How do I sort a list of objects based on an attribute of the objects?
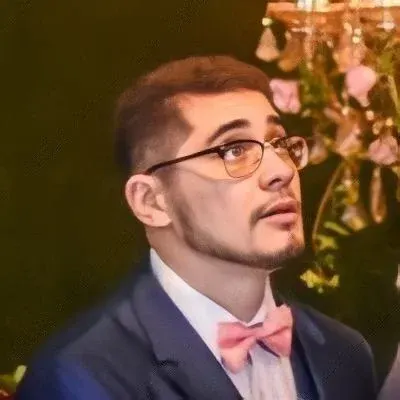

Sorting a List of Objects based on an Attribute: A Quick Guide
Are you struggling to sort a list of objects based on a specific attribute? Look no further! In this guide, we'll walk you through the process step by step, so you can sort your objects and conquer your coding challenges. Let's dive in and start sorting! 🚀
Understanding the Problem
The main challenge here is sorting a collection of Python objects by a particular attribute of each object. In this case, we have a list of objects with different attributes, including name
and count
. Our goal is to sort the list based on the count
attribute in descending order.
The Solution
To solve this problem, we'll utilize Python's built-in sorted()
function with a custom key. The key
parameter allows us to specify a function that will be used to extract a comparison key from each object in the list before sorting.
Here's the code snippet that accomplishes the task:
sorted_list = sorted(your_list, key=lambda obj: obj.count, reverse=True)
Let's break down the above code:
sorted()
is a powerful built-in function in Python that returns a new list containing all items from the original list, sorted in the specified order.The
key
parameter takes a lambda function, which extracts thecount
attribute from each object (obj.count
) for comparison during the sorting process.Setting
reverse=True
ensures the sorting is done in descending order.
Applying the Solution to Our Example
Using the provided context, let's apply the solution to our example. We want to sort a list of Tag
objects based on the count
attribute:
tags = [Tag(name="toe", count=10), Tag(name="leg", count=2), ...]
sorted_tags = sorted(tags, key=lambda tag: tag.count, reverse=True)
The resulting sorted_tags
list will have the objects sorted in descending order based on the count
attribute:
[Tag(name="toe", count=10), Tag(name="leg", count=2), ...]
💡 Pro Tips:
If you have a large list or need to sort the list multiple times, consider using the
list.sort()
method instead ofsorted()
. Thelist.sort()
method modifies the original list in place, resulting in better performance.If you're sorting based on a string attribute, you can use the
key
parameter along with thestr.lower
method to perform a case-insensitive sort, ensuring consistent results.Experiment with different attributes and sorting orders to customize the sorting results according to your specific requirements.
Conclusion
Sorting a list of objects based on a specific attribute is made easy with Python's sorted()
function and the key
parameter. By following the steps outlined in this guide, you can conquer this common coding challenge and sort your objects effortlessly.
Now that you have mastered the art of sorting objects, go ahead and give it a try in your own code. Feel free to share your experiences or any additional tips in the comments section below. Happy coding! 😄👩💻👨💻
Let's sort it like a pro!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
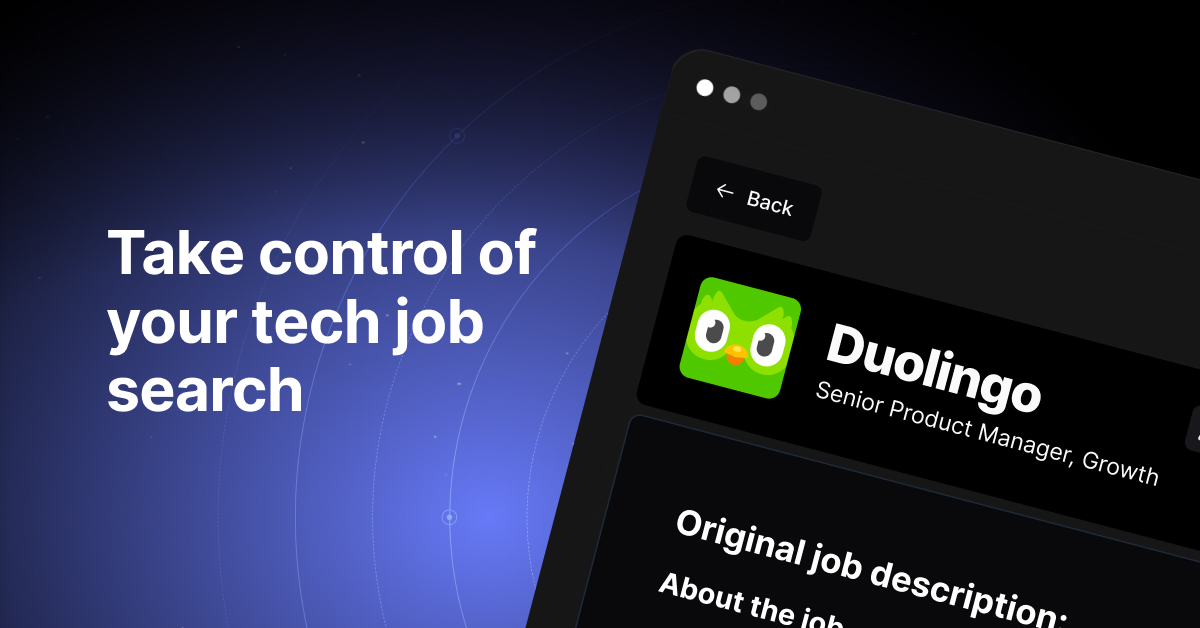