How do I split the definition of a long string over multiple lines?
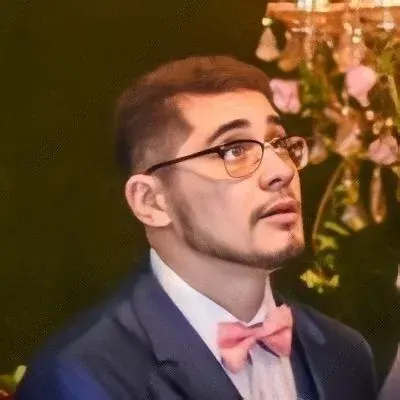
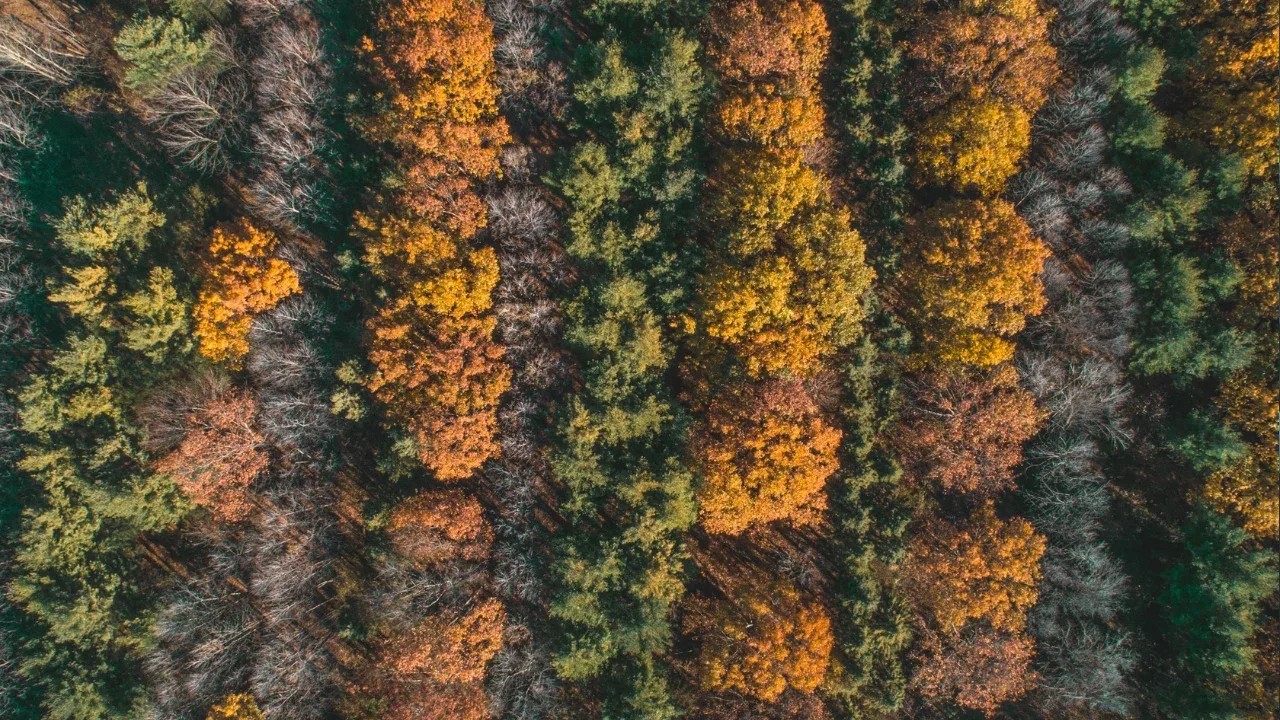
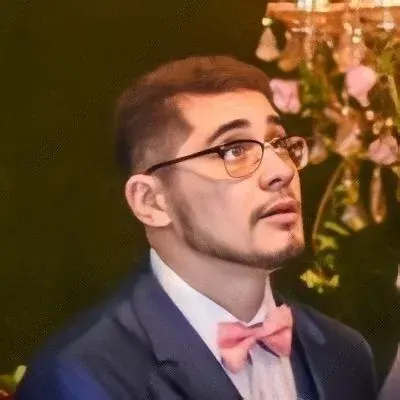
How to Split the Definition of a Long String over Multiple Lines? 📝
So, you have a ridiculously long string that you want to split into multiple lines for better readability and maintainability in your Python code. You've come to the right place! In this blog post, we'll explore the common issues with splitting long strings and provide easy solutions to make your code more readable. Let's get started! 💪
The Dilemma of Long Strings 🤔
Long strings can be a nightmare to work with, especially when you're trying to keep your code clean and readable. It's challenging to maintain the line length limit (which is typically around 80 characters) while ensuring that your code remains easy to follow.
The JavaScript Approach 🌐
In JavaScript, you can split a long string into multiple lines by using the +
operator to join them. While this might not be the most efficient way to handle long strings, it's often used for the sake of readability. Let's take a look at an example:
var long_string = 'some text not important. just garbage to' +
'illustrate my example';
The Pythonic Way 🐍
Unfortunately, Python doesn't support the same approach as JavaScript for splitting strings. But fear not! There's another way to achieve the same result in Python. Let's go through it step by step.
You've mentioned using the \
symbol to split the long string across multiple lines. While this works, it can lead to code that looks a bit awkward and cluttered. Let's take a look at your attempt:
query = 'SELECT action.descr as "action", '\
'role.id as role_id,'\
'role.descr as role'\
'FROM '\
'public.role_action_def,'\
'public.role,'\
'public.record_def, '\
'public.action'\
'WHERE role.id = role_action_def.role_id AND'\
'record_def.id = role_action_def.def_id AND'\
'action.id = role_action_def.action_id AND'\
'role_action_def.account_id = ' + account_id + ' AND'\
'record_def.account_id=' + account_id + ' AND'\
'def_id=' + def_id
As you can see, your attempt is missing some essential line breaks, resulting in a concatenated mess. Let's improve it together using a more readable and Pythonic approach.
The Pythonic Solution 🐍✨
To split a long string in Python while maintaining code readability, you can use parentheses to group the multiple lines together. Let's rewrite your code with this approach:
query = (
'SELECT action.descr as "action", '
'role.id as role_id, '
'role.descr as role '
'FROM '
'public.role_action_def, '
'public.role, '
'public.record_def, '
'public.action '
'WHERE role.id = role_action_def.role_id AND '
'record_def.id = role_action_def.def_id AND '
'action.id = role_action_def.action_id AND '
'role_action_def.account_id = ' + account_id + ' AND '
'record_def.account_id=' + account_id + ' AND '
'def_id=' + def_id
)
Now, that looks way more readable and organized! By enclosing the entire string in parentheses, we can split it into multiple lines without the need for the \
symbol at the end of each line.
Your Turn! 🚀
Congratulations! You now know how to split the definition of a long string over multiple lines in Python. Give it a try in your own code and experience the joy of cleaner and more readable code. If you have any other questions or need further assistance, feel free to leave a comment below. We're here to help! 😊
Let's make our code more readable together! 💪🔥