How do I sort a dictionary by key?
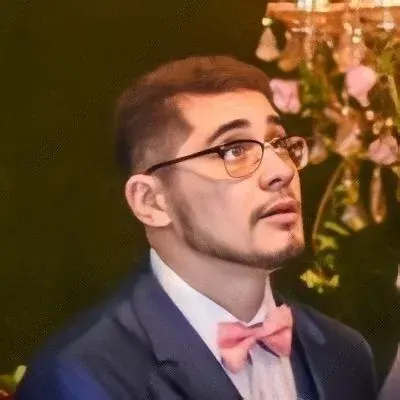
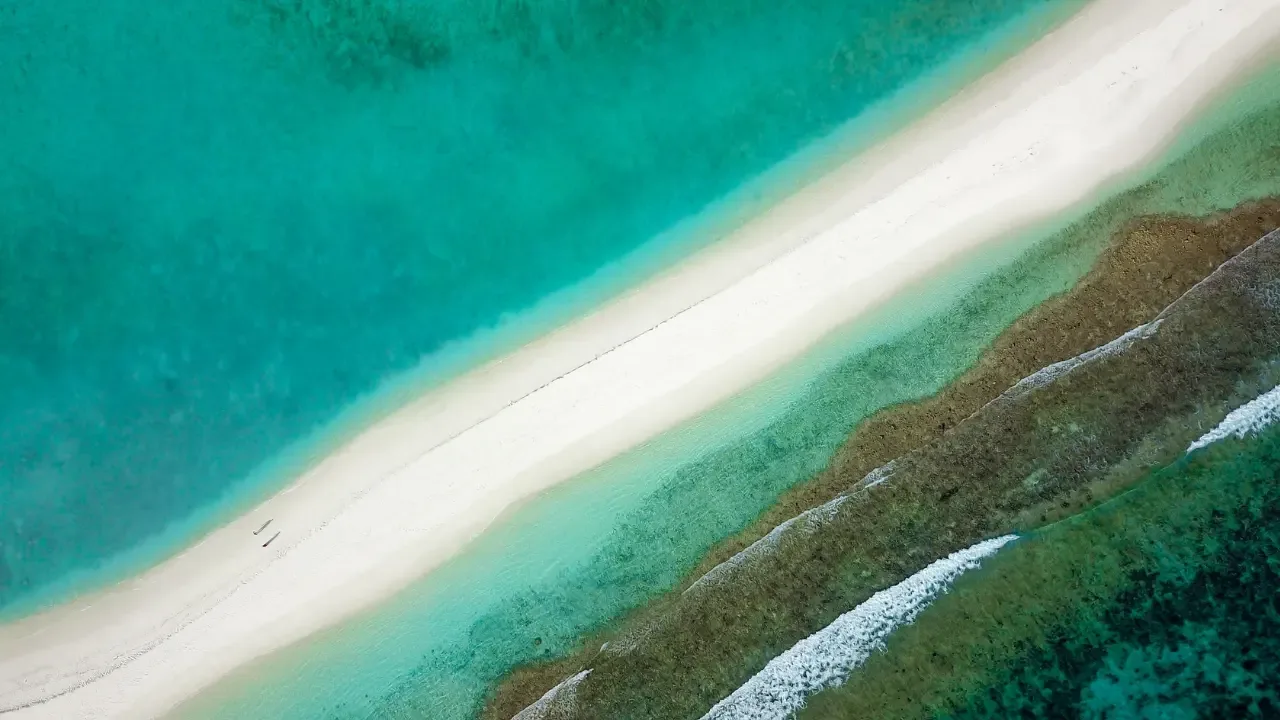
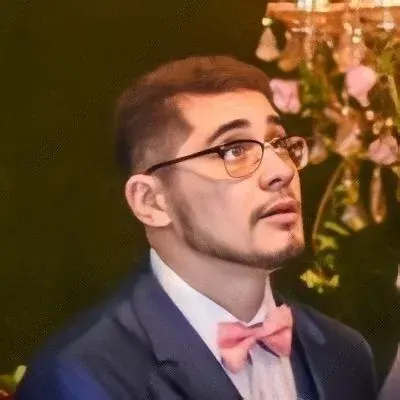
How to Sort a Dictionary by Key: A Simple Guide ๐
So you've stumbled upon a dictionary in your code and realized that it's not sorted the way you want it to be. Fear not, because I'm here to help you sort it out! ๐
The Problem ๐ค
By default, dictionaries in Python don't have a specific order. This means that when you print or iterate through a dictionary, the order of the keys may not be what you expect. But if you want to sort the dictionary by its keys, there's a simple solution for you! ๐ก
The Solution ๐ก
To sort a dictionary by its keys, you can make use of the built-in sorted()
function along with a lambda expression. Here's how you can do it:
my_dict = {2: 3, 1: 89, 4: 5, 3: 0}
sorted_dict = dict(sorted(my_dict.items(), key=lambda x: x[0]))
print(sorted_dict)
In the example above, we use the sorted()
function to sort the items of the dictionary my_dict
. The key=lambda x: x[0]
parameter specifies that we want to sort the dictionary based on its keys. Finally, we convert the sorted items back into a dictionary using the dict()
function.
The Output โ๏ธ
After sorting the dictionary, the output should be:
{1: 89, 2: 3, 3: 0, 4: 5}
Voila! Your dictionary is now properly sorted by its keys. ๐
Take It Further ๐
If you want to sort the dictionary in reverse order (descending), you can modify the key
parameter like this:
sorted_dict = dict(sorted(my_dict.items(), key=lambda x: x[0], reverse=True))
Feel free to experiment and play around with different options to suit your needs. ๐งช
Share Your Experiences! ๐ข
Sorting a dictionary by its keys is a common problem, and I hope this simple guide has provided you with a quick and easy solution. Now it's time to put it into practice! Share your experience in the comments below and let me know if there's anything else you'd like to learn.
Keep coding and keep exploring! ๐๐ป