How slicing in Python works
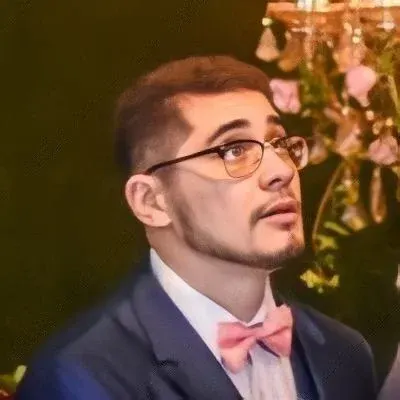

Slicing in Python: Unraveling the Mystery 😮🔪
Have you ever come across a puzzling slice notation in Python, like a[x:y:z]
, a[:]
, or a[::2]
, and wondered how it works to extract elements from a list or string? 🤔 You're not alone! In this blog post, we'll dive into the fascinating world of slicing and demystify its inner workings. 💪
Understanding the Basics 📚
Before we unravel the intricacies of slice notation, let's quickly recap what slicing is in Python. Slicing allows us to extract a portion (or a slice) of a list, tuple, or string based on a specified range or step. It's a powerful feature that can save you loads of time and effort when working with collections.
Breaking Down the Notation 📝
The basic syntax for slicing is start:stop:step
. Each component can be omitted, and they default to the following values:
start
defaults to the beginning of the sequence.stop
defaults to the end of the sequence.step
defaults to 1 (extract every element).
To better grasp the concept, let's explore some examples:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# Extracts elements from index 1 to 4 (exclusive)
slice_1 = numbers[1:4] # Returns [2, 3, 4]
# Extracts every second element
slice_2 = numbers[::2] # Returns [1, 3, 5, 7, 9]
# Extracts elements in reverse order
slice_3 = numbers[::-1] # Returns [10, 9, 8, 7, 6, 5, 4, 3, 2, 1]
# Extracts a copy of the entire list
slice_4 = numbers[:] # Returns [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
As you can see, slice notation provides a concise way to extract specific segments or patterns from a sequence.
What Gets Included in the Slice? 🕵️♂️
Determining which elements end up in the slice can be a bit tricky, especially when using negative indices or stepping through the sequence in reverse. Let's break it down:
When
start
is provided, the slice includes elements starting from that index.When
stop
is provided, the slice stops at the index before that element. It's an exclusive bound.When
step
is provided, the slice extracts elements at regular intervals based on the step value.
To make it easier, let's analyze the examples we saw earlier:
For
slice_1 = numbers[1:4]
, the slice includes elements at indices 1, 2, and 3 (but not 4).For
slice_2 = numbers[::2]
, the slice contains elements at every second index: 0, 2, 4, 6, and 8.For
slice_3 = numbers[::-1]
, the slice reverses the order of the list.For
slice_4 = numbers[:]
, the slice simply creates a copy of the entire list.
Remember, slice notation is incredibly flexible, allowing you to tweak the start
, stop
, and step
values to suit your needs. 🧙♂️
Additional Resources 📚
If you're interested in exploring the design decisions behind slice notation, check out this insightful discussion on Stack Overflow.
For practical usage of slicing and retrieving every Nth element from a list, take a look at this helpful Stack Overflow thread. It covers various approaches to solving the problem.
If you want to dig deeper into slice assignment (modifying a list slice), this Stack Overflow post provides more specific answers.
Your Turn to Slice! 🍕
Now that you've grasped the fundamentals of slicing in Python and how the slice notation works, it's time to put your newfound knowledge into action! Experiment with different slices, play around with negative indices, and explore creative ways to harness the power of slicing. 🚀
If you have any questions or want to share your slicing tips and tricks, drop a comment below! Let's enhance our slicing skills together! Happy coding! 😄💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
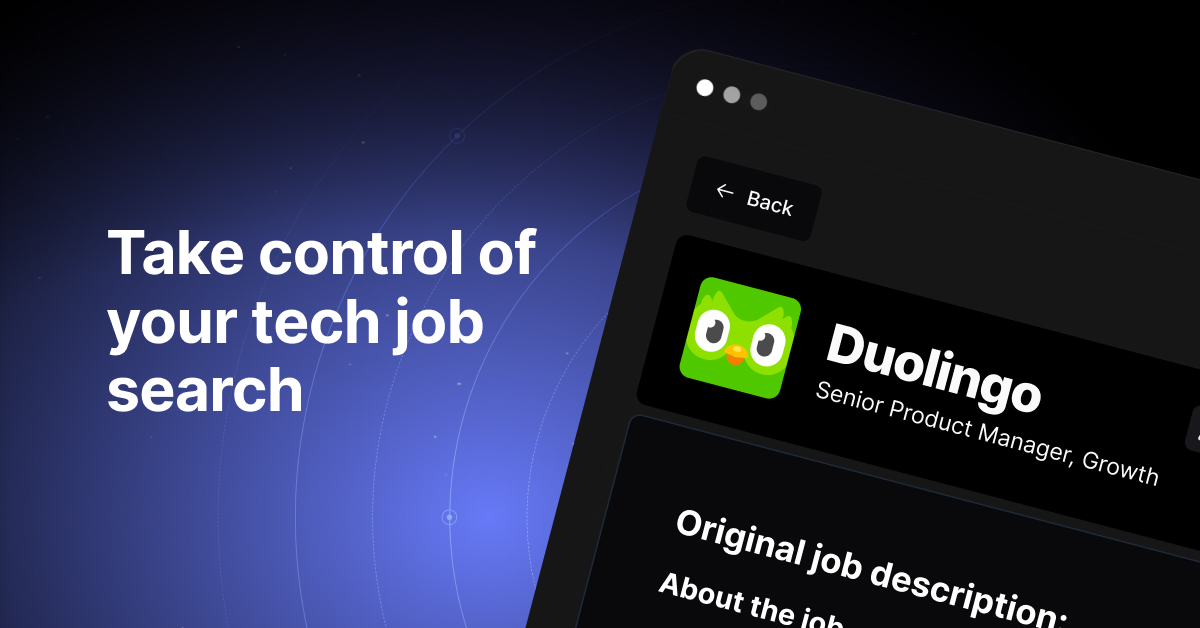