How do I pad a string with zeroes?
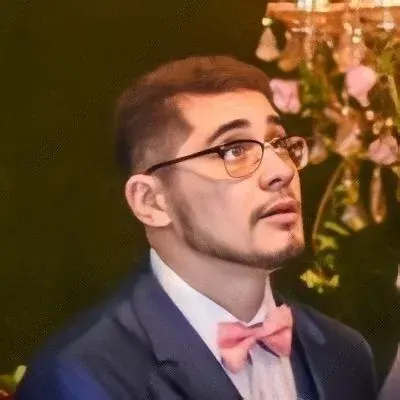
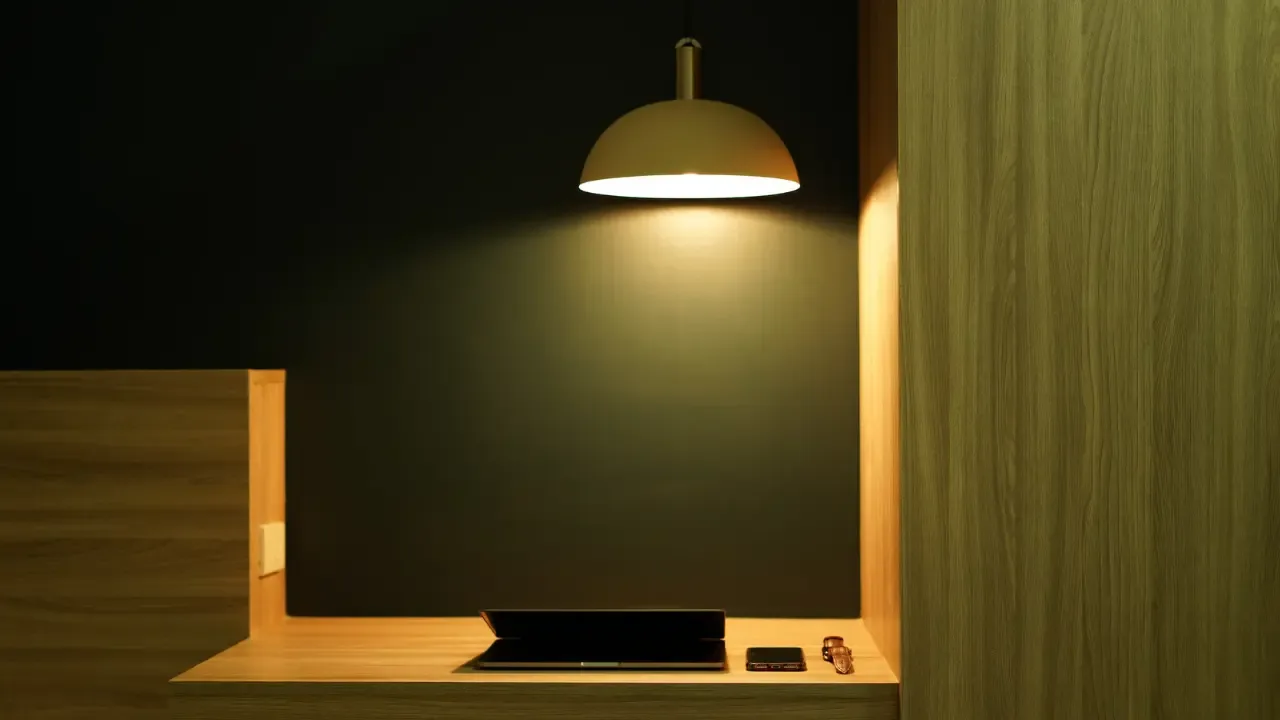
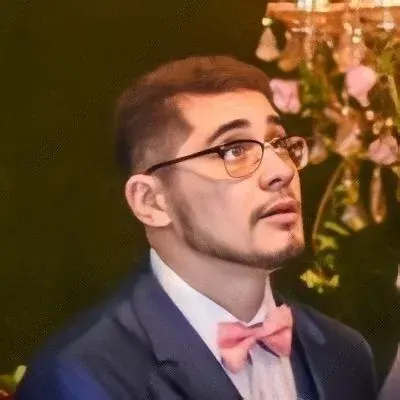
π₯ Adding Zeroes in Style: A Guide to Padding Strings π₯
So, you've got a numeric string, and you want to give it that extra boost by padding it with zeroes. Fear not! We've got your back with this guide on how to do it like a pro. Get ready to impress your audience with beautifully formatted strings. π
What's the Deal with Padding?
Before we dive into the specifics, let's understand the need for padding. Padding adds extra characters (in this case zeroes) to a string to make it a specific length. Itβs commonly used to maintain a consistent visual alignment or to meet specific requirements in various programming scenarios.
Common Issue: The Zeroes Are Missing!
One common issue when padding a numeric string is that the zeroes seem to vanish into thin air. Ouch! But fret not, we're here to help.
Easy Solution: Using String Functions
One way to pad a numeric string with zeroes is by utilizing the power of string functions. Let's take a look at a couple of examples using popular programming languages:
β¨ Example 1: JavaScript
const originalString = "42";
const desiredLength = 6;
const paddedString = originalString.padStart(desiredLength, "0");
console.log(paddedString); // Output: "000042"
In this example, we use the padStart
function in JavaScript to add zeroes to the left of the original string. The function takes two arguments: the desired length and the character to pad (in our case, "0").
β¨ Example 2: Python
original_string = "42"
desired_length = 6
padded_string = original_string.zfill(desired_length)
print(padded_string) # Output: "000042"
Python's zfill
method provides a similar solution. It pads the string with zeroes to the left, making it the specified length.
Level Up: Give the User Control!
You've successfully padded your numeric string with zeroes. But wouldn't it be cool if users could control the length of the padding dynamically? We've got just the thing for you!
Advanced Solution: Interactive Padding
Incorporating interactive padding into your code allows users to customize the length of the padding. Here's an example using JavaScript:
function padWithZeroes(originalString, desiredLength) {
return originalString.padStart(desiredLength, "0");
}
const userInput = prompt("Enter a numeric string:");
const maxLength = 10;
const paddedString = padWithZeroes(userInput, maxLength);
console.log(paddedString);
In this example, we create a function called padWithZeroes
that takes two parameters: the original string and the desired length. The user enters a numeric string using the prompt
function, which is then padded with zeroes using our custom function.
π£ Your Turn to Shine!
You've now mastered the art of padding strings with zeroes. But don't stop here! Experiment with different languages and explore other creative ways to enhance your strings. Get those creative juices flowing!
Share your favorite padding techniques or any other insights with us in the comments below. We'd love to hear from you! π