How do I split a string into a list of words?
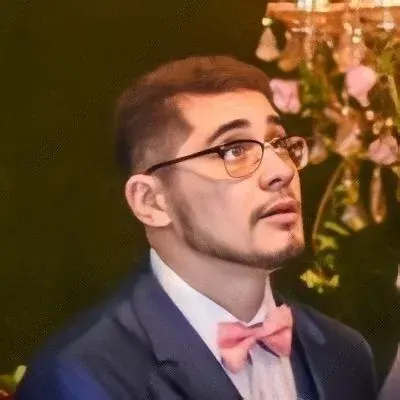
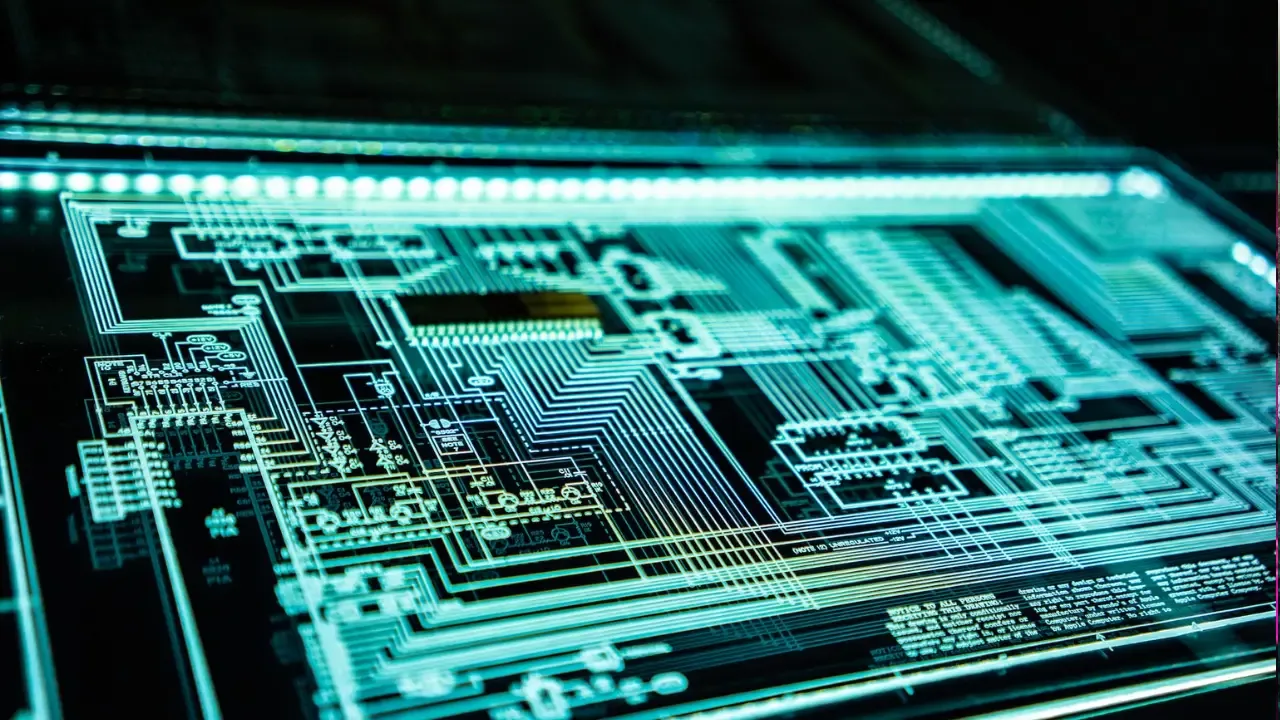
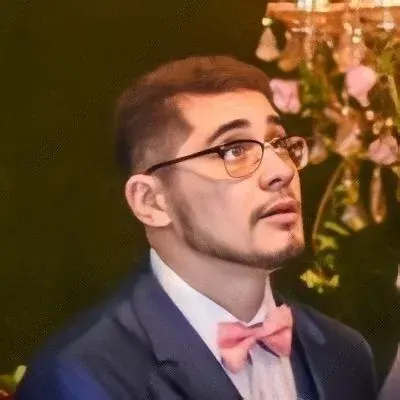
How to Split a String into a List of Words
So you want to split a sentence into individual words and store them in a list? No worries! In this guide, we'll walk you through the process step by step. πΆββοΈπΆββοΈ
The Problem
Let's say you have a string like this: "these are words"
. You want to split this sentence and store each word in a list, resulting in ["these", "are", "words"]
. How can you achieve this? π€
The Solution
Python provides a powerful built-in function called split()
that makes our lives easier in this situation. The split()
function splits a string into a list of substrings based on a specified delimiter. In our case, the delimiter is a space character. π―
Here's how you can split a string into a list of words:
sentence = "these are words"
words = sentence.split()
That's it! The split()
function does all the magic for you. It takes the input string sentence
, splits it into individual words based on space characters, and stores the words in the words
list. πͺ
Advanced Usage
The split()
function is quite flexible. It allows you to split a string based on a specific delimiter other than the default space character. For example, if you have a string like "apple,banana,orange"
, and you want to split it based on commas, you can do:
fruits = "apple,banana,orange"
fruit_list = fruits.split(",")
This will give you ["apple", "banana", "orange"]
. Amazing, right? πππ
Common Issues
Handling Extra Spaces
Sometimes, your sentence might contain extra spaces between words. These extra spaces can lead to empty strings in your resulting list. To avoid this, you can call the split()
function with the split(" ")
argument. This will ensure that any consecutive spaces are treated as a single delimiter. π§Ή
For example, let's consider the string "these are words"
. If you split it using split(" ")
, you'll get ["these", "are", "words"]
, eliminating the extra spaces.
Different Types of Whitespace
The split()
function uses space characters as the default delimiter. However, keep in mind that space characters include not only the regular space character but also tabs, newline characters, and other whitespace characters. This means that the function can handle strings with various types of whitespace without any extra effort. π
Conclusion
Splitting a string into a list of words is a common task, and with the split()
function in Python, it's super easy. Just remember to provide the necessary input string and let Python take care of the rest. π
Now that you know how to split strings into lists of words, go ahead and give it a try! Experiment with different sentences and explore the power of the split()
function. Happy coding! π»π