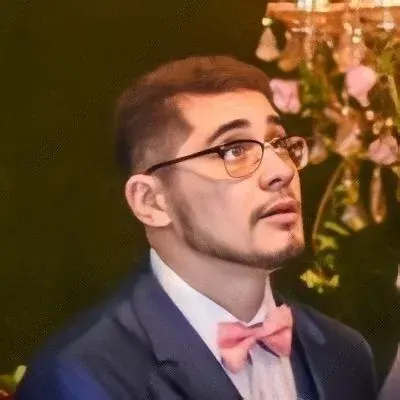
๐๐ป How to Add a Header Row to a Pandas DataFrame ๐งพ
Do you have a CSV file with data, but it doesn't have a header row? ๐ซ No worries! In this blog post, we'll navigate through this common issue and provide you with easy solutions to add a header row to your pandas DataFrame. Let's get started! ๐
The Problem: Missing Header Row ๐
So, you have a CSV file with several columns and rows, but it lacks that important header row. This header row helps us identify and manipulate data with ease. But fear not, we're here to help! Below is a code snippet similar to what you've tried:
Cov = pd.read_csv("path/to/file.txt", sep='\t')
Frame = pd.DataFrame([Cov], columns=["Sequence", "Start", "End", "Coverage"])
Frame.to_csv("path/to/file.txt", sep='\t')
The Error: "ValueError: Shape of Passed Values" ๐ฉ
๐ค So, you ran the code and encountered the dreaded error message:
ValueError: Shape of passed values is (1, 1), indices imply (4, 1)
What does this error mean? Well, it's telling you that the shape of the DataFrame you're trying to save doesn't match the expected shape. In our case, you're passing a DataFrame with a shape of (1, 1) instead of the expected (4, 1).
The Solution: Easy Steps to Add a Header Row ๐
To add a header row to your pandas DataFrame, follow these simple steps:
Read the CSV file without a header row using the
header
parameter set toNone
:df = pd.read_csv("path/to/file.txt", sep='\t', header=None)
Rename the columns of the DataFrame using the
columns
attribute:df.columns = ["Sequence", "Start", "End", "Coverage"]
Save the DataFrame back to the CSV file:
df.to_csv("path/to/file.txt", sep='\t', index=False)
That's it! You've successfully added a header row to your DataFrame. ๐
Wrapping Up and Taking Action ๐
Now that you know how to add a header row to a pandas DataFrame, go ahead and apply this knowledge to your own projects and datasets. You'll find it incredibly useful for data analysis, manipulation, and visualization.
Got any other pandas-related questions or data-related dilemmas? Let us know in the comments below, and we'll be happy to help! ๐
Keep exploring, keep coding! ๐ช๐
References: