How do I trim whitespace from a string?
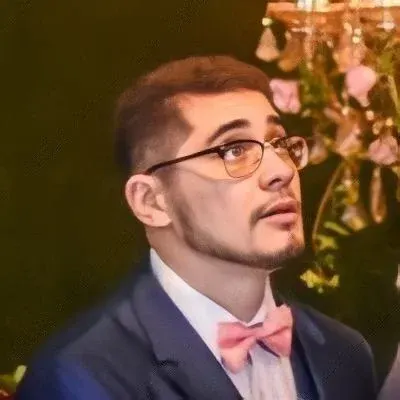
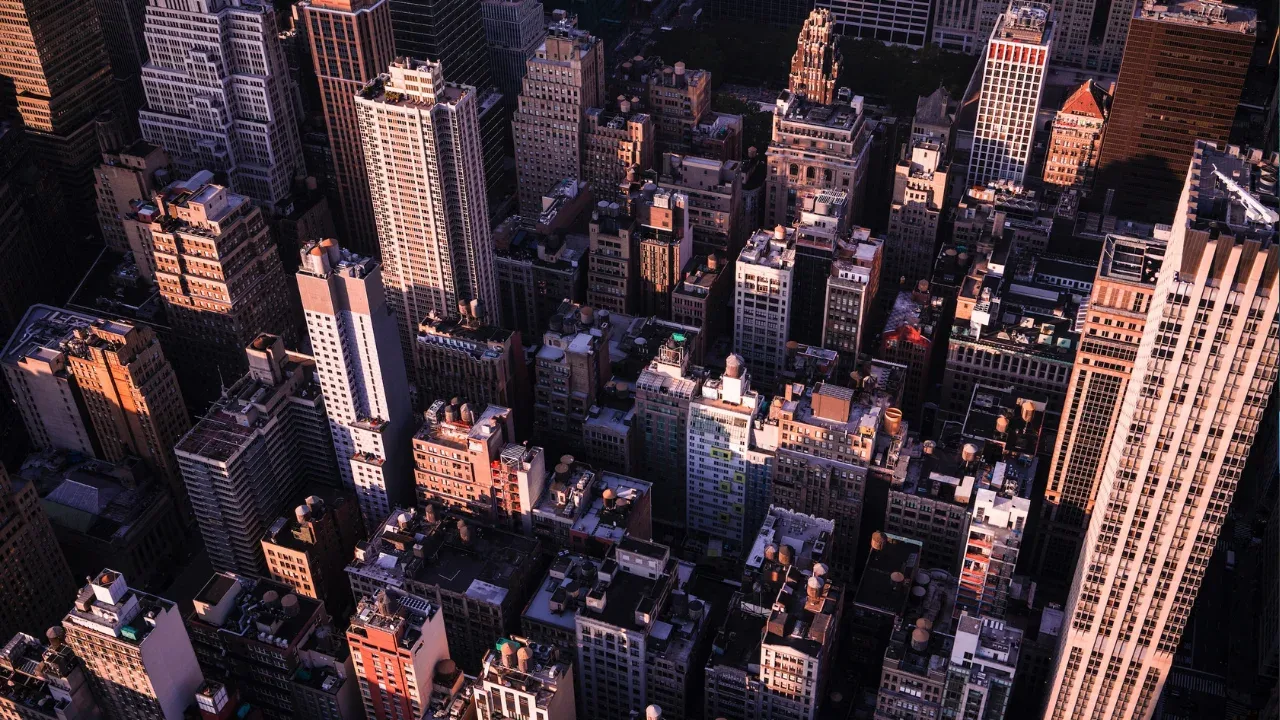
Trim Whitespace from a String: A Guide to Clean Data
š Hey folks, welcome back to my tech blog! Today we're diving into the world of string manipulation and figuring out how to trim those pesky whitespaces in Python. š Whether your data is messy or you just want a tidy output, this guide has got you covered! Let's get started! šŖ
The Problem: Whitespace Woes
Ever encountered a string with unwanted spaces at the beginning or end? Maybe it's causing havoc in your program or messing up your outputs. š« Well, fret no more! We have some super simple solutions to help you deal with this common issue.
The Solution: Strip, Lstrip, and Rstrip
Python offers us three magical methods - strip()
, lstrip()
, and rstrip()
- to trim whitespace from strings. Let's see how each of them works using some examples:
string = " Hello world " # Annoying whitespace all around!
# Using strip() method
trimmed_string = string.strip()
print(trimmed_string) # Output: "Hello world"
# Using lstrip() method
trimmed_string = string.lstrip()
print(trimmed_string) # Output: "Hello world "
# Using rstrip() method
trimmed_string = string.rstrip()
print(trimmed_string) # Output: " Hello world"
Explaining the Methods
The
strip()
method removes whitespace from both ends of the string. It's the Swiss Army knife of whitespace trimming! āļøThe
lstrip()
method specifically removes whitespace from the left (beginning) of the string, leaving the right side untouched. šThe
rstrip()
method, on the other hand, removes whitespace from the right (end) of the string, keeping the left side intact. š
Putting It All Together: An Example
Let's see how we can clean up data using string trimming techniques:
data = [" Apple ", " Banana ", " Cherry "]
cleaned_data = [fruit.strip() for fruit in data]
print(cleaned_data)
# Output: ["Apple", "Banana", "Cherry"]
In this example, we have a list of fruits with whitespace cluttering their names. By applying strip()
to each item in the list using a list comprehension, we get a beautifully cleaned dataset without any extra spaces. ššš
Call-to-Action: Share Your Experiences
Now that you have mastered the art of trimming whitespaces from strings, it's time to put your newfound knowledge to use! Try implementing these techniques in your own projects or share your experiences with us in the comments below. We'd love to hear how you're making your code neater and more efficient! āØš
That's all for this blog post, folks! I hope you found it helpful and enjoyable. Remember, keeping your data clean and well-trimmed is a crucial step towards successful programming. Stay tuned for more exciting tech tips and tricks coming your way! š©āš»š„
Until next time, happy coding! šāļø
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
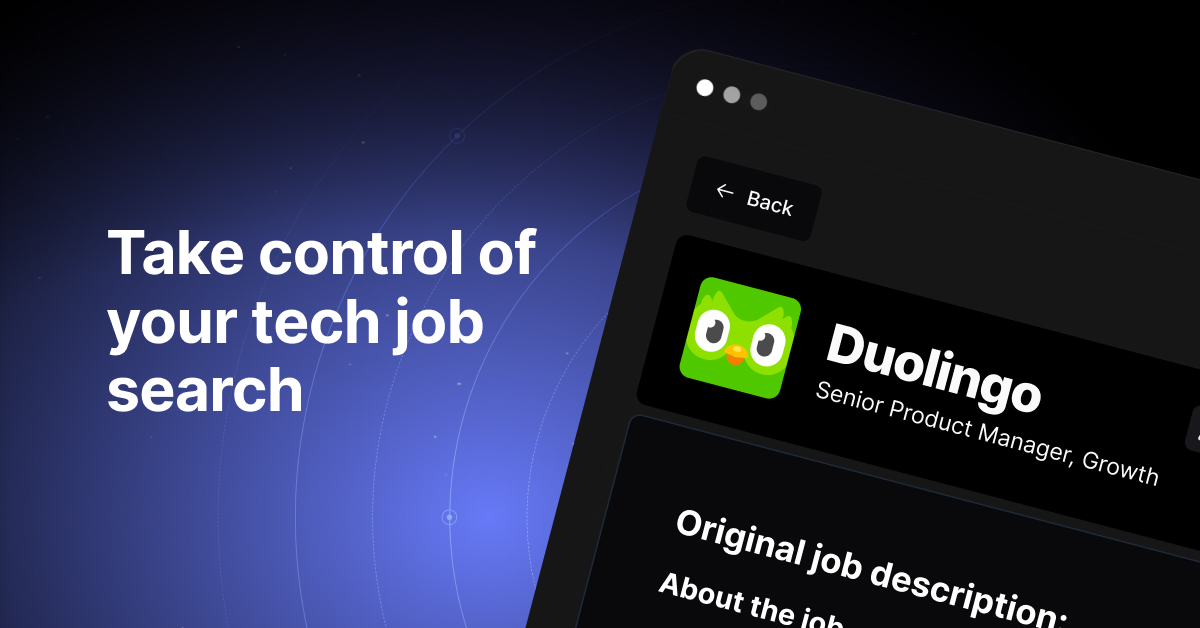