How do I return dictionary keys as a list in Python?
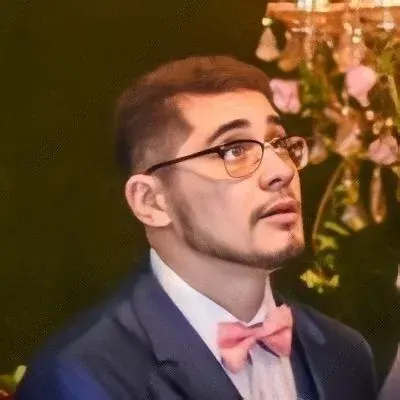
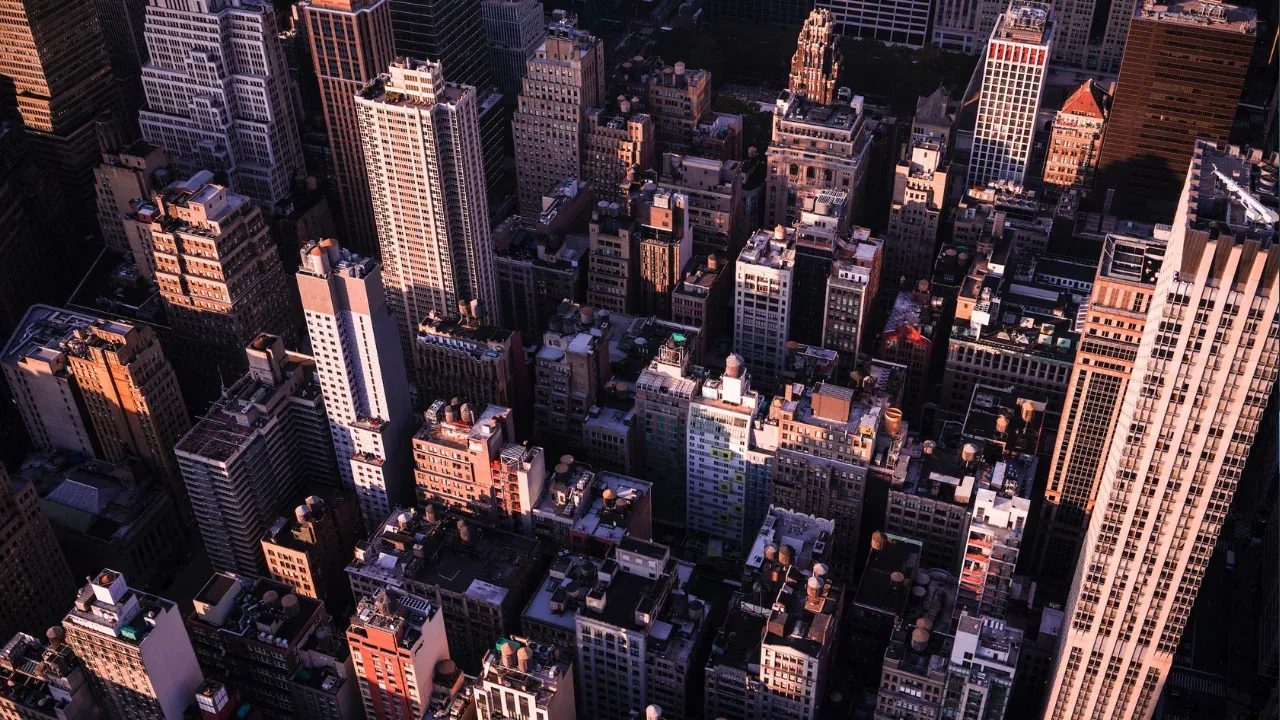
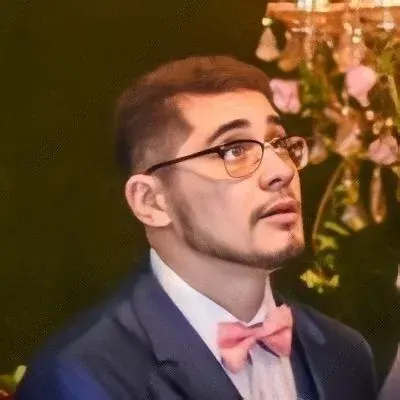
🔑 How to Return Dictionary Keys as a List in Python 🔑
If you've been working with Python dictionaries, you may have come across the need to return the keys as a list. In Python 2.7, it's pretty straightforward:
newdict = {1:0, 2:0, 3:0}
newdict.keys() # Returns [1, 2, 3]
But things change with Python 3.3 and above. When you try to get the keys from a dictionary, you might end up with something like this:
newdict.keys() # Returns dict_keys([1, 2, 3])
So, how can you get a plain list of keys with Python 3? Let's dive into some easy solutions!
Solution 1: Using the list()
Function
One way to get a list of dictionary keys in Python 3 is by using the list()
function. You can simply pass the dict_keys
object to list()
to convert it into a plain list:
keys_list = list(newdict.keys())
print(keys_list) # Returns [1, 2, 3]
By using list()
on the dict_keys
object, you can retrieve the keys as a list that can be easily used in your code.
Solution 2: Using a List Comprehension
Another approach is to use a list comprehension to extract the keys from the dictionary:
keys_list = [key for key in newdict.keys()]
print(keys_list) # Returns [1, 2, 3]
A list comprehension is a concise way to create a new list by iterating over an existing iterable, in this case, the dict_keys
object.
Solution 3: Using the keys()
Method with the list()
Function
If you prefer a one-liner solution, you can combine the keys()
method with the list()
function:
keys_list = list(newdict.keys())
print(keys_list) # Returns [1, 2, 3]
This approach gives you the same result as Solution 1 but in a more compact form.
Now that you know how to return dictionary keys as a list in Python 3, you can easily implement it in your code for various purposes.
Get Creative with Dictionary Keys!
Dictionary keys play a crucial role in organizing and retrieving data in Python. By mastering techniques to handle dictionary keys efficiently, you unlock the power to create dynamic programs and solve complex problems.
So go ahead and experiment with dictionaries! Try out different methods and explore the possibilities. Share your experiences and favorite techniques in the comments below! 🚀🔑
💡 Did you find this post helpful? Let us know by hitting the "Like" button and sharing it with your friends! Don't forget to subscribe to our newsletter for more Python tips and tricks. Happy coding! 😄🐍