How do you catch this exception?
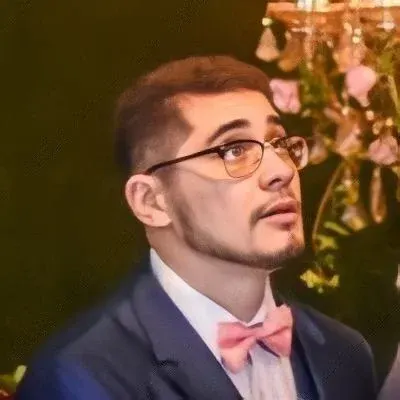

How to Catch That Exception in Django
So you've come across a code snippet that throws an exception in Django, but you're having trouble catching it. Don't worry, you're not alone! In this blog post, we'll explore how to catch that elusive exception and provide easy solutions to common issues. 🚀
The Code Example
Let's take a closer look at the code snippet causing the trouble:
try:
val = getattr(obj, attr_name)
except related.ReverseSingleRelatedObjectDescriptor.RelatedObjectDoesNotExist:
val = None # Does not catch the thrown exception
except Exception as foo:
print type(foo) # Catches here, not above
The problem is that the exception related.ReverseSingleRelatedObjectDescriptor.RelatedObjectDoesNotExist
is not being caught as expected. Instead, it falls into the generic except Exception
block, which may not handle the specific exception properly.
Understanding the Exception Hierarchy
To effectively catch this exception, we need to understand its hierarchy. In Django, the RelatedObjectDoesNotExist
exception is a subclass of both DoesNotExist
and AttributeError
.
Solution 1: Catch the Specific Exception
One solution is to catch the specific exception directly:
try:
val = getattr(obj, attr_name)
except related.ReverseSingleRelatedObjectDescriptor.RelatedObjectDoesNotExist:
val = None # Now catches the thrown exception
except Exception as foo:
print type(foo)
By catching related.ReverseSingleRelatedObjectDescriptor.RelatedObjectDoesNotExist
, we ensure that this specific exception is handled separately from other exceptions. 🎯
Solution 2: Catch the Base Exceptions
If you want to handle all exceptions related to non-existing objects, you can catch the base exceptions DoesNotExist
and AttributeError
:
try:
val = getattr(obj, attr_name)
except (related.DoesNotExist, AttributeError):
val = None # Catches both `DoesNotExist` and `AttributeError`
except Exception as foo:
print type(foo)
By catching these base exceptions, you cover all possible cases where the object doesn't exist or the attribute is missing. This approach provides more flexibility if you need to handle similar exceptions in different scenarios.
Conclusion and Call to Action
Catching exceptions in Django can sometimes be tricky, but now you have the tools to catch that exception with ease! Remember to catch the specific exception or the base exceptions to ensure proper handling. 🛡️
If you found this blog post helpful, make sure to share it with your fellow Django developers and leave a comment below. How do you usually catch exceptions in Django?
Keep coding, keep learning, and catch those exceptions like a pro! 💪✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
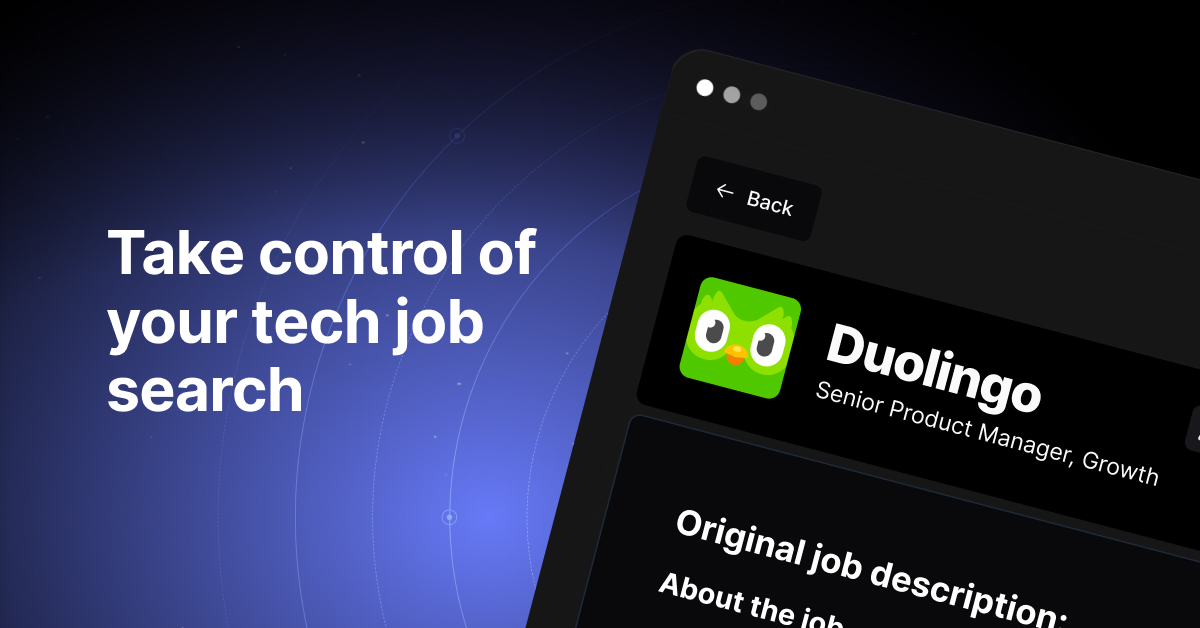