How does Python"s super() work with multiple inheritance?
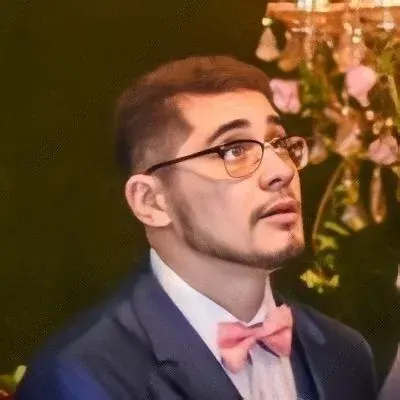
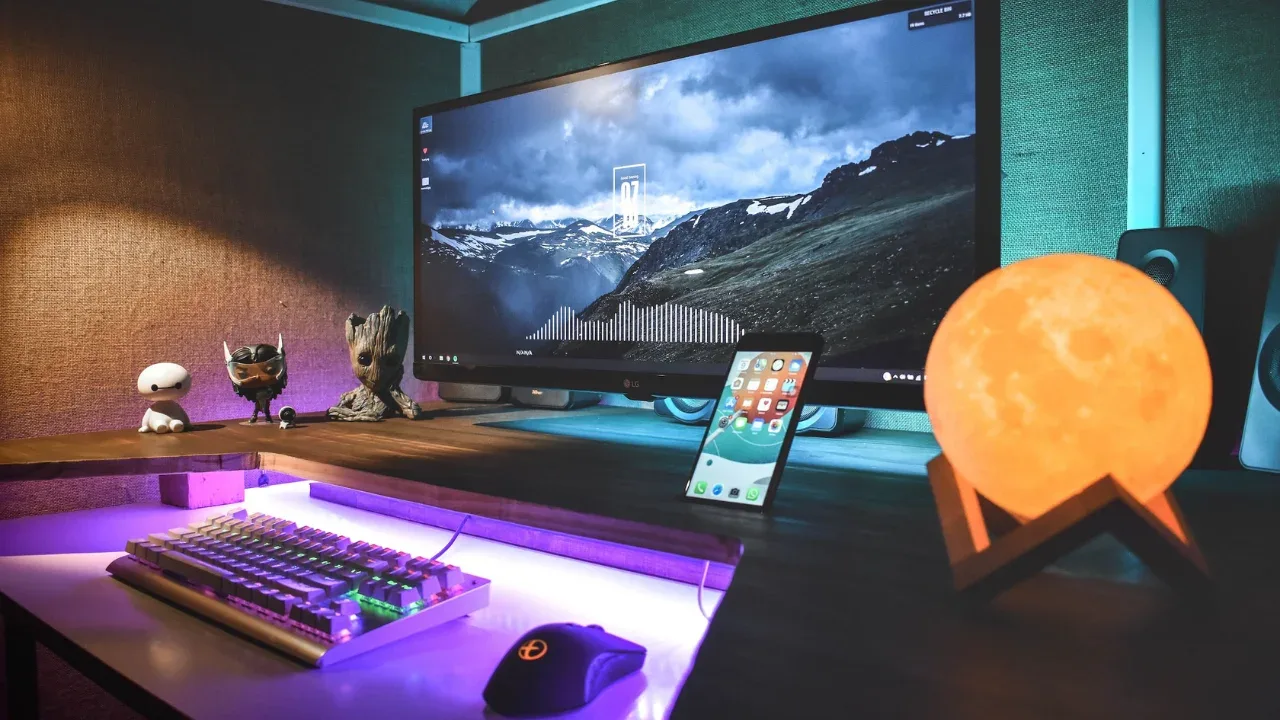
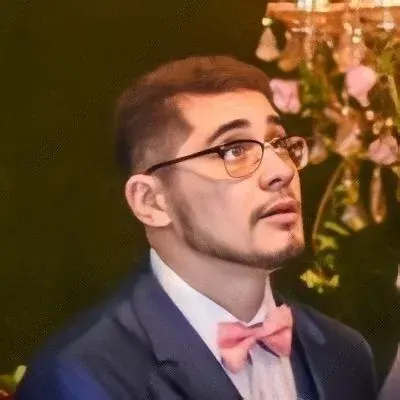
Understanding Python's super() with Multiple Inheritance
Have you ever found yourself confused about how the super()
function works with multiple inheritance in Python? 🤔 Fear not! In this blog post, we will demystify this topic and provide easy solutions to common issues that arise when dealing with super()
and multiple inheritance. Let's dive right in! 🚀
The Case of Third's Initialization
Consider the following code snippet, where we have a class Third
that inherits from both First
and Second
:
class First(object):
def __init__(self):
print("first")
class Second(object):
def __init__(self):
print("second")
class Third(First, Second):
def __init__(self):
super(Third, self).__init__()
print("that's it")
When we create an instance of Third
and initialize it, we might expect the output to be:
first
second
that's it
But how does super().__init__()
determine which parent method to refer to? Can we choose which method runs? Let's find out! 🕵️♂️
Method Resolution Order (MRO)
Before we dive into the workings of super()
with multiple inheritance, we need to understand the concept of Method Resolution Order, often referred to as MRO. The MRO is the order in which Python looks for methods and attributes in a class hierarchy. It determines which method is called when using the super()
function.
Python uses a specific algorithm called the C3 linearization algorithm to compute the MRO. You can read more about it here if you're interested in the details. 😉
In our example, the MRO for class Third
is determined by the order of inheritance: First
followed by Second
. Therefore, the MRO for Third
is Third
, First
, Second
, object
. So when we call super().__init__()
, it will invoke the __init__
method of First
.
Choosing Which Parent Method to Call
What if we want Third
to call the __init__
method of Second
instead of First
? Well, Python allows us to override the MRO by explicitly specifying the parent class we want to call using super()
.
Let's modify our code to enforce calling Second
's __init__
method:
class Third(First, Second):
def __init__(self):
super(Second, self).__init__()
print("that's it")
Now, when we create an instance of Third
, we can expect the following output:
second
that's it
🎉 We've successfully overridden the MRO to call Second
instead of First
! The super()
function is a powerful tool that allows us to control the order of method invocation in multiple inheritance scenarios.
Recap and Call-to-Action
In this blog post, we've explored how Python's super()
function works with multiple inheritance. We've learned about the MRO and how it influences the method resolution process. Additionally, we discovered how to choose which parent method to call using super()
.
Now it's your turn to put this knowledge into practice! 👩💻 Try creating your own classes with multiple inheritance and experiment with super()
to see how it behaves. Feel free to share your experiences and any questions you may have in the comments section below.
Remember, understanding super()
and managing multiple inheritance can be challenging, but with patience and practice, you'll become a master! Happy coding! 🐍💻