How do I reverse a string in Python?
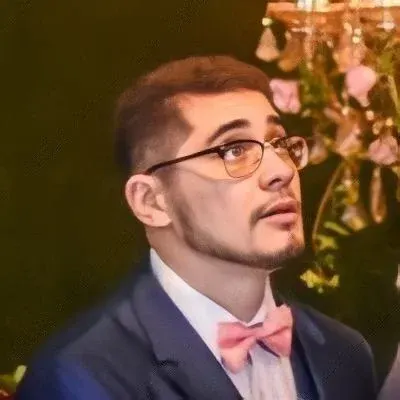
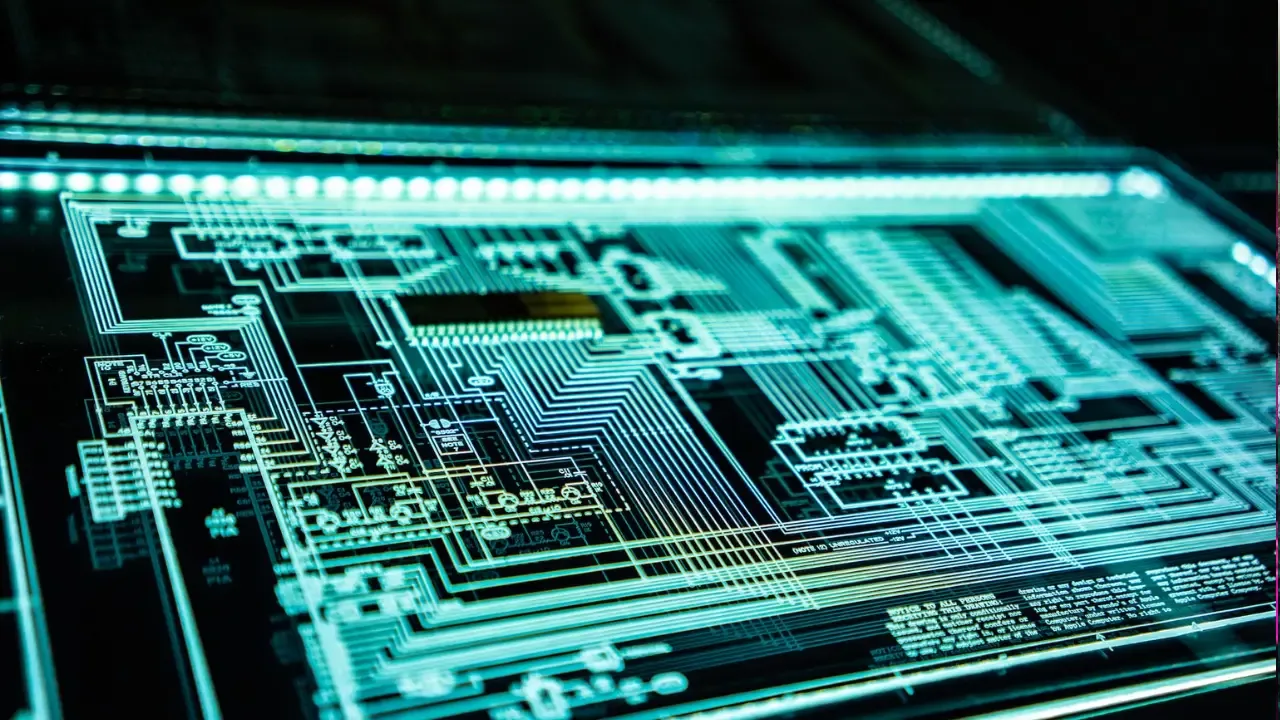
How to Reverse a String in Python: A Beginner's Guide 👨💻
Have you ever found yourself in a situation where you needed to reverse a string in Python? Maybe you want to transform "hello" into "olleh" or simply unravel a pesky palindrome puzzle 🧩. Fear not, for we have you covered! In this blog post, we will walk you through various ways to reverse a string in Python, addressing common issues, providing easy solutions, and guaranteeing your path to string reversal success. So let's dive in! 🏊♂️
The Challenge
So, you discovered that Python does not have a built-in reverse
function for its str
object. Bummer! But don't let this setback discourage you. There are several ways to tackle this problem that do not require writing a complex algorithm. Let's explore a few of them, shall we? 🤓
Solution 1: Using Slicing 🍰
One of the most straightforward and pythonic ways to reverse a string is by using slicing. Slicing allows you to access specific parts of a sequence, such as a string, list, or tuple. To reverse a string using slicing, follow this simple code snippet:
string = "hello"
reversed_string = string[::-1]
print(reversed_string) # Output: olleh
Here, [::-1]
is the slice that reverses the string. By omitting the start and end indices, we inform Python that we want to include all characters in the string in reverse order. Voilà! 🪄 You have your reversed string!
