How do I split a list into equally-sized chunks?
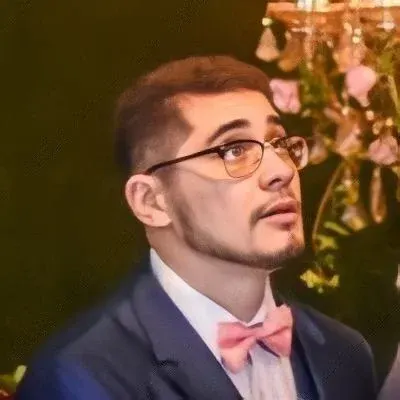
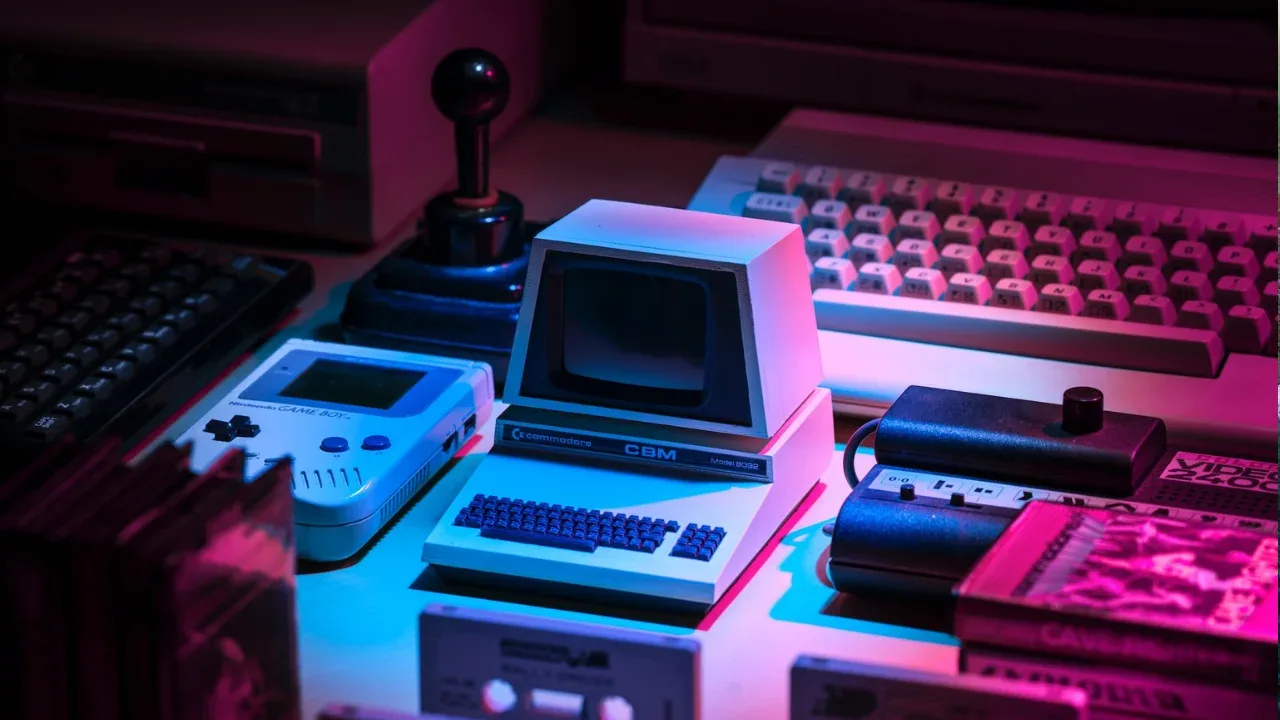
Splitting a List into Equally-Sized Chunks: A Complete Guide
Are you tired of trying to figure out how to split a list into equally-sized chunks? You're not alone! Many developers struggle with this common problem. Whether you're working with a large list of data or just want to organize your information into manageable pieces, splitting a list can be a tricky task.
In this blog post, we'll explore some common issues and provide easy solutions to help you divide your list into equal-sized chunks. By the end of this guide, you'll have a clear understanding of how to tackle this problem and improve your coding skills. Let's dive in! 💻🔥
The Problem: Splitting a List into Equal-Sized Chunks
Imagine you have a list of arbitrary length, and you want to split it into chunks of the same size. For example, let's say you have a list of 10 elements, and you want to split it into chunks of 3 elements each. How do you go about accomplishing this task programmatically?
