How do I profile a Python script?
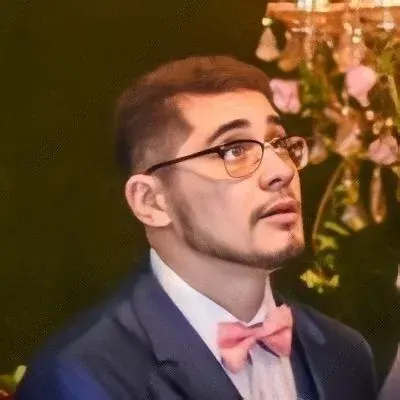
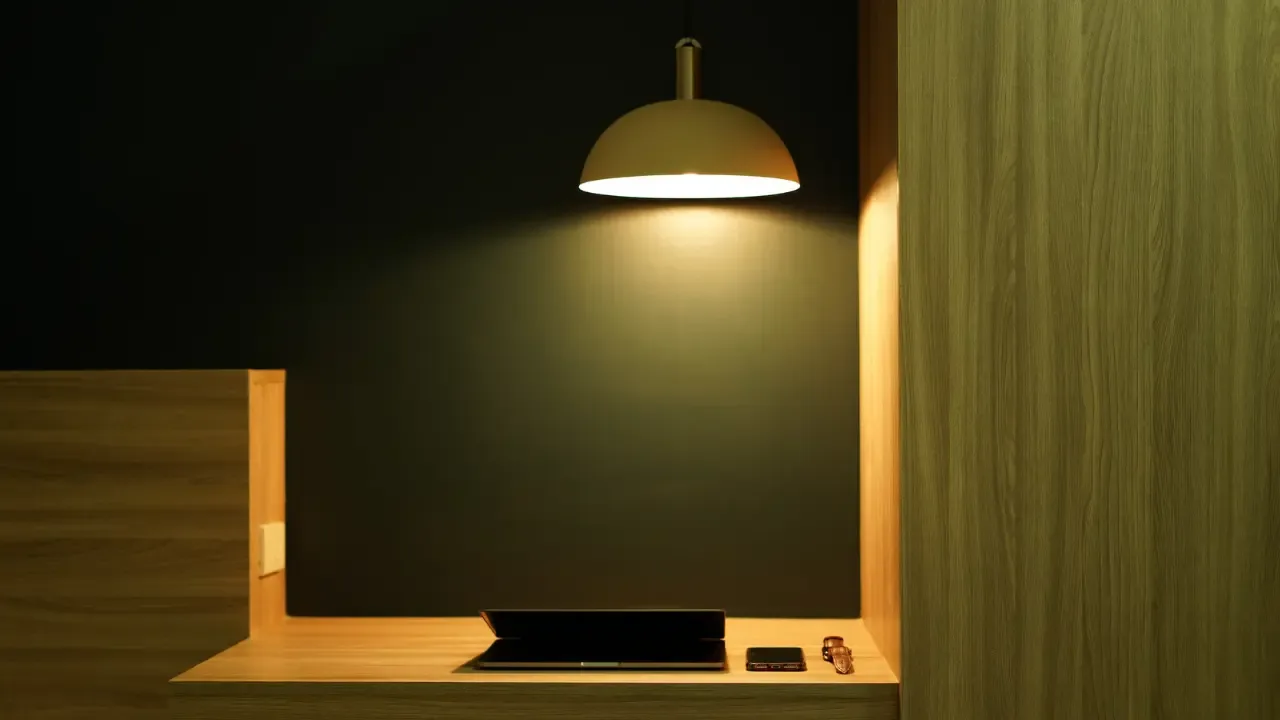
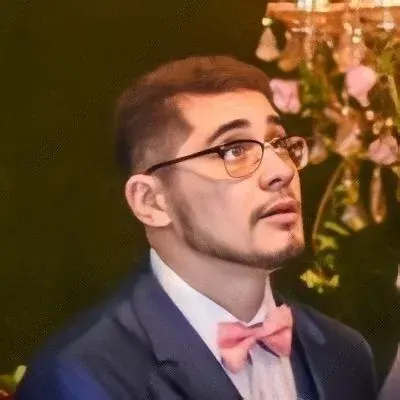
🔎 How do I profile a Python script? 🐍
Are you tired of guessing which part of your Python script is causing the slowdown? Is your code taking forever to run and you don't know why? 🤔 Don't worry, we've got you covered! In this blog post, we'll discuss how to effectively profile your Python script and find those performance bottlenecks. 💪
But first, let's understand the problem. ⚠️
When participating in coding contests like Project Euler, or simply optimizing your code, it's essential to measure the execution time accurately. 🕒 Adding timing code to the "main" section of your script might work, but let's be real, it's not the most elegant solution. 🙈
So, what's a good way to profile how long a Python program takes to run? Let's dive into some awesome solutions! 🎉
The Solution: Profiling Techniques
Using the timeit module 🕐
Python's built-in 'timeit' module provides a simple way to measure the execution time of small code snippets. You can either use it directly from the command line or within your script. For example, to measure the execution time of a function called 'my_function', you can use the following code snippet:
import timeit def my_function(): # Code to be executed pass execution_time = timeit.timeit(my_function, number=1) print(f"Execution time: {execution_time} seconds")
By default, 'timeit.timeit' runs the code 1 million times to provide accurate results. Feel free to adjust the
number
parameter based on your needs.Using the cProfile module 🔬
Python's 'cProfile' module allows you to profile your entire script or specific functions in detail. It provides a comprehensive analysis of the time taken by each function, the number of calls made, and more. To start profiling your script using 'cProfile', you can follow these steps:
import cProfile # Code to be profiled cProfile.run('main()') # Replace 'main()' with your script's main function
After execution, cProfile will provide a detailed breakdown of the execution time for each function. This valuable information will help you pinpoint the exact areas that need optimization.
Using third-party libraries 📚
If you need even more powerful profiling tools, you can leverage third-party libraries such as 'line_profiler' or 'memory_profiler'. These tools give you a line-by-line breakdown of the execution time or memory usage, respectively. You can install these libraries using pip and decorate the functions you want to profile with special annotations.
from line_profiler import LineProfiler @profile def my_function(): # Code to be profiled pass my_function() # Execute the function # After execution, profiling results will be displayed in the console
Third-party libraries like these offer advanced profiling capabilities and can be incredibly useful when optimizing complex Python scripts.
Call-to-Action: Optimize your Python script like a pro! 💻
Now that you know how to profile your Python script, it's time to put this knowledge into practice. Start by identifying the performance bottlenecks in your code, optimize them, and witness the speed boost! ⚡️
Don't forget to share your success story with us in the comments section below! We'd love to hear about the improvements you made and the impact it had on your Python projects. 🚀
Happy profiling! 😎✨