How do I print to stderr in Python?
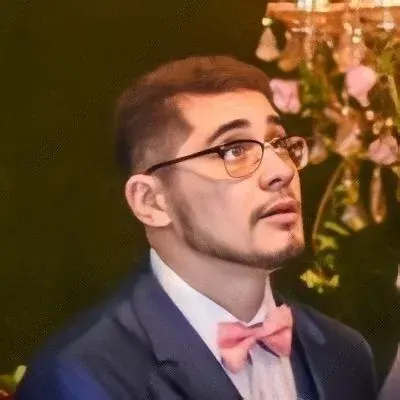
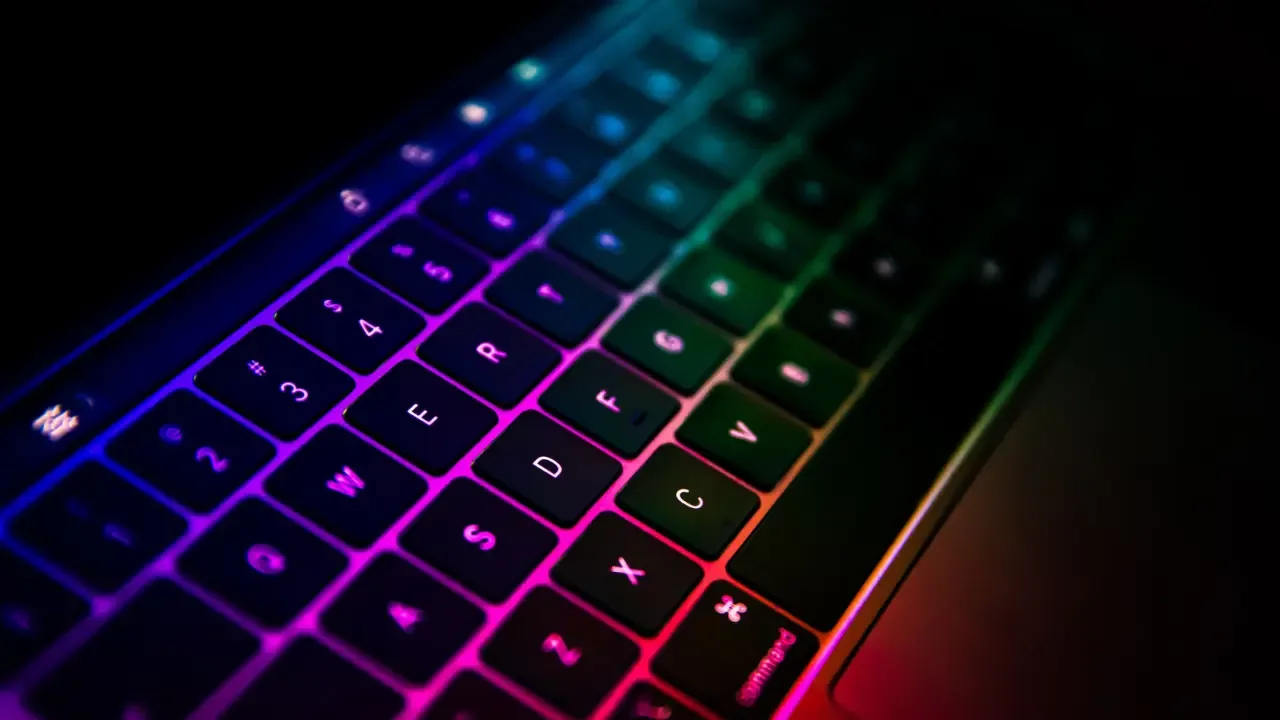
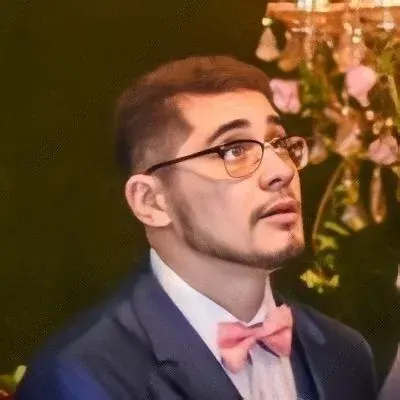
💻 How to Print to stderr in Python? 🐍
So, you want to print to stderr in Python? No worries, we've got you covered! In this blog post, we'll address common issues and provide easy solutions to help you master this skill. Let's dive in!
Understanding the Different Methods
There are several ways to print to stderr in Python. Let's go through each method and explore their differences:
Method 1: print >> sys.stderr, "spam"
This method is available only in Python 2. It uses the print
statement along with the >>
syntax to redirect the output to sys.stderr
. Here's an example:
print >> sys.stderr, "spam"
Method 2: sys.stderr.write("spam\n")
In this method, we directly write to sys.stderr
using the write
function. Optionally, we can append the newline character (\n
) to indicate the end of the line. Check out the example below:
sys.stderr.write("spam\n")
Method 3: os.write(2, b"spam\n")
Here, we use the os.write
function to write directly to file descriptor 2, which represents stderr. The b"spam\n"
syntax is used to create a byte string. Take a look at the following snippet:
os.write(2, b"spam\n")
Method 4: print("spam", file=sys.stderr)
By importing from __future__ import print_function
, we can use the Python 3 style print
function. We pass sys.stderr
as the file
argument to redirect the output to stderr. Here's an example:
from __future__ import print_function
print("spam", file=sys.stderr)
Choosing the Preferred Method
Now that we've explored the different methods, you might be wondering which one is the best choice. 🤔 Well, it depends on your specific use case.
If you're working with Python 2, the first method (
print >> sys.stderr, "spam"
) is a valid option. However, keep in mind that this method is not compatible with Python 3.If cross-compatibility is a concern or you're already using Python 3, the fourth method (
print("spam", file=sys.stderr)
) is the way to go. It provides a clean and consistent approach across Python versions.As for the second and third methods (
sys.stderr.write
andos.write
), they offer more flexibility but may require additional handling, such as appending the newline character manually.
Remember, it's important to choose the method that aligns with your project's Python version and requirements.
Take Action and Level Up! 🚀
Now that you have a solid understanding of how to print to stderr in Python, it's time to put your knowledge into practice! Choose the method that suits your needs and start coding.
If you found this blog post helpful, share it with your friends and colleagues who might benefit from it. Also, comment below to let us know your preferred method or any other Python-related topics you'd like us to cover in the future.
Keep exploring, keep growing! Happy coding! 😉👩💻👨💻
[Your Tech Blog Name]
P.S. Don't forget to follow us on Twitter and Facebook for more exciting tech tips and tutorials!