How do I make a flat list out of a list of lists?
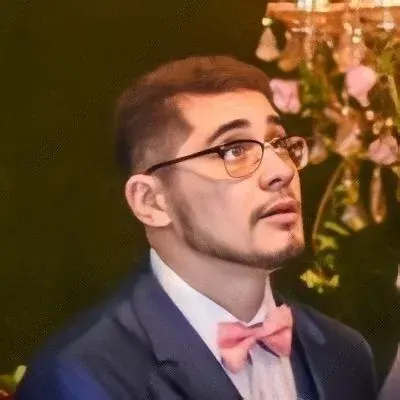
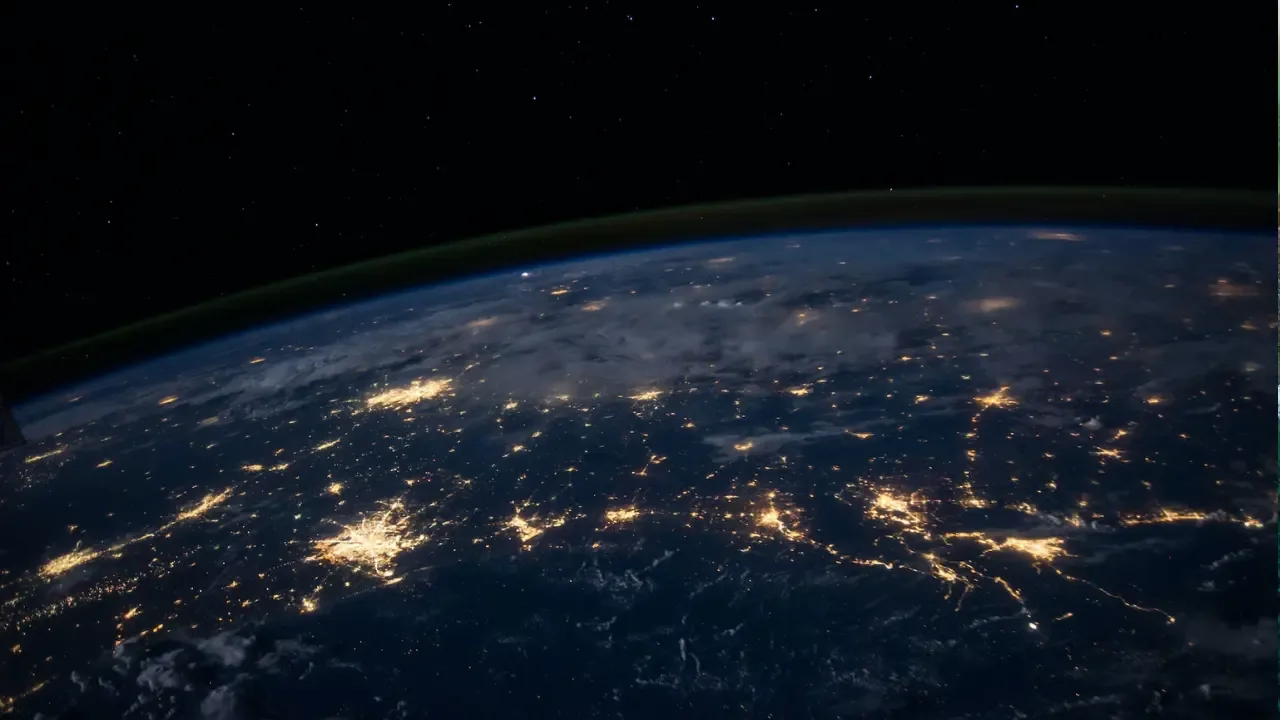
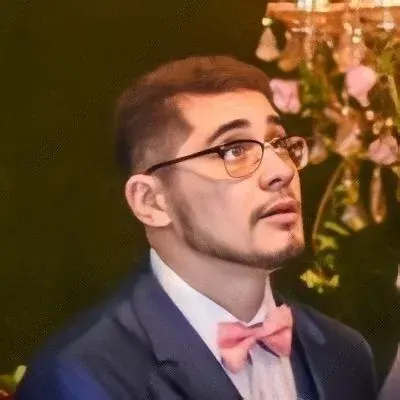
How to Flatten a List of Lists
Having a list of lists can be a bit tricky to work with, especially when you want to operate on the individual elements rather than the sublists. In this blog post, we will explore how to effectively flatten a list of lists, so you can easily access and manipulate the elements within it. 📚📝
The Problem: Flatten a List of Lists
Let's say you have a list of lists like this:
[
[1, 2, 3],
[4, 5, 6],
[7],
[8, 9]
]
And you want to obtain a single flat list like this:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
How can we achieve this? Let's dive in! 💪🔍
Solution 1: Using List Comprehension
One straightforward and Pythonic way to flatten a list of lists is by using a list comprehension. Here's an example:
nested_list = [
[1, 2, 3],
[4, 5, 6],
[7],
[8, 9]
]
flattened_list = [element for sublist in nested_list for element in sublist]
print(flattened_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
In the above code, we use two for
loops within the list comprehension. The outer loop iterates over each sublist, while the inner loop collects each element from the sublists. The result is a single flattened list. 🌟🐍
Solution 2: Using itertools.chain()
Another way to flatten a list of lists is by using the itertools.chain()
function. This function takes multiple iterables as arguments and returns a single iterator that combines the elements from all the iterables. Here's an example:
import itertools
nested_list = [
[1, 2, 3],
[4, 5, 6],
[7],
[8, 9]
]
flattened_list = list(itertools.chain(*nested_list))
print(flattened_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
In this example, we pass all the sublists as arguments to itertools.chain()
using the splat operator (*
). Then, we convert the returned iterator into a list using the list()
function. 🌈🔗
Take It Further
Now that you know how to flatten a list of lists, you can apply this knowledge to solve various programming challenges. For instance, you can use it to merge multiple datasets, process nested JSON objects, or simplify complex data structures. The possibilities are endless! 💡💪
Conclusion
Flattening a list of lists is a common task in Python programming. By using either list comprehension or itertools.chain()
, you can easily convert a nested structure into a flat list. So go ahead and apply these techniques in your projects to make your code more efficient and straightforward! 🚀💻
Have you ever encountered a situation where flattening a list of lists proved to be useful? Share your experience in the comments below! Let's learn from each other and keep the conversation going. 😄✨
References: