How do I read a large csv file with pandas?
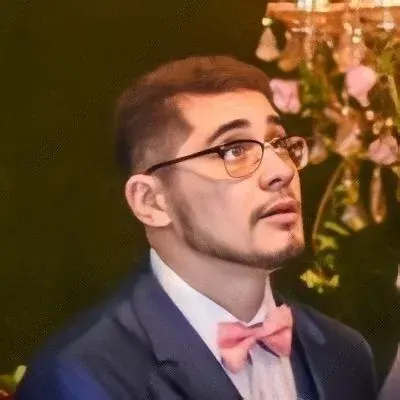
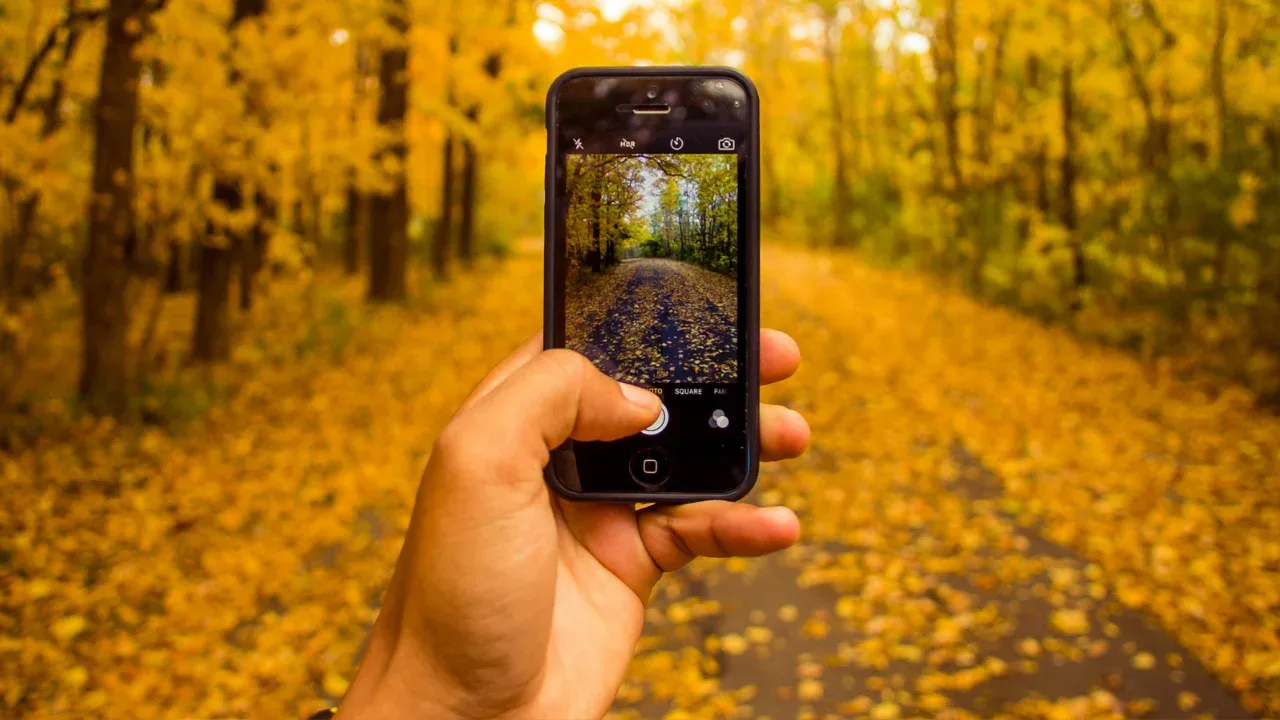
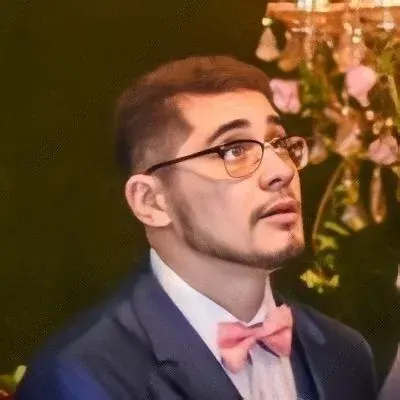
How to Tackle Reading Large CSV Files with πͺPandasπͺ
So, you've got a big π CSV file (approximately 6 GB) that needs to be read into your πΌ Pandas dataframe. You're all set to use the read_csv
function, but there's just one π₯ tiny problem β you keep running into a pesky MemoryError
. Frustrating, right? π
Don't worry! In this guide, we'll walk you through common issues and provide easy solutions to help you conquer this memory hurdle. By the end, you'll be able to read and handle large CSV files with ease. Let's dive right in! π
β οΈ Common Issue: MemoryError
The MemoryError
occurs when Pandas runs out of memory while attempting to load the entire CSV file into memory. This issue typically arises with large datasets, as the memory required to store the entire file exceeds the available resources. π§ π₯
π‘ Solution 1: Read Only Required Columns
One simple solution is to read only the columns you actually need from the CSV file. This can help reduce memory consumption. Here's how you can do it with Pandas:
# Specify the columns you want to read
columns = ['column1', 'column2', 'column3']
# Read the CSV file with the selected columns
df = pd.read_csv('your_file.csv', usecols=columns)
By specifying the usecols
parameter, you can select the specific columns you want to load into memory. This way, you discard the unnecessary ones and save memory space. π
π‘ Solution 2: Chunking the CSV File
Another approach is to load the CSV file in smaller chunks, rather than loading the entire file at once. This way, you can process the data in manageable pieces.
# Define the chunk size (adjust as per your requirements)
chunk_size = 10000
# Create an empty list to store each chunk
chunks = []
# Load the CSV file in chunks
for chunk in pd.read_csv('your_file.csv', chunksize=chunk_size):
chunks.append(chunk)
# Concatenate the chunks into a single dataframe
df = pd.concat(chunks)
Breaking down the file into chunks allows you to process data in a more memory-efficient manner. You can adjust the chunk_size
based on your available resources and the size of the CSV file. π
π‘ Solution 3: Specifying Data Types
By default, Pandas tries to infer the data types for each column in the CSV file, which can sometimes lead to excess memory usage. You can specify the data types explicitly to optimize memory consumption. π
# Define the column data types
dtypes = {'column1': np.int32, 'column2': np.float64, 'column3': str}
# Read the CSV file with specified data types
df = pd.read_csv('your_file.csv', dtype=dtypes)
By providing explicit data types for each column in the dtype
dictionary, Pandas avoids the need to infer them. This reduces memory overhead and speeds up the loading process.
π£ Take Action!
Now that you have learned some effective strategies to tackle large CSV files with Pandas, it's time to put your newfound knowledge to use. π
Consider the following questions to solidify your understanding:
What is the most suitable solution for reading a large CSV file with memory constraints?
How can you optimize memory usage when reading a CSV file with multiple data types?
Engage with fellow readers by sharing your answers in the comments section below. Let's learn together! π
Remember, working with large datasets requires careful considerations, but with the power of Pandas and these solutions in your toolbox, you are now better equipped to handle massive CSV files like a pro! πͺπΌ
Happy coding! π»πΌβ¨