How do I reverse a list or loop over it backwards?
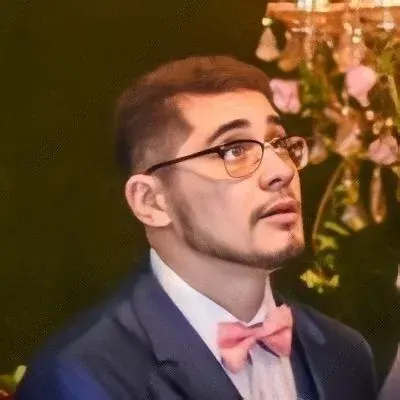
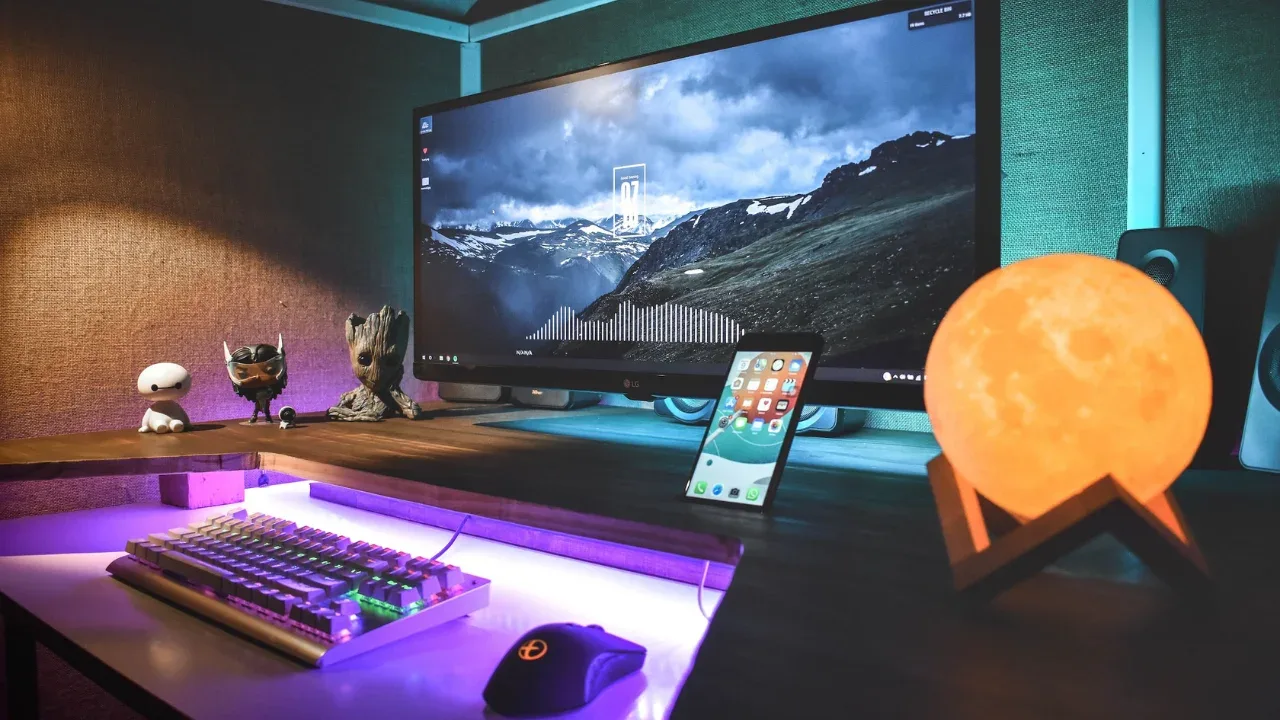
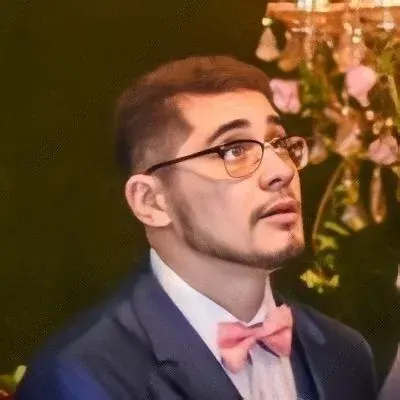
Reversing a List: Loop Your Way Back 🔄
Do you ever find yourself needing to reverse a list or loop over it backwards? You're not alone! This is a common question, especially among Python developers. In this blog post, we'll explore different approaches to tackle this problem and provide you with easy-to-understand solutions. 🚀
The Challenge
Let's start by addressing the challenge at hand. Picture this: you have a list in Python and you want to iterate over it in reverse order. Maybe you need to process the elements of the list in the opposite direction, or perhaps you want to display them differently on a web page. Whatever the reason, reversing a list can be a puzzling task, especially for those newer to programming.
The Easy Solutions
Luckily, Python offers multiple solutions to reverse a list. Let's dive into a few commonly used approaches:
Approach 1: Using the reversed()
Function
Python has a built-in function called reversed()
that allows you to reverse any iterable, including lists. Here's a simple example:
my_list = [1, 2, 3, 4, 5]
reversed_list = list(reversed(my_list))
In this example, we use the reversed()
function to reverse my_list
, and then convert the reversed iterable into a list using the list()
constructor. Voila! Now reversed_list
contains the elements of my_list
in reverse order.
Approach 2: Utilizing Slicing
Another elegant way to reverse a list is by using slicing. Slicing allows you to extract a portion of a list based on specified indices. By specifying a step size of -1, we can traverse the list in reverse order. Take a look at this example:
my_list = [1, 2, 3, 4, 5]
reversed_list = my_list[::-1]
Here, my_list[::-1]
creates a new list that contains all elements of my_list
, but in reversed order. This concise solution is often favored by Pythonistas.
Taking It Further
Now that you have a couple of handy solutions under your belt, you can explore other ways to play around with reversing lists. Here's a bonus tip: You can directly loop over the reversed list using a for
loop. This allows you to iterate over the elements in reverse order without explicitly creating a new variable. Check it out:
my_list = [1, 2, 3, 4, 5]
for item in reversed(my_list):
# Do something with item
print(item)
In this code snippet, we use the reversed()
function as part of a for
loop to directly iterate over the elements of my_list
in reverse order. You can perform any desired operations within the loop, such as printing, modifying the elements, or performing calculations.
Conclusion
Reversing a list or looping over it backward might seem like a tricky task, but with these easy solutions in your toolkit, you'll be able to tackle it effortlessly. By leveraging Python's built-in functions like reversed()
and utilizing slicing techniques, you can manipulate lists in reverse order and perform various operations as desired. So go ahead, give it a try, and unlock new possibilities in your Python programming journey!
Have you encountered any interesting challenges while reversing lists? Share your experiences and questions in the comments below! Let's reverse and rock together! 🎸