How do I print an exception in Python?
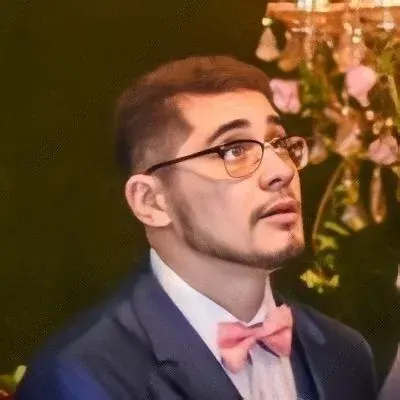
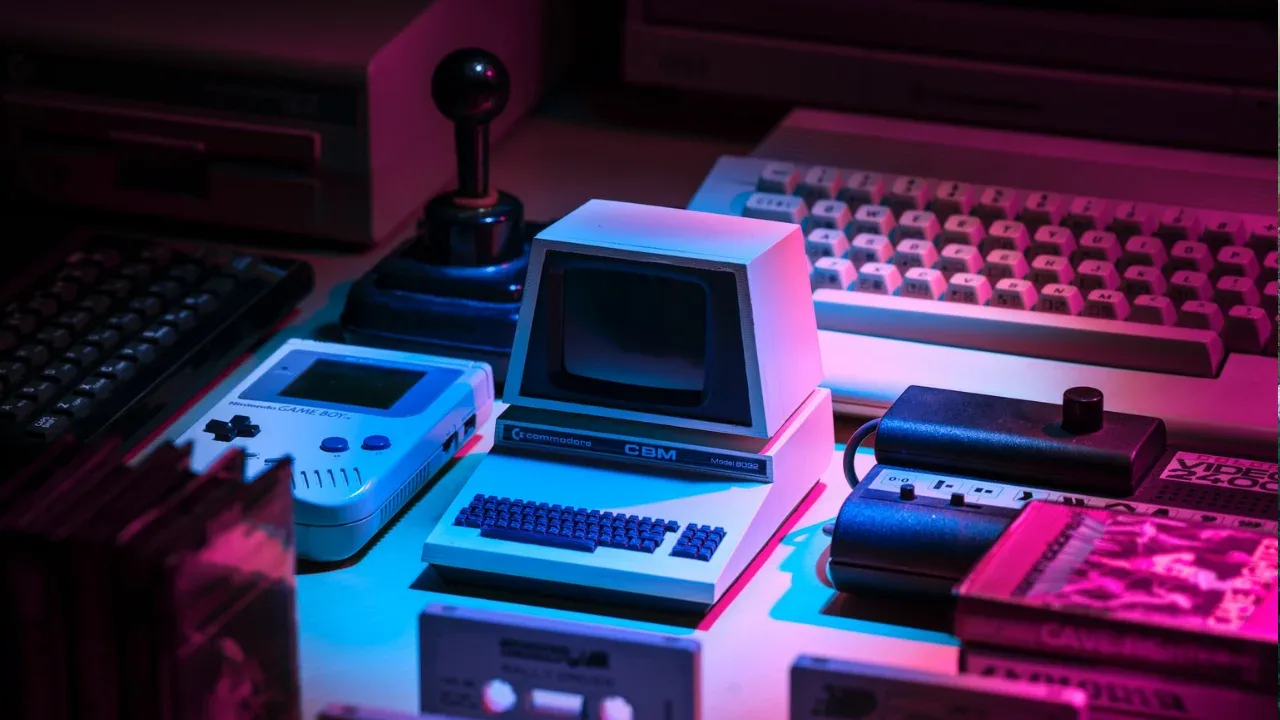
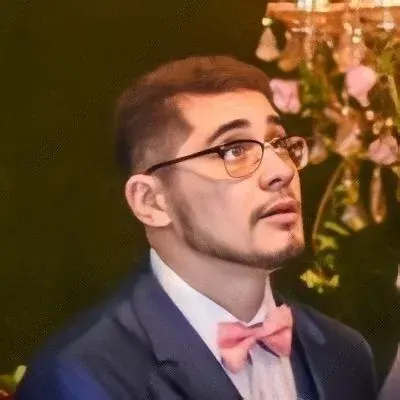
🖨️ How to Print an Exception in Python 🐍
So, you're working on your Python code and suddenly, an exception error pops up. 😱 We've all been there! But don't worry, printing the error or exception in the except
block can help you understand and troubleshoot the problem more effectively. In this blog post, we'll explore how you can easily print exceptions in Python and provide some useful tips to make your debugging process smoother. 😉
🙋♂️ Why Print Exceptions?
Exceptions occur when something unexpected happens in your code. They are a valuable tool for error handling and debugging. By printing these exceptions, you can get more information about what went wrong, making it easier to identify and fix the issue. So, let's dive in and see how it's done! 🏊♀️
📝 The Usual Mistake
You might be tempted to go with the following approach to print an exception:
try:
# Your code here
except:
print(exception)
But hold on! 😮 This won't quite give you what you're looking for. The problem here is that exception
is not a defined variable, so Python will raise a NameError
when you try to print it. Let's see a more optimal solution next. 💡
✅ Printing the Exception Properly
To print an exception correctly, we need to access the exception object itself. This can usually be done using the as
keyword and assigning it to a variable. Here's the modified version of the code:
try:
# Your code here
except Exception as e:
print(e)
By using Exception as e
, we're catching any exception that occurs and assigning it to the variable e
. Then we simply print out e
to see the error message. 🎯
💡 Additional Tips
Now that you know how to print exceptions properly, here are a few extra tips to enhance your debugging skills:
Print the exception type: In addition to the error message, printing the type of exception can give you more insights into the issue. You can do this by calling
type(e)
. For example:try: # Your code here except Exception as e: print(type(e), e)
Logging the exception: Instead of just printing the exception, you can utilize Python's logging module to record the exception in a file for later analysis. This is especially useful in production environments. Here's a simple example:
import logging try: # Your code here except Exception as e: logging.exception("An error occurred:")
This will create a log file with the error message and a stack trace for further investigation.
🙌 Your Turn!
Now that you have learned how to print exceptions in Python, it's time to put that knowledge into action! Take a moment to review your existing code and consider adding exception handling with proper printing.
If you have any questions or insights to share, please leave a comment below. Let's help each other become better Python developers! 🚀
Happy coding! 😄✨