The Solution: Python to the Rescue! 🐍
Python provides an elegant solution to this problem using list comprehensions and the range()
function. Here's a simple code snippet that splits a list into equal-sized chunks:
def chunk_list(lst, size):
return [lst[i:i+size] for i in range(0, len(lst), size)]
Let's break down this solution step by step:
We define a function called
chunk_list
that takes two parameters:lst
(the list to be chunked) andsize
(the desired chunk size).Using list comprehension, we iterate over the range of indices. The start index is 0, and we increment it by
size
on each iteration.For each iteration, we extract a portion of the list starting from the current index (
lst[i:i+size]
), which gives us a chunk of the desired size.The resulting chunks are collected in a new list and returned as the output.
That's it! With just a few lines of code, you can split a list into equally-sized chunks.
Example and Test
To put this solution into perspective, let's go back to our initial example of splitting a list of 10 elements into chunks of 3 elements each:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
chunked_list = chunk_list(my_list, 3)
print(chunked_list)
The output will be: [[1, 2, 3], [4, 5, 6], [7, 8, 9], [10]]
.
As you can see, the list has been successfully split into equal-sized chunks, with the last chunk containing the remaining elements.
Feel free to test this solution with different list sizes and chunk sizes to ensure it handles various scenarios correctly.
Wrapping Up and Taking Action! ✨
Congratulations! You now have the knowledge and tools to split a list into equally-sized chunks using Python. Make sure to bookmark this guide for future reference so you can easily tackle this problem whenever it arises.
But don't stop here! Exploring other programming languages and their approaches to this problem can further improve your coding skills. Additionally, you can adapt this solution to suit your specific needs, such as chunking strings or implementing more complex logic.
Now it's your turn! Put your newfound knowledge into practice and share your experience with us in the comments below. How do you split lists in other programming languages? We'd love to hear your insights and learn from your expertise. Happy coding! 💪🚀
<hr /> <p><sub>See also: <a href="https://stackoverflow.com/q/434287">How to iterate over a list in chunks</a>.<br /> To chunk strings, see <a href="https://stackoverflow.com/questions/9475241">Split string every nth character?</a>.</sub></p>
Did you find this guide helpful? Share it with your fellow developers and spread the knowledge! 🌟📚
In this blog post, we addressed the common issue of splitting a list into equally-sized chunks. We provided an easy and elegant solution using Python, explained it step by step, and even provided an example to illustrate how it works. Make sure to bookmark this guide, try it out in your own projects, and share your insights with us in the comments below! 💡💬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
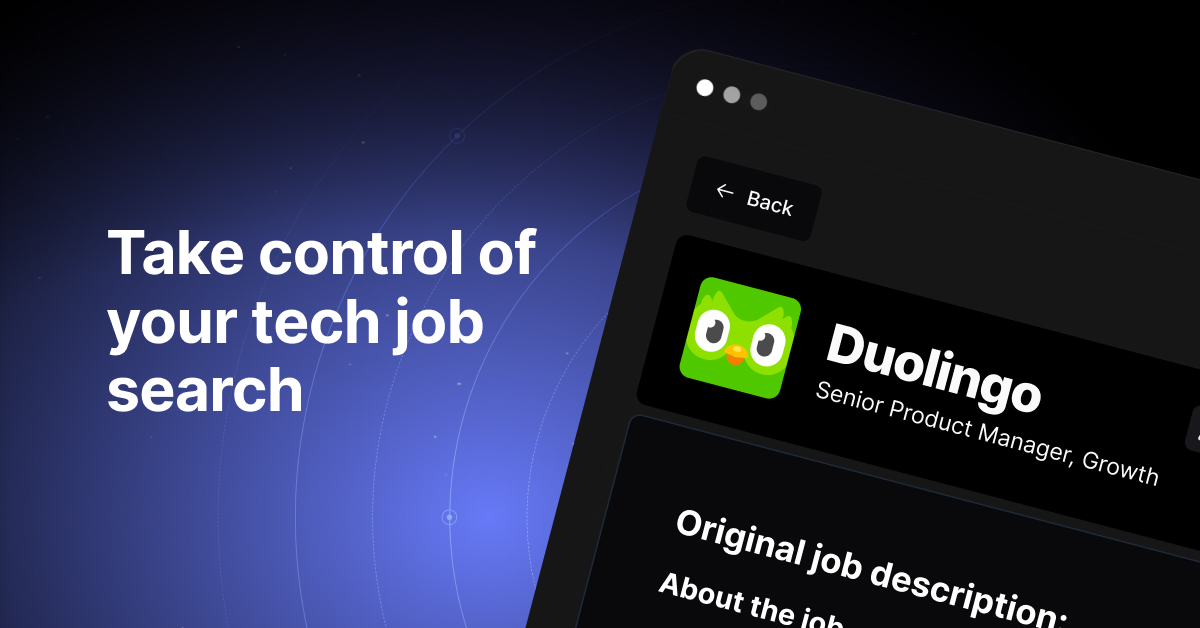