How exactly do Django content types work?
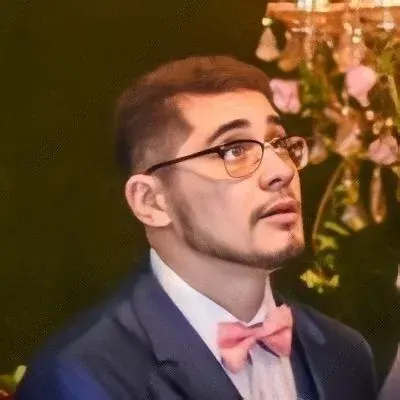
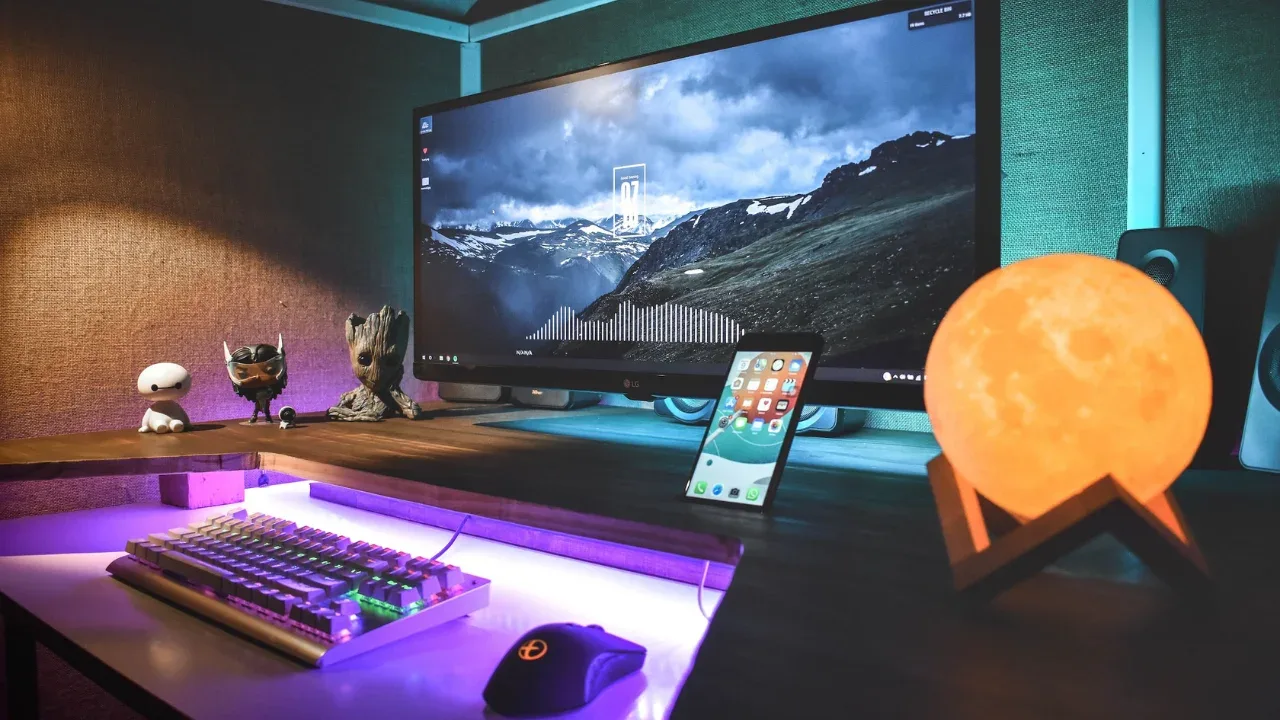
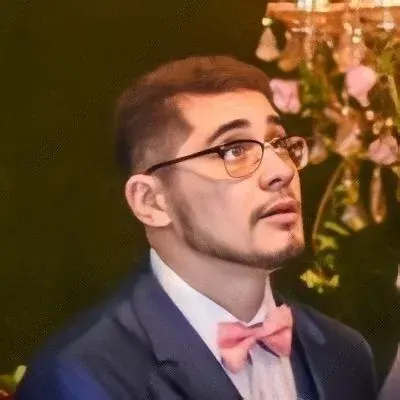
How exactly do Django content types work? 🤔
If you've ever found yourself scratching your head over Django's content types, you're not alone! But fear not, because in this blog post, we're going to break down the concept of Django content types in a practical and easy-to-understand way. 🚀
Understanding Django Content Types
First, let's get a basic understanding of what content types are in Django. At its core, a content type is a way to define models that can be generic across multiple objects. It allows you to create dynamic models without the need for changing your database schema. 🗃️
One common use case for content types is when you need to implement generic relationships between models. For example, consider a scenario where you have models for a BlogPost and a Comment. With content types, you can create a relationship between any model and the Comment model without specifically defining that relationship in the models themselves. Pretty neat, huh? 🎩
Practical Example: Blog Post and Comment Models
Let's dive into a practical real-world example to help illustrate how content types work. Imagine you have the following models in your Django application:
from django.contrib.contenttypes.fields import GenericForeignKey
from django.contrib.contenttypes.models import ContentType
from django.db import models
class BlogPost(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
class Comment(models.Model):
content_type = models.ForeignKey(ContentType, on_delete=models.CASCADE)
object_id = models.PositiveIntegerField()
content_object = GenericForeignKey('content_type', 'object_id')
text = models.TextField()
In this example, we have a BlogPost model and a Comment model. The Comment model has a ForeignKey to ContentType and uses a GenericForeignKey to establish a relationship with any model in your Django application.
Implementing Content Types in Django
To create a comment for a specific blog post using content types, follow these steps:
Get the content type of the BlogPost model:
blog_post_type = ContentType.objects.get_for_model(BlogPost)
Create the BlogPost object:
blog_post = BlogPost.objects.create(title="My First Blog Post", content="Lorem ipsum dolor sit amet.")
Create the Comment object:
comment = Comment.objects.create(content_type=blog_post_type, object_id=blog_post.id, text="Great post!")
And that's it! You've successfully created a comment for a specific blog post using content types. By utilizing content types, you have the flexibility to create generic relationships between models, making your application more dynamic and adaptable. 🌈
Wrapping Up
Django content types may initially seem a bit bewildering, but with a practical example and straightforward implementation steps, I hope this blog post has shed some light on how they work. Remember, content types allow you to create generic relationships between models, opening up a whole new world of possibilities for your Django applications. 💡
If you still have any questions or want to share your experiences with Django content types, I'd love to hear from you in the comments below! Let's continue the conversation and unravel even more mysteries together. Happy coding! 💻🚀
Read more: Check out our previous blog posts on Django tips and tricks, and be sure to subscribe to our newsletter for regular updates and exclusive content. Don't miss out! 😉📧