Solution 2: Using the join
and reversed
functions 🍥
If you prefer a more concise approach, you can use the join
and reversed
functions in combination. Here's a simple code snippet that demonstrates this technique:
string = "hello"
reversed_string = ''.join(reversed(string))
print(reversed_string) # Output: olleh
In this example, the reversed
function returns an iterator that goes through the characters of the string in reverse order. We then use the join
function to concatenate these characters back into a single string. As a result, we obtain the reversed string we were yearning for. 🎉
Solution 3: Using a loop ✨
Suppose you want to reverse a string manually, without relying on any fancy built-in functions. No problemo! Python's loop capabilities come to the rescue. Here's a code snippet demonstrating how to achieve this:
string = "hello"
reversed_string = ""
for char in string:
reversed_string = char + reversed_string
print(reversed_string) # Output: olleh
In this example, we initialize an empty string reversed_string
and iterate over each character char
in the original string. By concatenating each character to the beginning of reversed_string
, we build the reversed string step by step. And just like that, you've got yourself a reversed string! 💪
Efficiency Considerations 🚀
When it comes to efficiency, the slicing method (Solution 1) is the winner. Slicing allows Python to access the string in reverse order without explicitly creating a new object. On the other hand, Solution 2 and Solution 3 both involve extra memory allocation to store intermediate objects, which may impact performance for large strings. Therefore, if efficiency is crucial for your use case, slicing should be your go-to solution.
Your Turn! 📢
Now that you've learned a few different ways to reverse a string in Python, it's time to put your skills to the test. Try using these techniques to solve a palindrome problem, build a creative word game, or just broaden your Python knowledge! 🧠
Feel free to share your Python string reversal adventures with our tech-savvy community in the comments section below. We can't wait to hear your stories and learn from your experiences! Let the string reversing party begin! 🎊🎉
So what are you waiting for? Start coding and reverse those strings like a pro! Happy coding! 😄💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
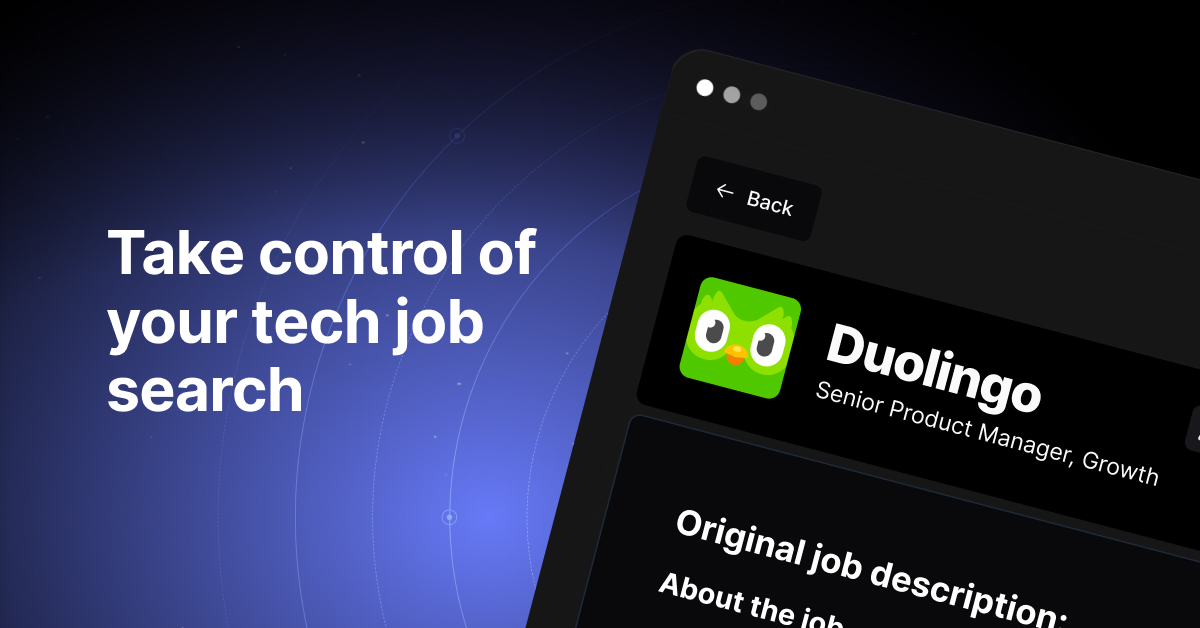