How do I use threading in Python?
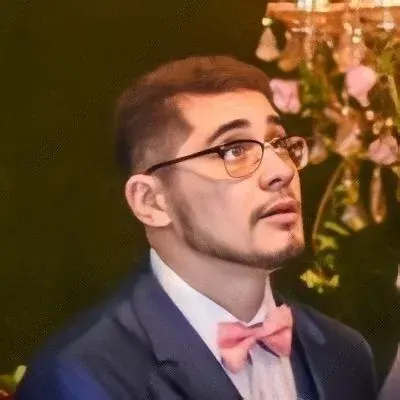
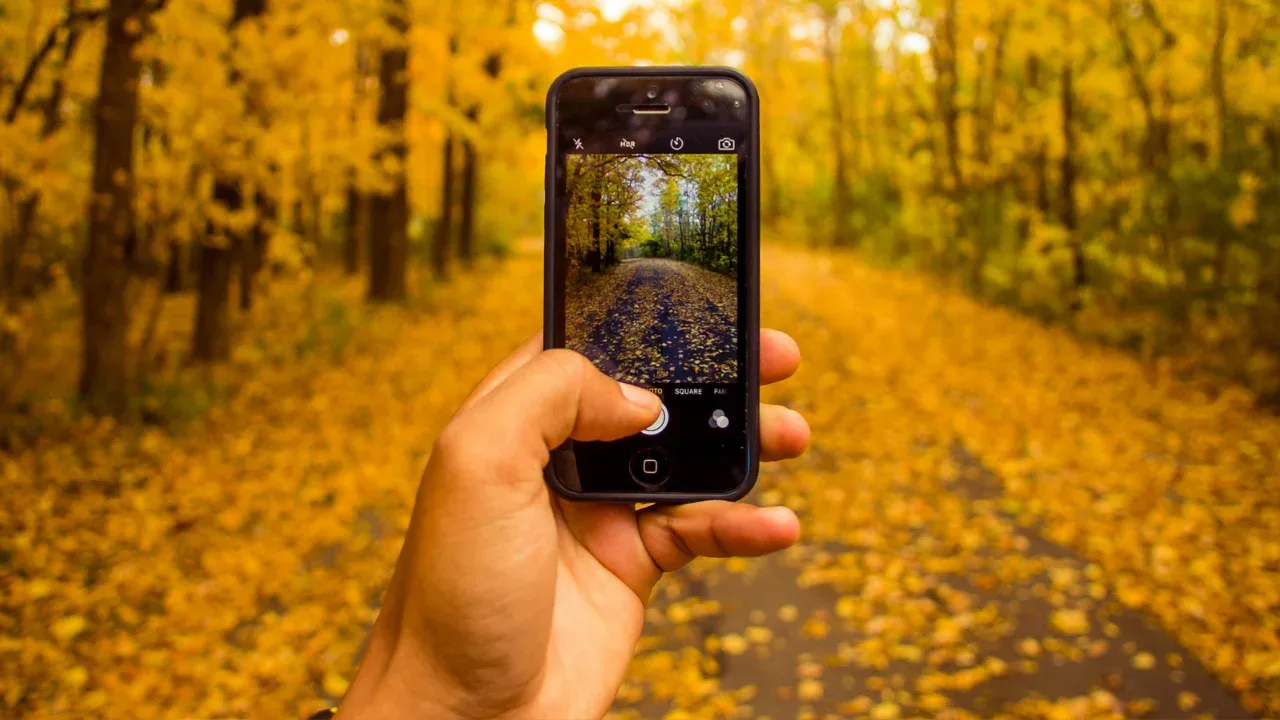
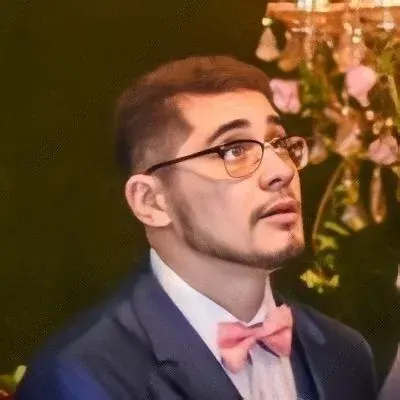
๐งต Threading in Python: Divide and Conquer Tasks Like a Pro! ๐
Are you ready to take your Python game to the next level โฌ๏ธ? Look no further! In this blog post, we will dive into the exciting world of threading ๐งต in Python, where we can divide and conquer tasks like absolute pros! ๐ช
Have you ever felt like your Python code is taking forever to complete because it's executing tasks one after another, causing your program to become sluggish โ? Well, worry no more! With threading, we can split those tasks into multiple threads, allowing them to run concurrently ๐.
Threading 101: Getting Started
Before we delve into the nitty-gritty of threading in Python, let's first understand the basic concepts. Threads are lightweight processes within a program that can execute tasks independently. Unlike traditional sequential execution, threads can run simultaneously, giving a boost to performance when dealing with time-consuming operations.
The Power of Dividing Tasks: An Example
To better visualize the power of threading, let's consider a scenario ๐ : you have a list of 1000 images that need to be resized, and applying the resizing operation sequentially is time-consuming. By implementing threading, we can divide this task among multiple threads, each handling a portion of the images. This approach will undeniably save us a significant amount of time โณ.
Implementing Threading in Python
To start using threading in Python, we need to import the threading
module:
import threading
Next, we can create a new thread by subclassing the Thread
class in Python's threading
module. Let's see an example where we divide the image resizing task among four threads:
import threading
class ResizingThread(threading.Thread):
def __init__(self, images):
threading.Thread.__init__(self)
self.images = images
def run(self):
for image in self.images:
# Perform image resizing operation here
pass
# Create a list of 1000 images
images = ['image1.jpg', 'image2.jpg', ...]
# Divide the images into four equal parts
chunk_size = len(images) // 4
# Create four resizing threads
threads = []
for i in range(4):
thread = ResizingThread(images[i * chunk_size : (i+1) * chunk_size])
thread.start()
threads.append(thread)
# Wait for all threads to complete
for thread in threads:
thread.join()
Notice how we create a new class ResizingThread
, which extends the Thread
class. In the run
method, we loop over the images assigned to that thread and perform the resizing operation. By dividing the images equally among the threads, we ensure each thread gets a fair share of the workload.
A Word of Caution: Thread Safety
When working with threads, it's crucial to be aware of thread safety โ๏ธ. In our example, each thread handles a distinct set of images, so we don't encounter any conflicts. Yet, if multiple threads manipulate shared resources simultaneously, we need to implement synchronization mechanisms like locks or semaphores, ensuring data integrity.
Unlock the Power of Threading ๐
Are you excited to take advantage of threading in your own Python projects? We bet you are! By dividing and conquering your tasks like a pro, you'll witness a significant boost in performance and responsiveness.
So, what are you waiting for? Grab your code editor, import the threading
module, and unleash the full potential of Python threading ๐.
๐ We'd love to hear about your experience with threading in Python! Share your thoughts, interesting use cases, or any questions you have in the comments below. Let's conquer the world of multithreading together! ๐ช๐ฌ