How do I parse a string to a float or int?
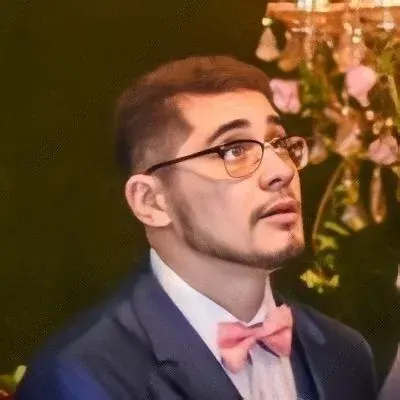
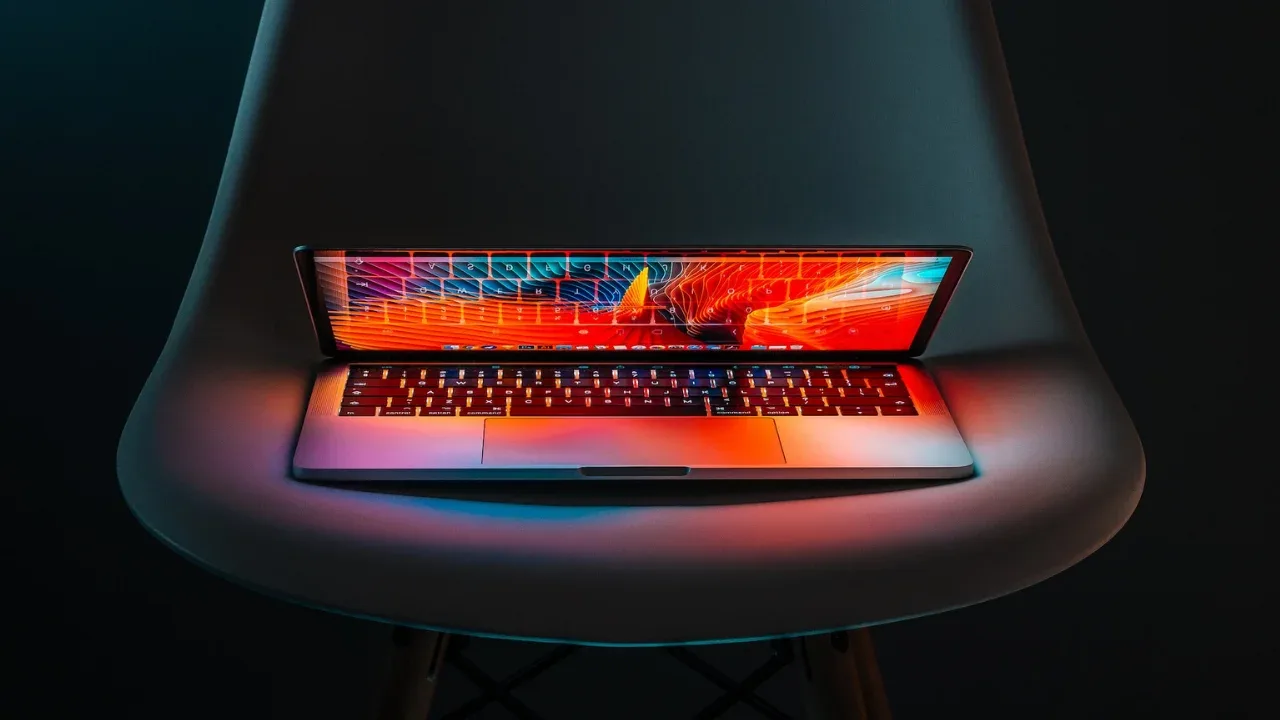
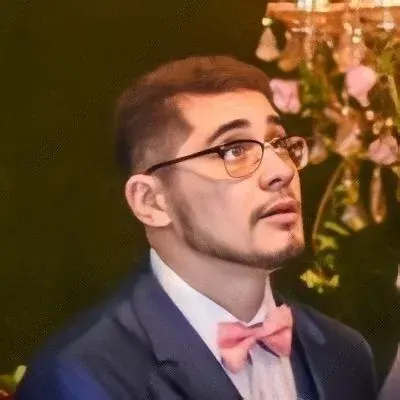
How to Parse a String to a Float or Int in Python
So you have a string and you want to convert it to a float or int in Python? No worries, we've got you covered! In this blog post, we'll address common issues and provide easy solutions to parsing strings to floats or ints. 🚀
Converting a String to a Float
Let's start by tackling the first question: how to convert a string to a float.
string_number = "545.2222"
float_number = float(string_number)
print(float_number)
Output:
545.2222
As simple as that! You can use the float()
function in Python to parse a string containing a float number and convert it into an actual float datatype.
Converting a String to an Int
Now, let's move on to converting a string to an integer.
string_number = "31"
int_number = int(string_number)
print(int_number)
Output:
31
Just like with floats, you can use the int()
function to parse a string representing an integer and convert it into an int datatype.
Handling Errors
It's important to note that the code examples above assume that the string being parsed is a valid number representation. However, if the string contains any non-numeric characters, you will encounter a ValueError
exception.
To handle this scenario, you can wrap the conversion code in a try-except block to catch the exception and handle it gracefully.
string_number = "not a number"
try:
float_number = float(string_number)
print(float_number)
except ValueError:
print("Oops! That was not a valid number.")
Output:
Oops! That was not a valid number.
Your Turn!
Now that you know how to parse strings to floats and ints in Python, it's time to put your newfound knowledge into practice. Try converting different strings to see the results!
If you have any questions or encounter any issues, feel free to leave a comment below. We're here to help! 😊
Conclusion
In this blog post, we have learned how to easily parse a string into a float or int in Python. By using the float()
and int()
functions, you can convert strings representing numbers into their respective datatypes.
Remember to handle potential errors gracefully to ensure your code doesn't break when encountering invalid inputs.
Now go ahead and start parsing those strings like a pro! Happy coding! 👩💻👨💻
If you found this blog post helpful, don't forget to share it with your friends and colleagues!