How do I move a file in Python?
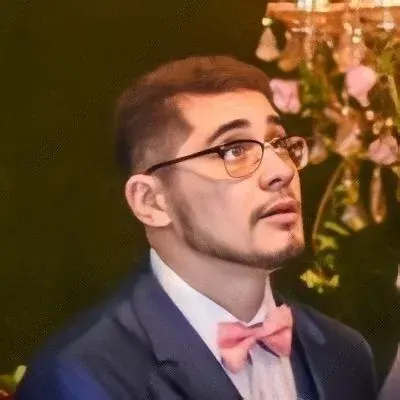
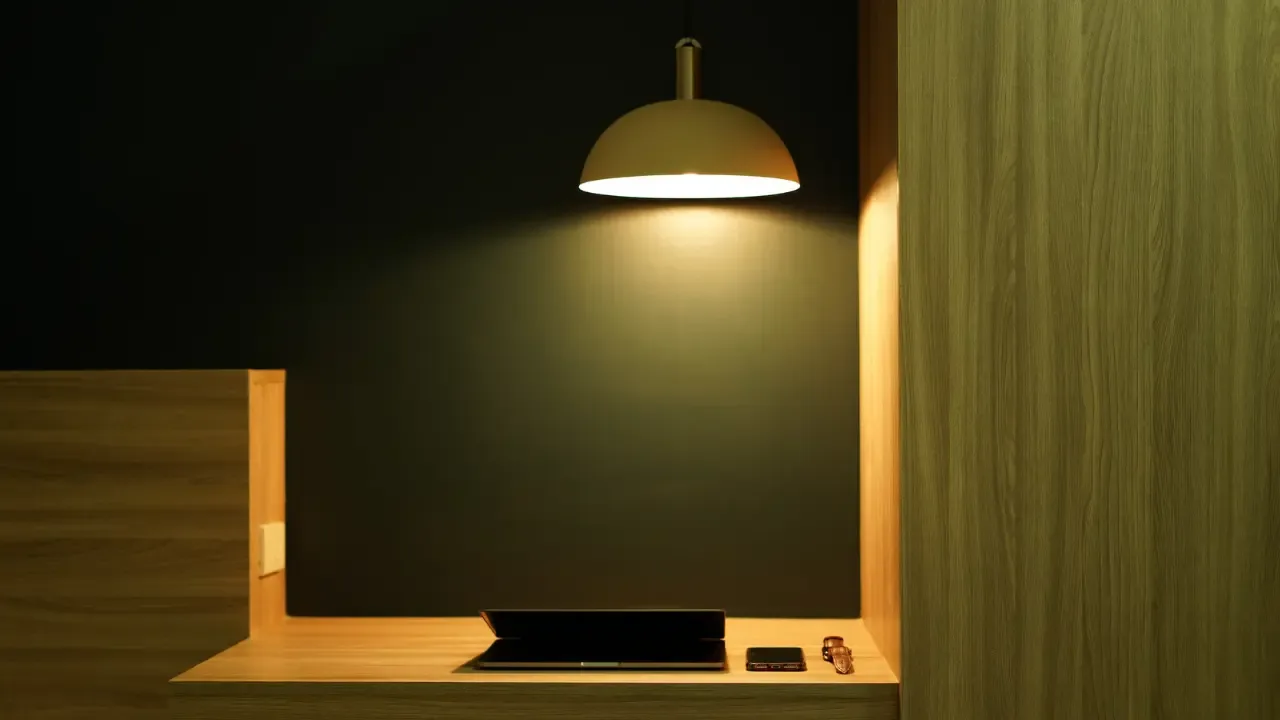
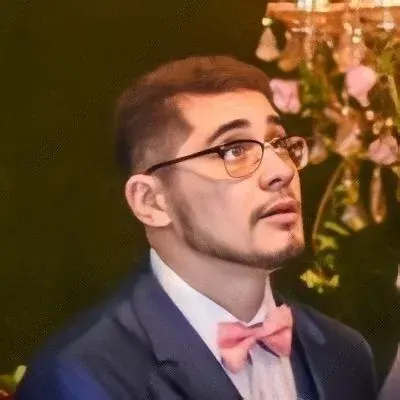
How to Move a File in Python 🐍
So, you want to move a file in Python, huh? No problemo! Whether you're a Python newbie or a seasoned programmer, this guide will help you effortlessly move files with ease! 📂✨
Common Issues
Before we dive into the solutions, let's address some common issues you might encounter while trying to move a file in Python:
File Not Found Error: Make sure that the file you're trying to move actually exists in the specified location. Double-check the paths to ensure they are correct.
Permission Denied Error: You might encounter this error if you don't have proper permissions to access or modify the file you're trying to move. Ensure that you have the necessary permissions before proceeding.
File In Use Error: If the file is currently being used by another process, you won't be able to move it until it's released. Be sure to close any open file handles before attempting to move the file.
Easy Solutions
Now that we've covered the common issues, let's explore some easy solutions to move a file in Python. There are several ways to achieve this, but we'll focus on two popular methods:
1. Using the shutil
module
The shutil
module in Python provides a high-level interface for file operations. Here's how you can use it to move a file:
import shutil
source = "path/to/current/file.foo"
destination = "path/to/new/destination/for/file.foo"
shutil.move(source, destination)
That's it! With just a few lines of code, you can effortlessly move a file in Python using the shutil
module. Easy peasy, right? 😎
2. Using the os
module
Another way to move a file in Python is by using the os
module. Here's an example:
import os
source = "path/to/current/file.foo"
destination = "path/to/new/destination/for/file.foo"
os.rename(source, destination)
The os.rename()
function in the os
module is capable of renaming a file and moving it to a different directory. Simple and straightforward! 🚀
Call-to-Action
Now that you know how to move a file in Python, it's time to put your newfound knowledge to use! Go ahead and give it a try in your next Python project. 📦💨
If you have any questions or other Python-related topics you'd like me to cover, feel free to leave a comment below. Remember, sharing is caring, so don't forget to share this post with your fellow Pythonistas! 🙌✨
Happy coding! 🐍💻