How do I lowercase a string in Python?
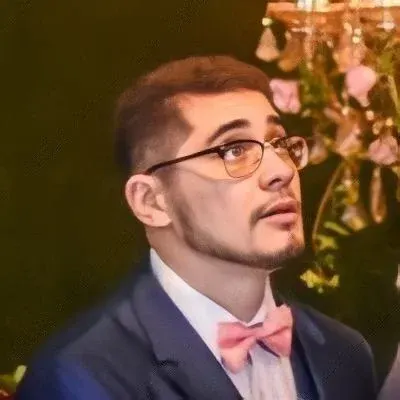
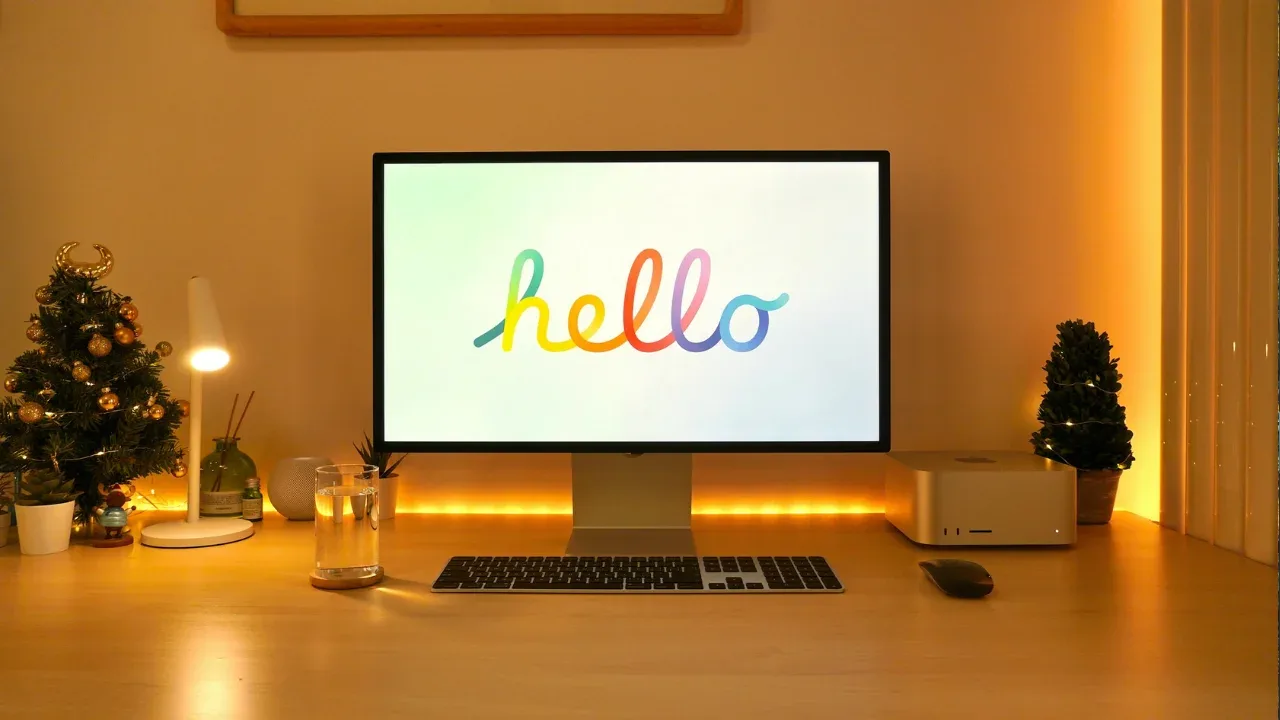
How to Lowercase a String in Python? ππ»π
Are you tired of dealing with strings in uppercase and need a quick way to convert them to lowercase? Look no further! In this guide, we'll explore different methods to achieve this in Python. Whether you're a beginner or an experienced developer, you'll find the solution that suits you best. Let's dive in!
The Problem π
So, you have a string like "Kilometers"
, and you want the same string in lowercase, like "kilometers"
. How can you achieve this?
