How do I select rows from a DataFrame based on column values?
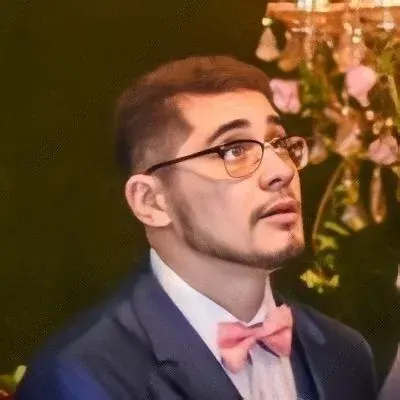
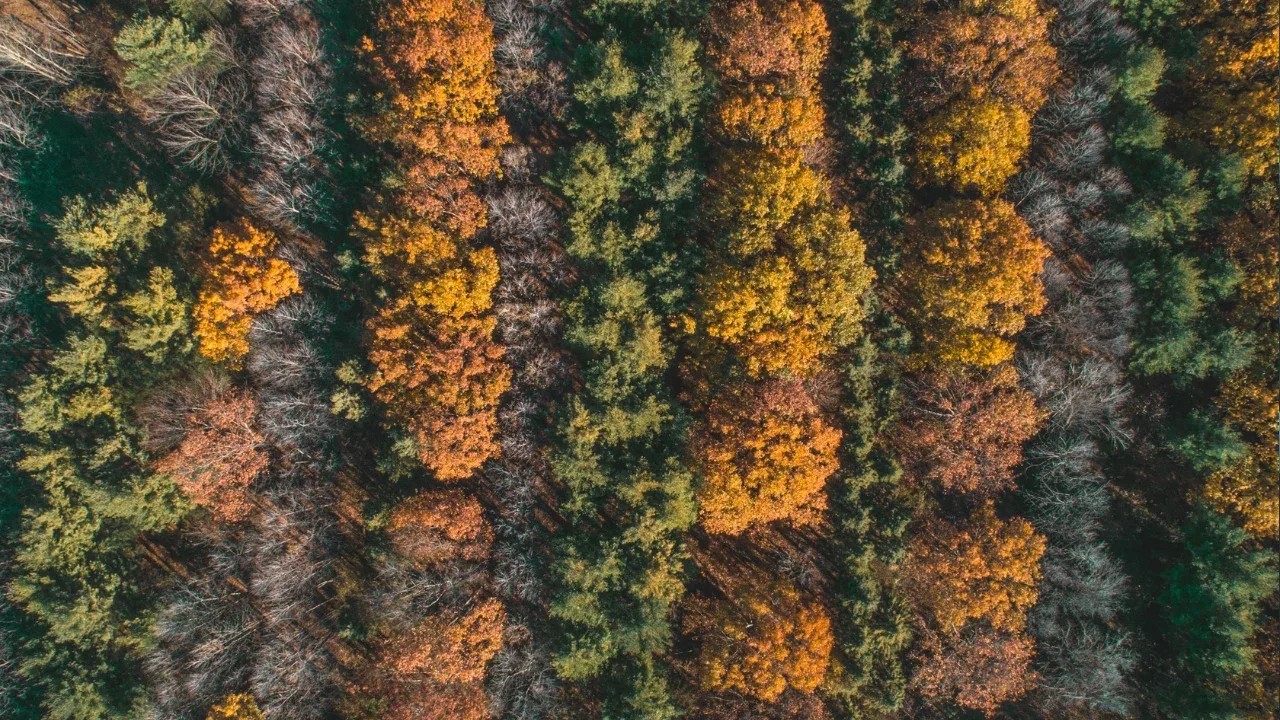
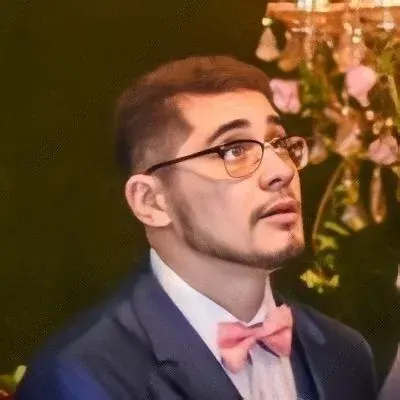
๐ DataFrame Magic: Selecting Rows Based on Column Values ๐งโโ๏ธ
Are you a data wizard with a knack for DataFrame manipulation? If so, you've probably come across the common conundrum of selecting rows from a DataFrame based on specific column values. Fear not, my fellow data sorcerer! In this enchanting guide, I'll show you how to cast a spell on your DataFrame and summon only the rows that meet your criteria. โจ
๐ค The Challenge
Imagine you have a DataFrame filled with magical data, and you want to extract only the rows that match certain values in a specific column. You may have tried to solve this puzzle by turning to your trusty SQL skills, but unfortunately, traditional SQL syntax won't work here. So how can you conquer this challenge?
๐ก The Solution
With the Pandas library, you hold the power to filter rows based on column values quickly and effortlessly. To perform this sorcery, you only need a few lines of code. Let's dive in and reveal the magical incantations you'll need.
Step 1: Import the Pandas Library
Before you embark on your DataFrame manipulation quest, make sure to import the Pandas library:
import pandas as pd
Step 2: Create Your DataFrame
Next, create a DataFrame using your magical data. You can use various sources like CSV files, SQL databases, or simply create one from scratch.
data = {'Name': ['Harry', 'Hermione', 'Ron', 'Draco', 'Luna'],
'House': ['Gryffindor', 'Gryffindor', 'Gryffindor', 'Slytherin', 'Ravenclaw'],
'Year': [7, 7, 6, 5, 5]}
df = pd.DataFrame(data)
Your DataFrame now contains enchanted data with columns named 'Name', 'House', and 'Year'.
Step 3: Select Rows Based on Column Values
The moment of truth has arrived! Use the following Pandas syntax to elegantly select rows based on column values:
selected_rows = df[df['House'] == 'Gryffindor']
In this example, we selected all the rows from the DataFrame where the 'House' column has the value 'Gryffindor'. Feel free to adjust the comparison operator and value to meet your specific requirements. The result will be a new DataFrame containing only the rows that match your criteria.
Step 4: Behold the Magic! ๐ช
Marvel at your mystical powers as you display the selected rows:
print(selected_rows)
Now, when you execute this spell, you will witness only the rows that are affiliated with the noble house of Gryffindor.
๐ฆ Advanced Uses
Now that you possess the baseline knowledge of selecting rows based on column values, let's explore some additional sorcery that will make you an expert in DataFrame manipulation.
Multiple Conditions
Suppose you want to filter your DataFrame by multiple conditions. Simply combine the conditions using logical operators like &
(and) or |
(or).
selected_rows = df[(df['House'] == 'Gryffindor') & (df['Year'] == 7)]
In this example, we selected only the rows where the 'House' column has the value 'Gryffindor' and the 'Year' column has the value 7.
Negation
To choose rows that do not match certain criteria, you can use the negation operator ~
.
selected_rows = df[~(df['House'] == 'Gryffindor')]
This example will select all the rows except those affiliated with the Gryffindor house.
๐ฃ Share Your Wisdom
Congratulations, budding DataFrame magician! You now possess the knowledge to select rows from a DataFrame based on column values. Use this newfound power wisely and remember to share your wisdom with the enchanted community. ๐ซ
Let's start a conversation! Share your favorite DataFrame manipulation spells or ask questions about other enigmatic challenges you're facing. Join the discussion below and embark on a magical journey together! ๐งโโ๏ธ๐งน
โจ Stay enchanted and happy coding! โจ