How do I pass a variable by reference?
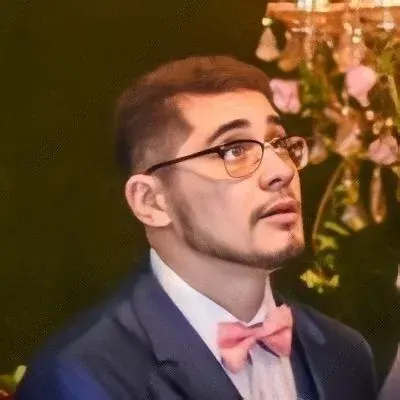
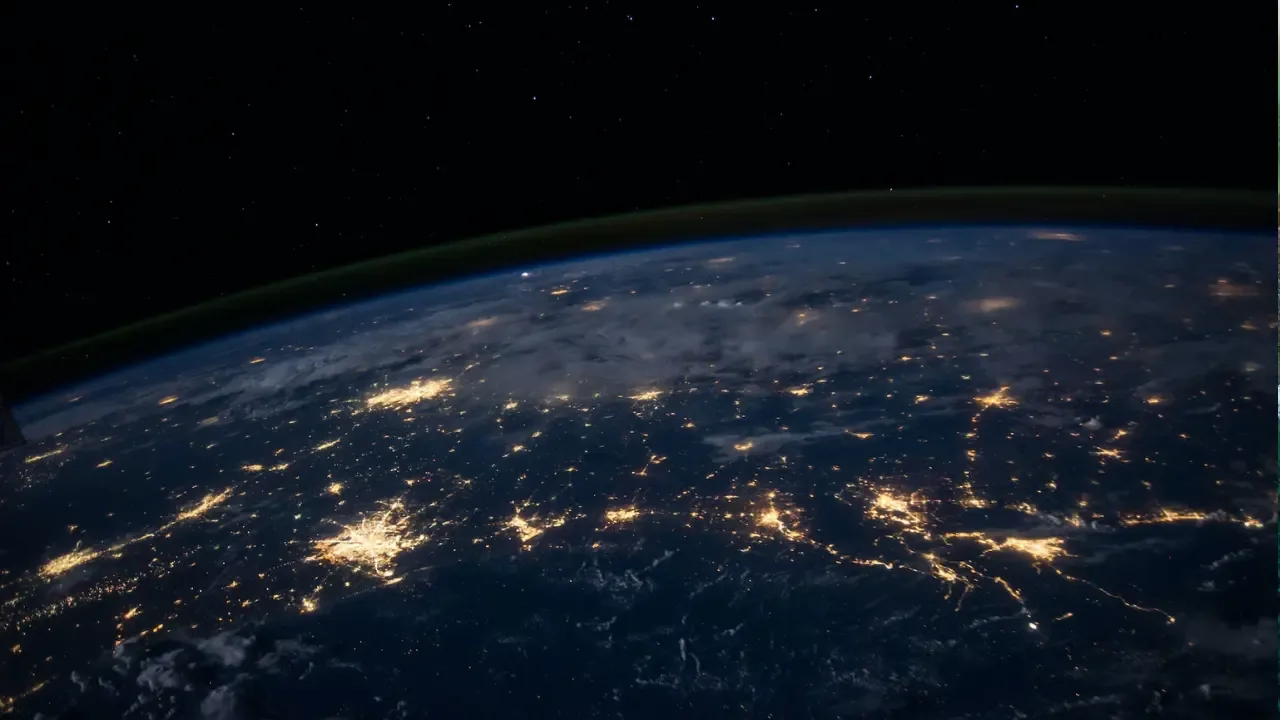
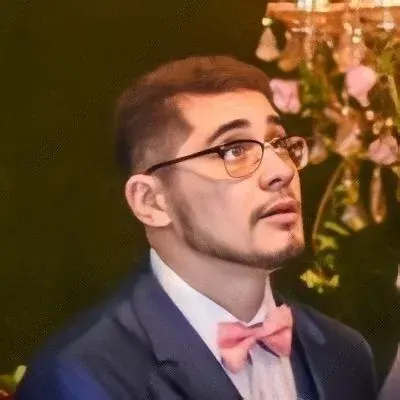
How to Pass a Variable by Reference: A Guide for Python Developers 👩💻🐍
So, you've encountered the age-old question in Python: "How do I pass a variable by reference?" 🤔
You might have noticed that even though you attempted to modify the variable, the change didn't persist outside of the function. In Python, parameters are indeed passed by value by default. But worry not! We have some nifty workarounds to achieve the effect of pass-by-reference. Let's dive in! 💪
Understanding the Pass-by-Reference Concept 📚
Before we jump into the solutions, let's clarify what pass-by-reference means. In pass-by-reference, instead of passing the value of a variable, you pass the reference (memory address) of the variable. This allows modifications to the variable to be visible outside the scope of the function.
Approach 1: Using Mutable Objects 📦
Python handles mutable and immutable objects differently. Mutable objects, such as lists, dictionaries, and sets, can be modified in place. This property can help us achieve the desired effect of pass-by-reference. 🔄
Here's an updated version of your code using lists to demonstrate the concept:
class PassByReference:
def __init__(self):
self.variable = ['Original']
self.change(self.variable)
print(self.variable)
def change(self, var):
var[0] = 'Changed'
By passing a list as the variable, any modifications made to the list will be visible outside the function. Hence, the output would be 'Changed'
as desired! 🎉
Approach 2: Using Classes and Objects 🏗️
Another way to mimic pass-by-reference in Python is by using classes and objects. Objects are passed by reference, allowing us to modify their attributes and have the changes persist.
Let's take a look at an example:
class VariableHolder:
def __init__(self):
self.variable = 'Original'
class PassByReference:
def __init__(self):
self.container = VariableHolder()
self.change(self.container)
print(self.container.variable)
def change(self, obj):
obj.variable = 'Changed'
In this example, we use a separate class VariableHolder
to hold our variable. By passing the container object, we can modify its attribute within the change
method. Consequently, the output will be 'Changed'
.
Conclusion and Call-to-Action 🏁📣
Passing variables by reference is not directly supported in Python, which defaults to pass-by-value. However, by using mutable objects like lists or objects with attributes, we can achieve similar effects. 🚀
Next time you encounter a scenario where you need to pass variables by reference, remember these workarounds to save the day! 💡
If you found this guide helpful, don't forget to share it with your fellow Python developers! 😄
Got any other Python questions or coding dilemmas on your mind? Leave a comment below, and let's start a conversation! 👇🤓