The Solution(s) π‘π
1. The lower()
Method π§ͺβ
Python provides a handy built-in method called lower()
that does exactly what you need. It returns a new string with all the characters converted to lowercase. Let's see it in action:
string = "Kilometers"
lowercase_string = string.lower()
print(lowercase_string) # Output: kilometers
The lower()
method converts each uppercase character to its lowercase equivalent, leaving any lowercase characters unchanged. It's that simple! π
2. Using the str.casefold()
Method ππ‘
If you're working with Unicode characters, the casefold()
method is a safer option. It not only converts uppercase characters to lowercase ones but also handles special characters and non-English alphabets more reliably. Here's an example:
string = "ΔΓΆΔΓΌΕ"
lowercase_string = string.casefold()
print(lowercase_string) # Output: ΔΓΆΔΓΌΕ
The casefold()
method is more robust than lower()
and guarantees consistent results across different languages and character sets.
3. ASCII Lowercasing with str.lower()
πΊπΈπ’
In some cases, you might only be working with ASCII characters, like letters from the English alphabet. If that's your situation, you can use the str.lower()
method along with the string.ascii_lowercase
constant from the string
module like this:
import string
string = "HELLO WORLD"
lowercase_string = string.translate(str.maketrans(string.ascii_uppercase, string.ascii_lowercase))
print(lowercase_string) # Output: hello world
Here, we create a translation table using the str.maketrans()
method and then use str.translate()
to apply the table to the original string, converting all uppercase characters to their lowercase equivalents. π€π
Conclusion and Call-to-Action πππͺ
Converting a string to lowercase in Python doesn't have to be a headache! π€βοΈ We explored three different methods: the lower()
method for general usage, the casefold()
method for handling Unicode characters, and the combination of str.lower()
and str.translate()
if you're dealing with ASCII characters.
Now it's your turn to give it a try! Experiment with different strings and see how these methods work for you. If you have any questions or other cool string manipulation tricks to share, let us know in the comments below! Happy coding! ππ¨βπ»π©βπ»
<hr />
See How to change a string into uppercase? for the opposite.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
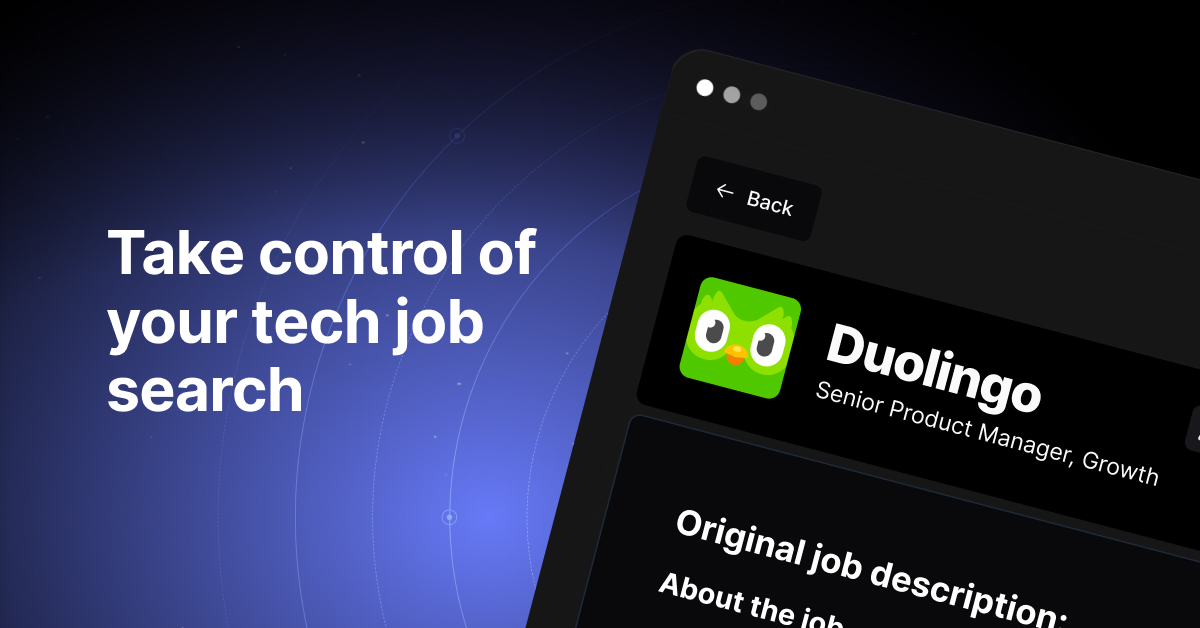