How do I merge two dictionaries in a single expression in Python?
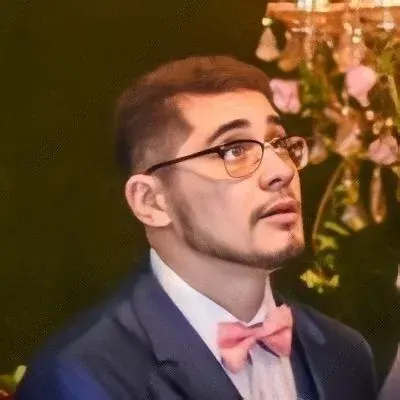
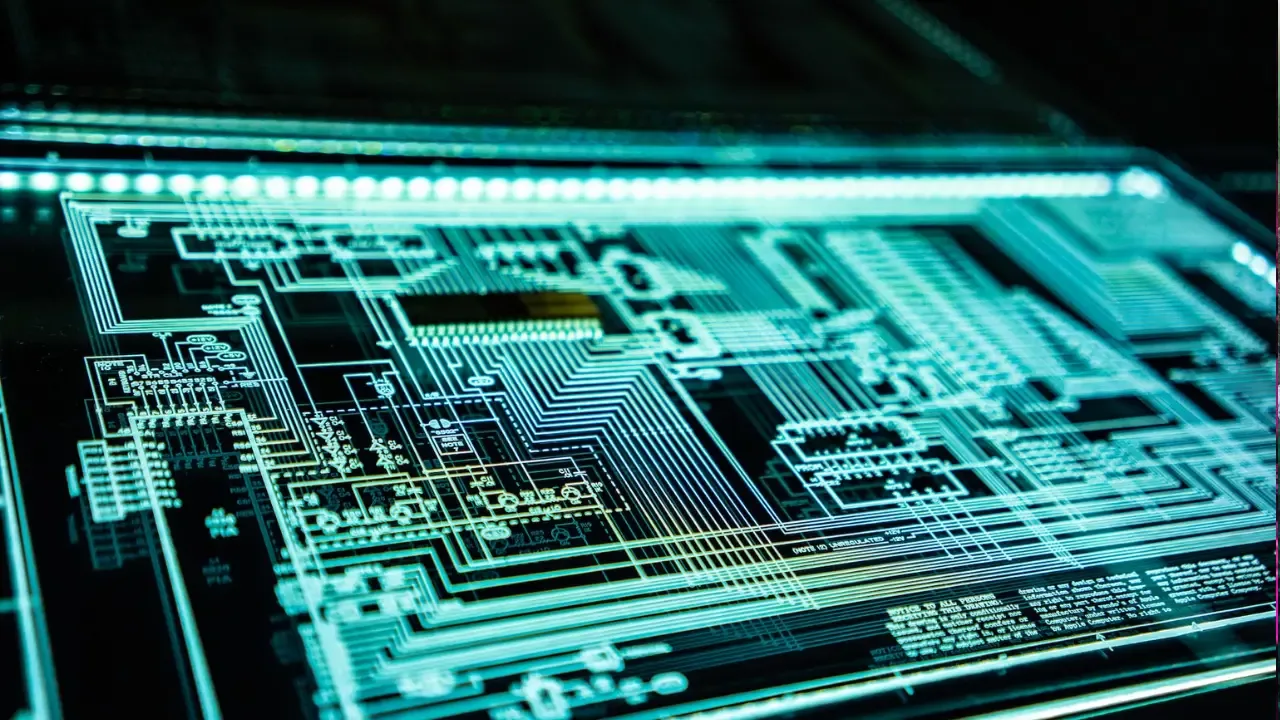
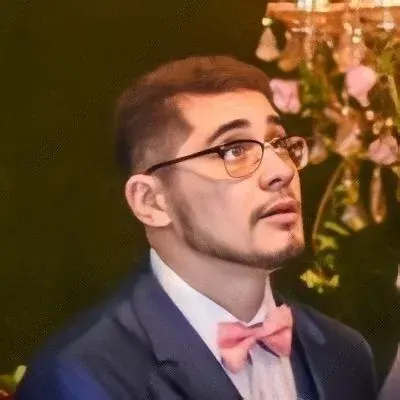
š Tech Blog: How to Merge Two Dictionaries in a Single Expression in Python
š§āāļø Welcome to another exciting blog post where we dive into the world of Python programming! š Today, we're going to tackle the question of how to merge two dictionaries into a single expression. This nifty trick can save you time and make your code more concise. Let's get started! š»
š§ The Problem: Merging Two Dictionaries
Imagine you have two dictionaries, x
and y
, and you want to combine them into a new dictionary, z
. However, there's a catch! If a key exists in both dictionaries, you want to keep the value from y
. š
Here's the initial code snippet that showcases the problem:
x = {'a': 1, 'b': 2}
y = {'b': 3, 'c': 4}
z = merge(x, y)
print(z)
# Output: {'a': 1, 'b': 3, 'c': 4}
As you can see, the key 'b'
exists in both x
and y
. We want the final dictionary z
to reflect this by keeping the value 'b': 3
from y
. The expected output should be {'a': 1, 'b': 3, 'c': 4}
.
š” The Solution: A Single Expression to Merge Dictionaries
Python offers a concise solution to this problem using a single expression. Let's dive into it step by step:
Step 1: Using the **
Operator
The **
operator is commonly used for dictionary unpacking. In this case, we can leverage it to combine the dictionaries x
and y
. Here's the code:
z = {**x, **y}
That's it! š By using the **
operator with the dictionaries x
and y
, we are able to merge them into the new dictionary z
.
Step 2: Overwrite Duplicate Keys
By using this one-liner expression, Python automatically handles the duplicate keys conflict resolution for us. When merging the dictionaries, the duplicate keys are overwritten with the values from the second dictionary. Exactly what we wanted! š
Step 3: Enjoy the Merged Dictionary!
Now, you can simply use the z
dictionary in your code. Feel free to access its keys and values as you would with any other dictionary. Enjoy the merged data! š
š„ Practice Makes Perfect: Example Usage
Let's take a look at another example to solidify our understanding:
fruits = {'apple': 5, 'banana': 10, 'orange': 7}
new_fruits = {'banana': 3, 'kiwi': 5, 'pear': 2}
merged_fruits = {**fruits, **new_fruits}
print(merged_fruits)
# Output: {'apple': 5, 'banana': 3, 'orange': 7, 'kiwi': 5, 'pear': 2}
In this case, we have two dictionaries representing quantities of different fruits. By merging the dictionaries using the **
operator, we obtain a new dictionary where the duplicate key 'banana'
takes the value from the new_fruits
dictionary.
š¢ Engage with Us and Share Your Thoughts!
We hope you found this blog post helpful and that it saves you time in your Python programming adventures! Do you have any other cool Python tips and tricks to share? Let us know in the comments below!
š£ Don't forget to share this post with your friends and colleagues who might also benefit from this Python hack. Happy coding! šŖ
ā ļø Remember, when using the
**
operator, the order in which you merge dictionaries matters. If duplicate keys exist, the value from the dictionary appearing later in the expression will overwrite the earlier one.
š” Pro Tip: If you are using Python 3.9 or above, you can even merge multiple dictionaries using a single expression by chaining the
**
operator multiple times.
āļø That's all for now, folks! Stay tuned for more amazing Python tips and tricks in our upcoming blog posts. Happy coding! š