How do I measure elapsed time in Python?
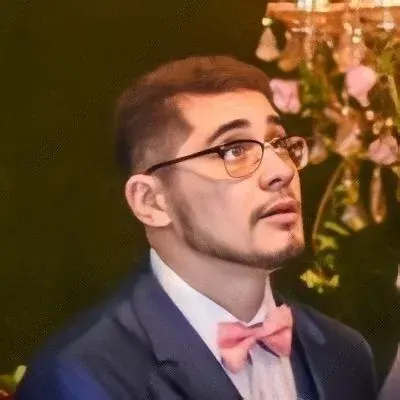
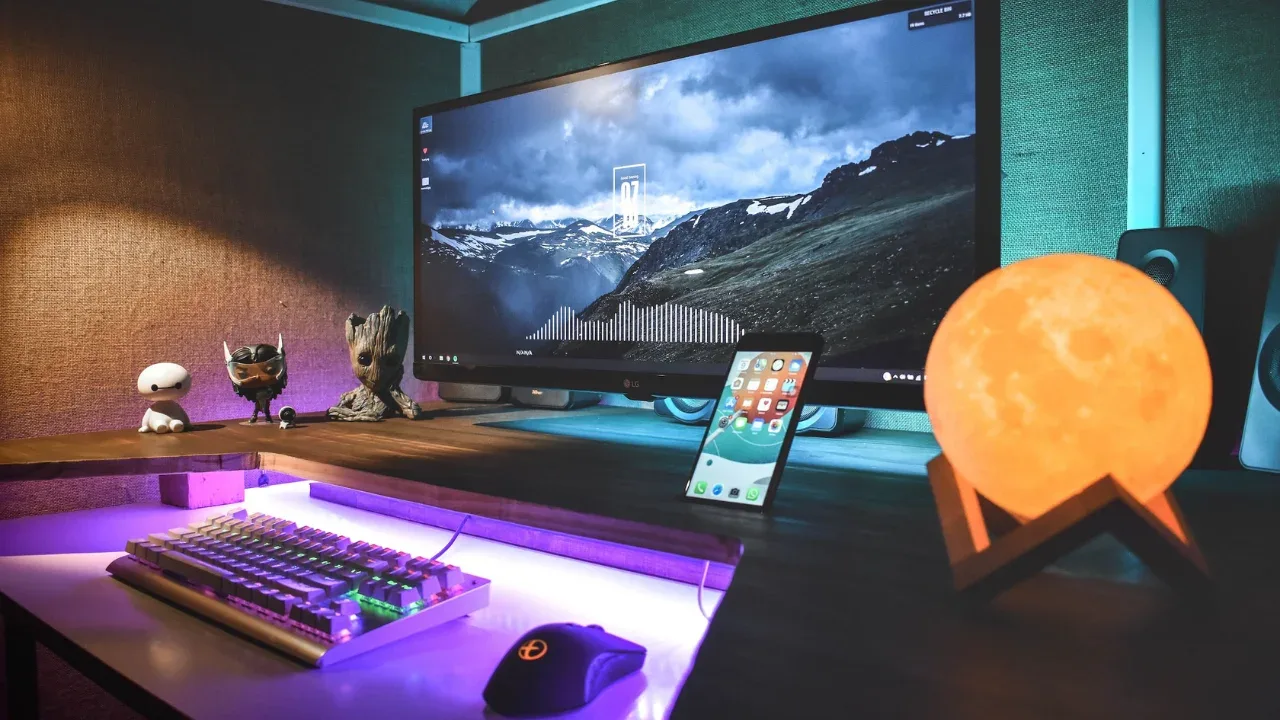
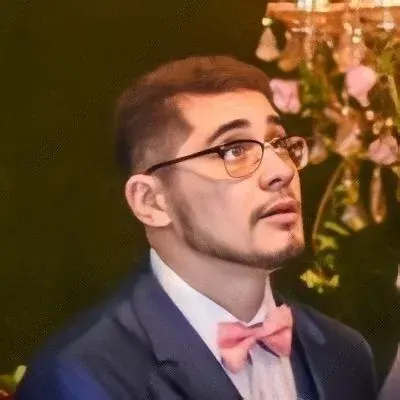
How to Measure Elapsed Time in Python? ⏱️
Are you tired of guessing how long your Python functions take to execute? Do you want an accurate way to measure elapsed time? Look no further! In this guide, we'll explore how to measure elapsed time in Python and tackle some common issues along the way. 🐍
The Trouble with timeit
🕒
One might naturally turn to the timeit
module in Python for timing functions. However, there seems to be a common misconception about how to use it correctly. Let's take a look at the code you provided as an example:
import timeit
start = timeit.timeit()
print("hello")
end = timeit.timeit()
print(end - start)
🤔 At first glance, you might expect this code to measure the time it takes to print "hello." But unfortunately, that's not the case. The reason is that timeit.timeit()
is not meant for measuring the time spent running a single statement.
A Simpler Solution ⭐
Luckily, Python offers a simpler alternative to measure elapsed time: the time
module. Let's check out an example:
import time
start = time.time()
print("hello")
end = time.time()
print(end - start)
🎉 Congratulations! You've successfully measured the elapsed time for the print statement. Here's how it works:
We import the
time
module, which provides functions for working with time-related tasks.We call
time.time()
to get the current time, which we store in thestart
variable.We execute the function or code we want to time.
We call
time.time()
again and store it in theend
variable.Finally, we calculate the elapsed time by subtracting the
start
time from theend
time.
💡 The resulting output will be the elapsed time in seconds.
Wrapping It Up 🎁
By transitioning from the timeit
module to the time
module, you now have a simple and effective way to measure elapsed time in Python. No more guessing or struggling with confusing syntax.
So the next time you have a function you want to time, remember to reach for the time
module. Measure the elapsed time with confidence and optimize your code like a pro! ⚡
If you found this guide helpful or have any additional insights, feel free to leave a comment below. Happy coding! 💻💬