How do I type hint a method with the type of the enclosing class?
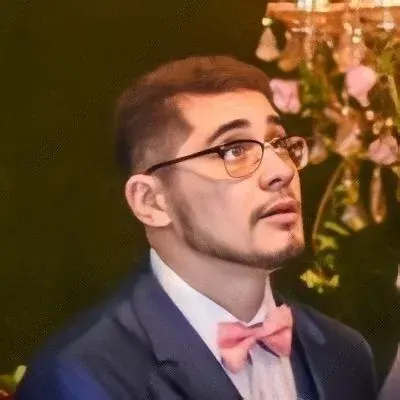
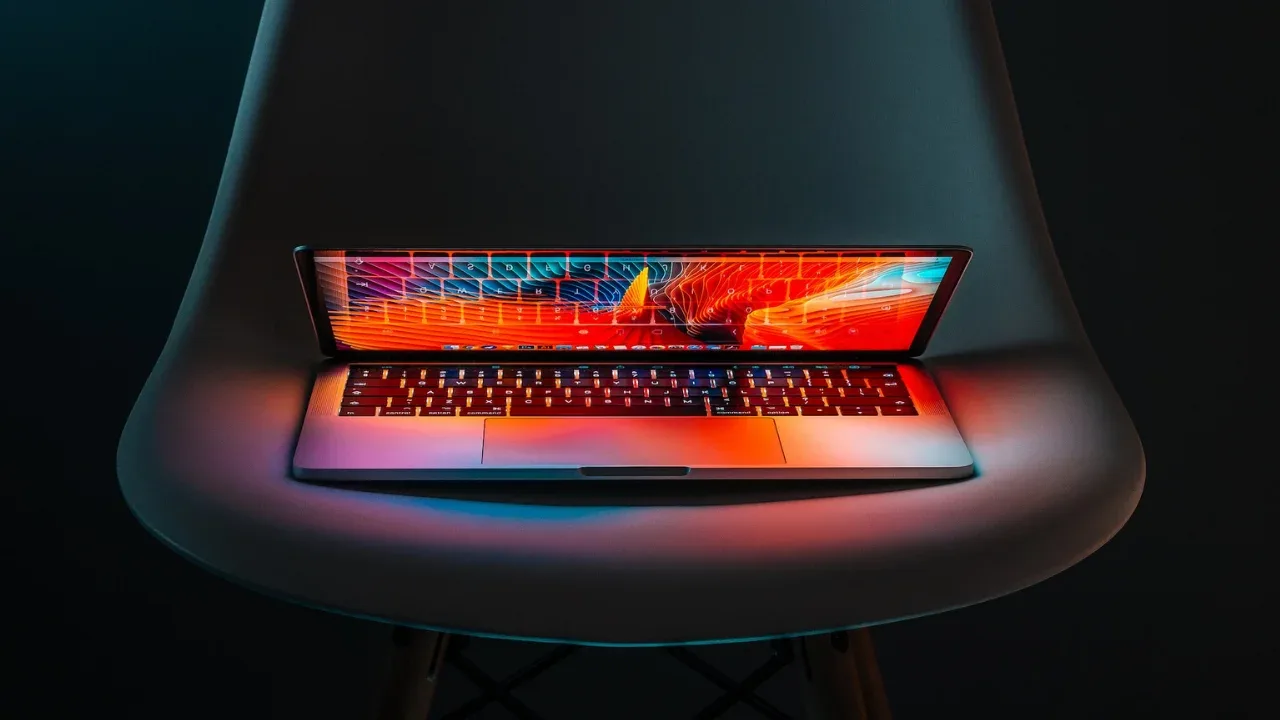
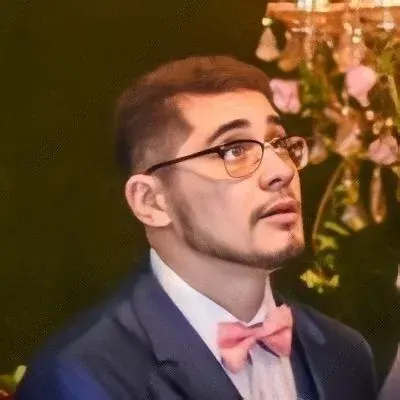
How to Type Hint a Method with the Type of the Enclosing Class 💡
Have you ever encountered an issue where your editor tells you that a reference in your code cannot be resolved? Specifically, have you faced this problem when trying to type hint a method with the type of the enclosing class in Python 3? Fear not, for there is a simple solution to this problem.
Let's take a look at an example to understand the issue better. Suppose we have the following code snippet in Python 3:
class Position:
def __init__(self, x: int, y: int):
self.x = x
self.y = y
def __add__(self, other: Position) -> Position:
return Position(self.x + other.x, self.y + other.y)
In this code, we have a Position
class with a __add__
method. The intention here is to specify that the return type of the method should be of type Position
. However, you may encounter a problem where your editor (in this case, PyCharm) shows a warning that the reference Position
cannot be resolved within the __add__
method.
But fret not, my friend, for this is actually just a PyCharm issue! PyCharm uses the information in its warnings and code completion to provide suggestions and improve your coding experience. In this case, you can rest assured that your code is correct, and you don't need to use any other syntax.
To verify this, you can try running your code and observe that it functions as expected, without any errors or issues. The type hinting works correctly, despite the warning shown by PyCharm.
However, if you still prefer to avoid the warning in your editor, you can make use of a simple workaround. Instead of directly referencing the class name within the type hint, you can use a string representation of the class name. Here's an example:
class Position:
def __init__(self, x: int, y: int):
self.x = x
self.y = y
def __add__(self, other: 'Position') -> 'Position':
return Position(self.x + other.x, self.y + other.y)
By encasing the type hint in quotes, like other: 'Position'
, you are providing PyCharm with enough information to recognize the reference correctly and eliminate the warning. This method is considered a valid solution to overcome the warning in PyCharm, while still maintaining the integrity and accuracy of your code.
To summarize, if you encounter the PyCharm warning stating that a reference cannot be resolved when type hinting a method with the type of the enclosing class, you have two options:
Trust the correctness of your code: Even though PyCharm shows a warning, it does not affect the functionality of your program. Feel free to ignore the warning and proceed with confidence.
Use string representation: If you prefer cleaner code without any warnings, enclose the class name within quotes in the type hint, like
other: 'Position'
. This helps PyCharm recognize the reference correctly.
🚀 Now that you know how to overcome this issue, go ahead and confidently use type hints in your code. Enhance your coding experience and make it easier for others to understand and collaborate with you!
If you found this guide helpful, consider sharing it with other developers who might benefit from it. Let's spread the knowledge and create a thriving community of Python enthusiasts! 🐍💻
Have you faced any other challenges with typing hints in Python or PyCharm? Share your experiences and let's discuss in the comments below!