How does the @property decorator work in Python?
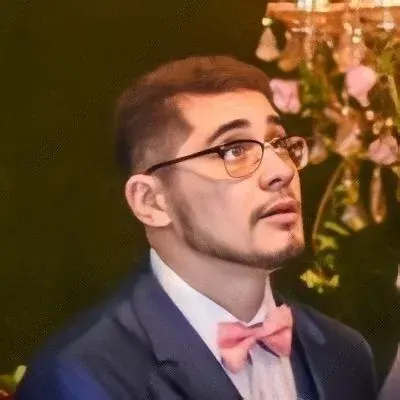
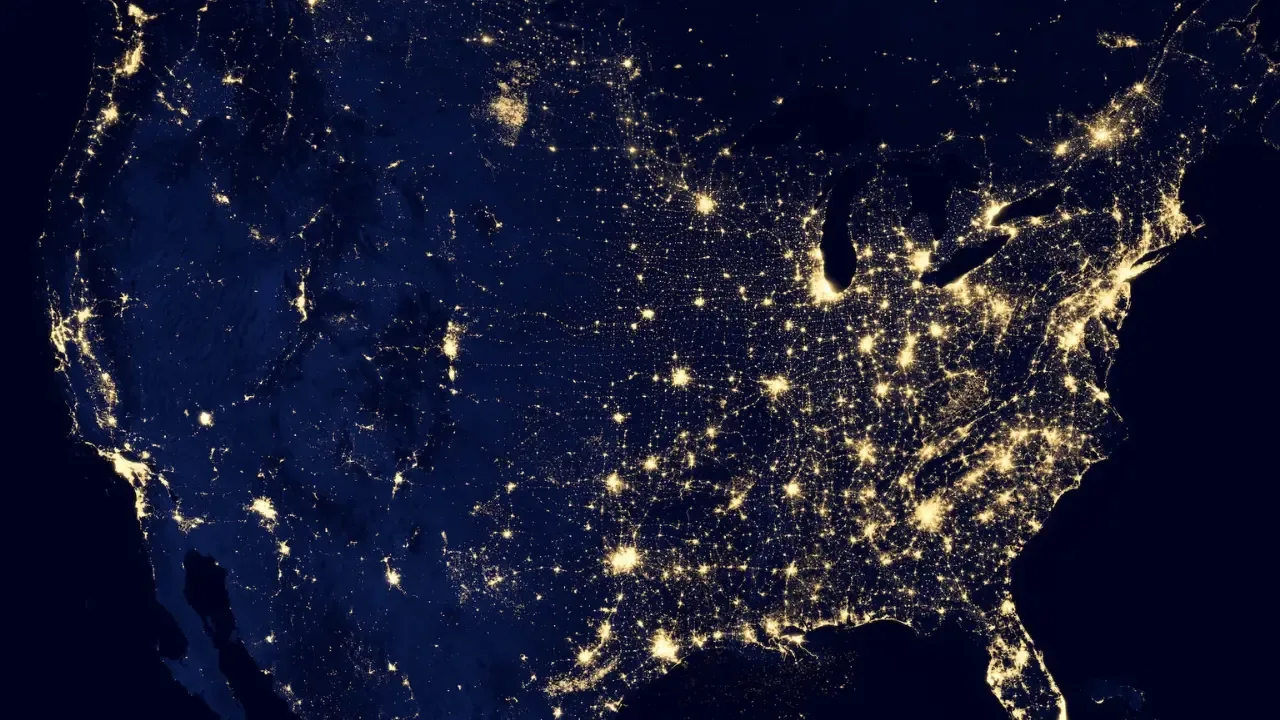
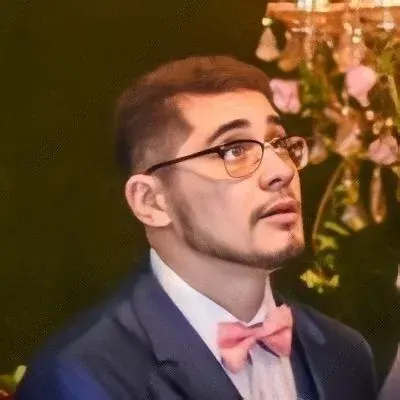
Understanding the Magic Behind the @property Decorator in Python 🧙♂️
Have you ever come across the @property
decorator in Python and wondered how it actually works? 🤔 It's a powerful tool that allows you to define specific behaviors for attribute access, like getters, setters, and deleters, all in a clean and concise way. But how does it do all that magic? Let's dive in and find out! 💡
Declaring Properties the Traditional Way 👨🏫
Before we explore the wonders of the @property
decorator, let's first understand how properties can be declared the traditional way. The following example comes straight from the Python documentation:
class C:
def __init__(self):
self._x = None
def getx(self):
return self._x
def setx(self, value):
self._x = value
def delx(self):
del self._x
x = property(getx, setx, delx, "I'm the 'x' property.")
In this example, the property
function is used with the getx
, setx
, delx
, and a docstring as arguments. This creates a property called x
with custom getter (getx
), setter (setx
), and deleter (delx
) methods.
Simplifying with the @property Decorator 🎉
Now, let's explore how the @property
decorator simplifies this process and makes our code more readable. Take a look at the following example:
class C:
def __init__(self):
self._x = None
@property
def x(self):
"""I'm the 'x' property."""
return self._x
@x.setter
def x(self, value):
self._x = value
@x.deleter
def x(self):
del self._x
In this example, we've eliminated the need for the property
function and declared the x
attribute as a property directly using the @property
decorator.
But what about the setter and deleter methods? How are they attached to the x
property? Here comes the magic! 😎
When using the @property
decorator, additional decorators called @x.setter
and @x.deleter
are automatically created. These decorators allow us to define the setter and deleter methods separately, making our code more organized and easier to understand.
Giving the @property Decorator a Closer Look 🔬
Underneath the hood, the @property
decorator works by implementing the descriptor protocol. Don't be scared by the fancy term! It simply means that the decorator enables customizing attribute access on an object.
The @property
decorator transforms the x
method into a data descriptor, which allows it to intercept access to the x
attribute. When we use @x.setter
and @x.deleter
, they are also modifying the descriptor behavior to intercept attribute assignment and deletion accordingly.
By leveraging the power of descriptors, the @property
decorator enables us to define the behavior of properties with just a few lines of code. It's like having a magic wand for attribute access! ✨
Now It's Your Turn! 💪
Congratulations on making it this far! Now that you understand how the @property
decorator works in Python, it's time to put your newfound knowledge into practice. Go ahead and try using the decorator in your code to create clean and organized property access.
Remember, with great decorator power comes great responsibility! 😄 So, use the @property
decorator wisely, sparingly, and only when it makes sense to define specific behavior for attribute access.
If you have any questions or want to share some cool ways you've used the @property
decorator, leave a comment below! Let's keep the conversation going and continue exploring the wonders of Python together. 🐍💻
📚 Learn More
Python Documentation: property - Dive deeper into the official Python documentation to learn more about the
property
function and how to use it effectively.