How do I read from stdin?
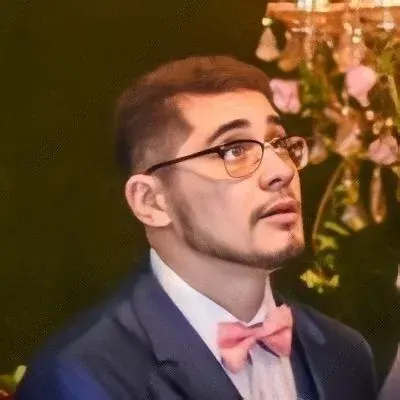
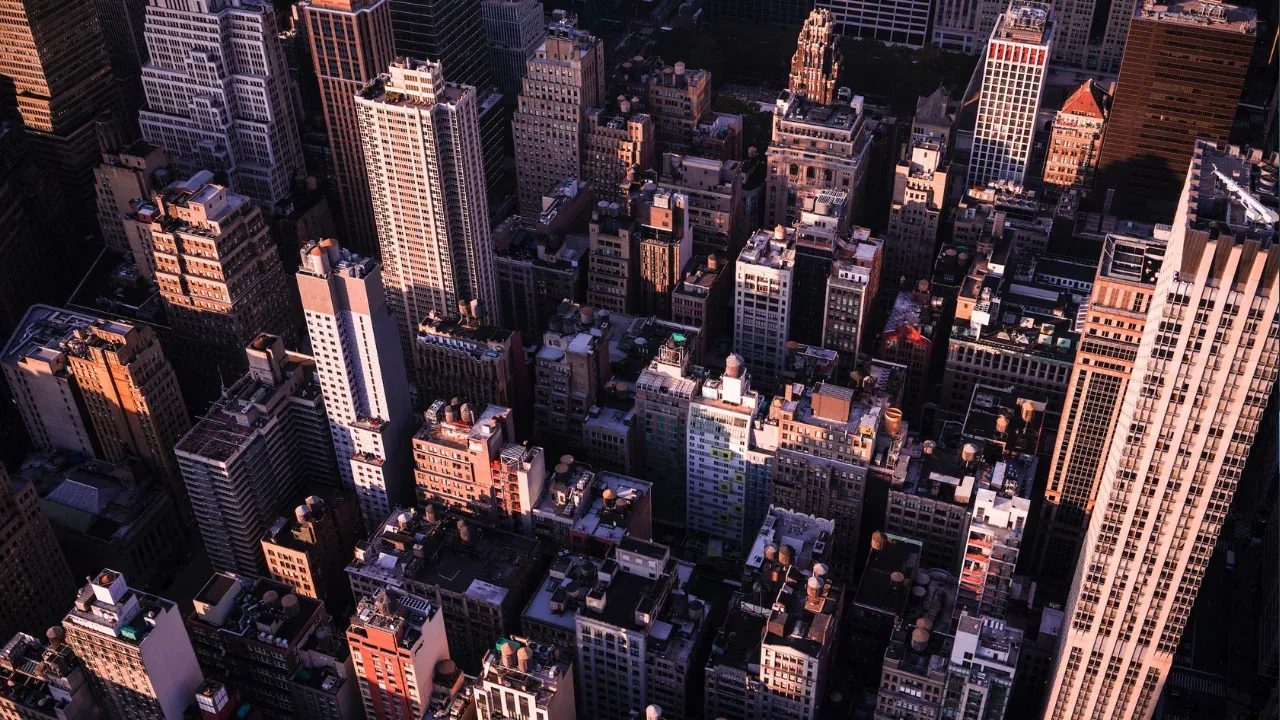
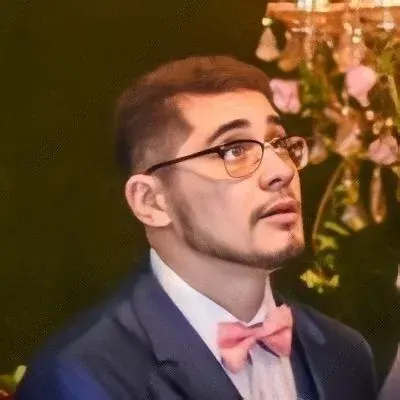
📝 A Complete Guide on How to Read from stdin: Simplified Edition 📝
You may have stumbled upon the question "How do I read from stdin?" while trying to solve code golf challenges or tackling other coding tasks. Don't worry, you're not alone! Understanding and effectively utilizing stdin (standard input) can be a bit tricky, but fear not, because we're here to help you sail through this sometimes perplexing topic. 🚀
🤔 What is stdin?
Before we dive into the nitty-gritty details, let's quickly define what stdin actually is. In simple terms, stdin refers to the standard input stream. It is the default source of input in many programming languages and allows you to gather information during program execution. Typically, this input stream is controlled by the user, whether it's through typing in the terminal or providing input from a file.
📥 Reading Input from stdin
Reading from stdin might vary slightly depending on the programming language you are using. However, the underlying concept remains relatively consistent across languages. Let's explore some examples in popular programming languages to give you a clear understanding.
🐍 Python
In Python, you can utilize the built-in input()
function to read input from the user through stdin. It's as easy as this:
user_input = input("Enter your input: ")
The input()
function prompts the user for input and returns the entered value, which you can then store in a variable.
🔥 JavaScript
JavaScript offers various ways to read input from stdin, but one simple approach is to utilize the readline
module, which is available in both the browser and the Node.js environment. Here's an example:
const readline = require('readline');
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
rl.question('Enter your input: ', (userInput) => {
console.log(`You entered: ${userInput}`);
rl.close();
});
Here, we use the readline
module to create an interface for reading input from stdin. The rl.question()
function prompts the user for input, and once provided, the callback function receives the entered value.
📝 Other Programming Languages
While we've highlighted examples in Python and JavaScript, it's important to note that reading from stdin is a fundamental concept and can be accomplished in numerous programming languages. Familiarize yourself with your language's documentation or consult relevant tutorials and guides to learn how to read input from stdin in your preferred language.
🧩 Addressing Common Issues
Now that you have a basic understanding of reading from stdin, let's tackle some commonly encountered issues and how to overcome them.
🚫 Blank Input Handling
Consider a scenario where you want to read multiple inputs from the user, and they might provide a blank input to indicate the end of the inputs. To handle this, you can use a simple loop that reads until a blank input is encountered. Here's an example in Python:
inputs = []
while True:
user_input = input("Enter your input (blank to exit): ")
if not user_input:
break
inputs.append(user_input)
In this example, the loop continuously prompts for input and checks if the input is blank. If it is, the loop breaks, and the user inputs are stored in the inputs
list.
📂 File Input Instead of stdin
Sometimes, instead of relying on direct user input through the terminal, you may need to read input from a file. You can redirect file content to stdin using the <
symbol in most command-line interfaces. Here's an example in C++:
./my_program < input.txt
In this example, the input.txt
file's content will be passed as input to my_program
.
💡 Engage with the Community!
Mastering the art of reading from stdin is not only essential for code golf challenges but can greatly enhance your programming skills overall. We encourage you to practice, experiment, and get involved with coding communities to explore different use cases and gain insights from your peers.
Share your experiences and any tips or tricks you've discovered along the way in the comments section below. Let's learn, grow, and write amazing code together! 🌟
Keep coding and stay curious! 🤓💻✨