How do I list all files of a directory?
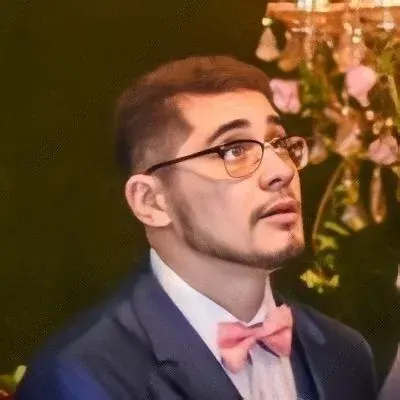
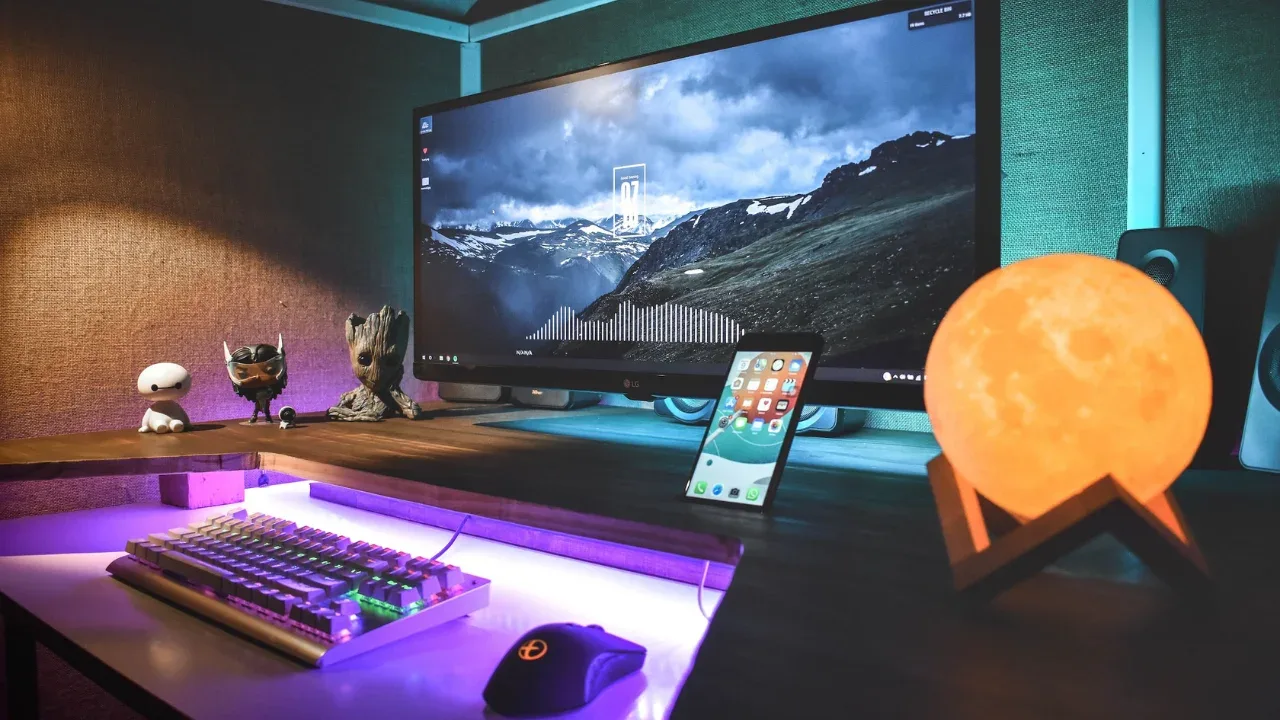
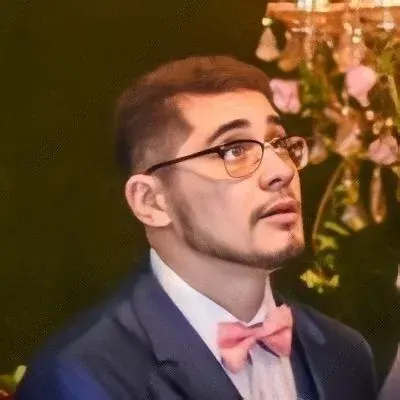
πTech Blog Post: The Ultimate Guide to Listing All Files in a Directory
πAre you a Python enthusiast trying to navigate the world of directories and files? πβ¨ Look no further! We have the solution to your problem. In this blog post, we will dive into the excellent way to list all files of a directory using Python and add them to a list
. ππ₯οΈ
π€ Why do you need to list all files in a directory? Listing all files in a directory is a common task in any programming project that deals with file manipulation. It allows you to gather information about the files in a given directory, iterate over them, analyze file names, or perform actions on specific file types.
π‘Approach 1: Using the os
module
The first approach we'll explore is using Python's built-in os
module. This module provides a range of functions that allow us to interact with the underlying operating system. To list all files in a directory, we'll utilize the os.listdir()
function.
Here's an example of how to use it:
import os
def list_files(directory):
files = os.listdir(directory)
return files
In the above example, we imported the os
module and defined a function called list_files
that takes a directory
parameter. The os.listdir()
function returns a list of all files and directories in the specified directory. We simply return this list, containing all the file names.
πExample
Let's say we have a directory called documents
located at C:\Users\YourUsername\Documents
. To list all files in this directory, we can use the following code:
files = list_files('C:\\Users\\YourUsername\\Documents')
print(files)
When you run the code, you will see a list of all the files in the documents
directory printed in the console.
πEasy, isn't it? You can now effortlessly list all the files in a directory using Python!
π‘Approach 2: Using the glob
module
Another approach is to use the glob
module, which provides advanced pattern-matching functionality for file and directory searches. The glob.glob()
function will allow us to filter files based on specific criteria, such as file extensions.
Consider the following example:
import glob
def list_files(directory):
files = glob.glob(directory + '/*')
return files
In the above code snippet, we imported the glob
module and defined the list_files
function. By using the glob.glob()
function, we specify the directory path with "/*"
to obtain all files within that directory. This approach provides more flexibility when dealing with specific file types.
πExample
Let's say you want to list all the .txt
files in a directory called data
. You can use the following code:
files = list_files('data/*.txt')
print(files)
When running the code, you will get a list of all the .txt
files within the data
directory.
π’Call-to-Action: Share your experience!
Now that you have mastered the art of listing all files in a directory, it's time to put your skills to the test. Share your experience and let us know how this guide has helped you! If you have any questions or alternative solutions, we'd love to hear about them in the comments section below. Let's learn and grow together! ππ©βπ»
So, what are you waiting for? Start exploring the vast world of directories and files with confidence. Happy coding! π»π
Disclaimer: The code snippets provided in this blog post are to be used as a reference and may require modifications based on your specific use case.