How does asyncio actually work?
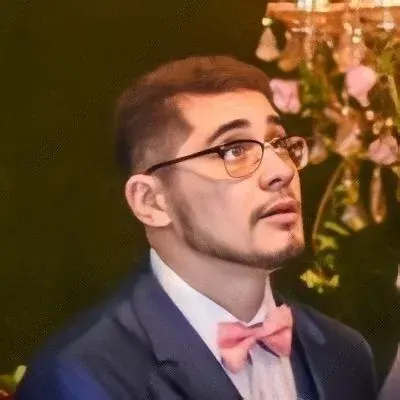
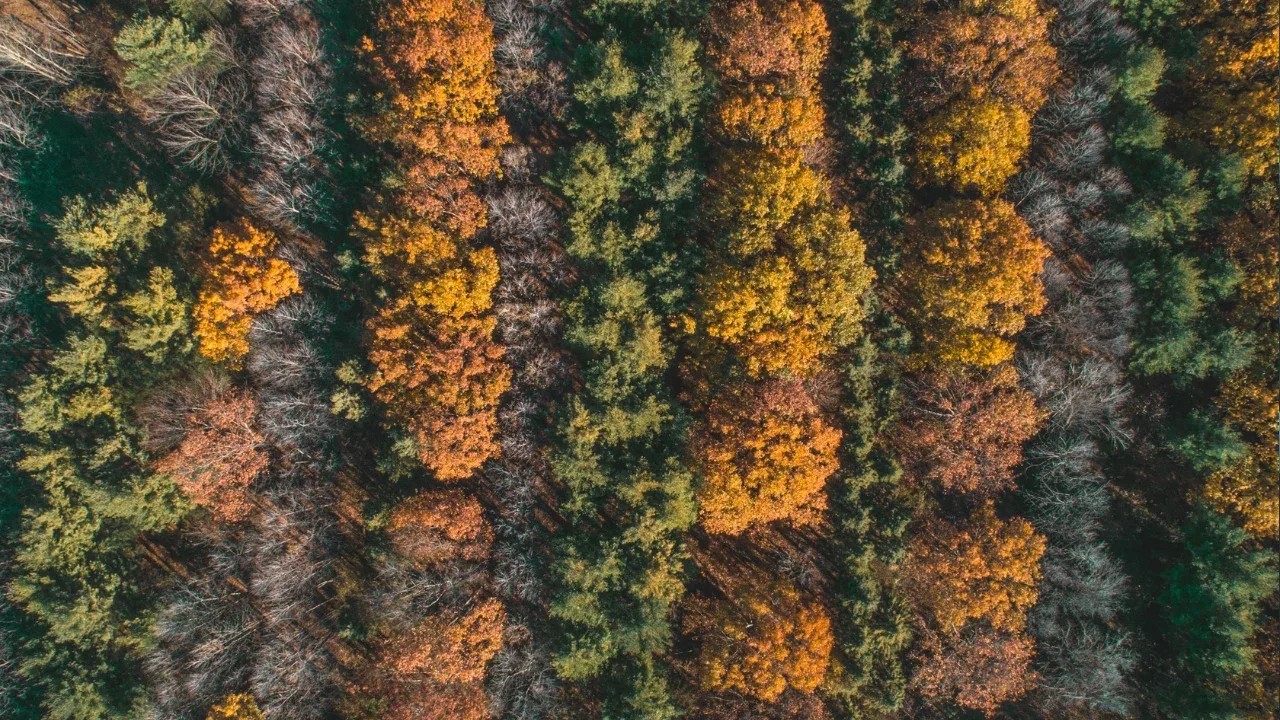
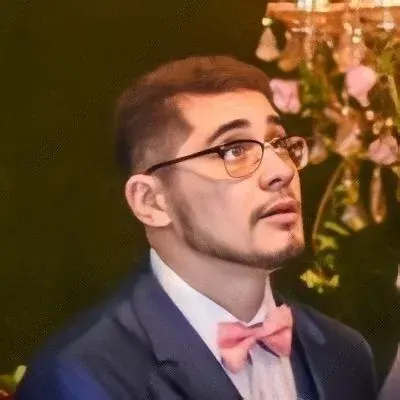
How does asyncio actually work? π‘π§
Have you ever wondered how asyncio works under the hood? π€ It can be a bit confusing, especially when you're faced with thousands of lines of C code and not much helpful documentation to guide you. But fear not, because in this blog post, we'll demystify asyncio and explain how it actually works in a way that is easy to understand. π©βπ»π¨βπ»
The Basics of asyncio π
Asyncio is a powerful framework in Python for writing asynchronous code. It allows you to write concurrent code using coroutines, tasks, and event loops. But how does it all come together to make your I/O operations asynchronous? Let's break it down step by step. πΆββοΈπΆββοΈ
Procedure definitions with the
async def
syntax are actually interpreted as methods of a class inheritingcoroutine
. So, when you define a coroutine function likeasync def foo(): ...
, it becomes a method of acoroutine
class. π―Coroutines are split into multiple methods by
await
statements. This allows the object, on which these methods are called, to keep track of the progress it has made through the execution so far. It's like following a recipe, where each step (await
statement) tells you to wait until something is ready before you can move on to the next step. ππ¦Execution of a coroutine involves calling methods of the coroutine object by a global manager, often referred to as the event loop. The event loop takes care of managing the execution of multiple coroutines, ensuring that they progress smoothly. It's like a conductor leading an orchestra, making sure everyone plays their part at the right time. π»πΊ
The event loop is aware of when I/O operations are performed by Python code and can choose which coroutine method to execute next after the current method relinquishes control. This happens when it encounters an
await
statement, signaling that it needs to wait for a certain task or event to complete before proceeding. It's like juggling multiple tasks, giving each one a turn in an organized manner. πͺβ¨
Understanding the "Desugaring" of asyncio Syntax ππ
As an example, let's take a look at a simple coroutine and how it can be "desugared" to make it more understandable. Consider the following code:
async def coro(name):
print('before', name)
await asyncio.sleep()
print('after', name)
asyncio.gather(coro('first'), coro('second'))
We can translate it into something more relatable like this:
class Coro(coroutine):
def before(self, name):
print('before', name)
def after(self, name):
print('after', name)
def __init__(self, name):
self.name = name
self.parts = self.before, self.after
self.pos = 0
def __call__(self):
self.parts[self.pos](self.name)
self.pos += 1
def done(self):
return self.pos == len(self.parts)
class AsyncIOManager:
def gather(*coros):
while not every(c.done() for c in coros):
coro = random.choice(coros)
coro()
In this simplified version, the Coro
class represents our coroutine, with before
and after
methods corresponding to the steps defined in the original coroutine. The AsyncIOManager
class simulates the event loop, ensuring that all coroutines are executed until they are done.
Handling I/O in asyncio π
But what about I/O operations? How does asyncio handle them? π
In asyncio, I/O operations like reading from a file or making a web request can be handled in multiple ways depending on the underlying implementation. Here are a few possibilities:
Separate Thread: One approach is to run I/O operations in a separate thread or process. This allows the Python interpreter to continue running other code while the I/O operation is being performed outside of the interpreter. The event loop can then handle the synchronization and coordination between the I/O operation and the coroutine execution.
Non-Blocking Sockets: Another technique is to use non-blocking sockets, which allow the Python interpreter to continue running other code while waiting for I/O operations to complete. This is achieved using low-level system calls like
select
orepoll
, which can efficiently monitor multiple I/O sources and notify the event loop when they are ready.Asynchronous Libraries: Some I/O operations can be inherently asynchronous, thanks to specialized libraries that are designed to work seamlessly with asyncio. These libraries provide a high-level interface for performing I/O operations asynchronously, without the need for explicit callbacks or low-level system calls.
Wrapping Up π
And there you have it! A simplified explanation of how asyncio actually works. We've uncovered the magic behind coroutines, event loops, and the coordination of I/O operations in a way that is easy to understand. Now you can dive deeper into the world of asynchronous programming with confidence. π
If you still have questions or want to share your thoughts about asyncio, feel free to leave a comment below. Let's keep the conversation going! π¬β¨
Click here to check out more articles and tutorials on our blog. Don't forget to share this post with your friends who are curious about asyncio! Let's spread the knowledge together. ππ