How do I iterate through two lists in parallel?
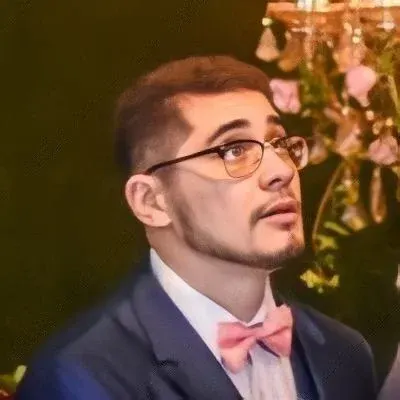
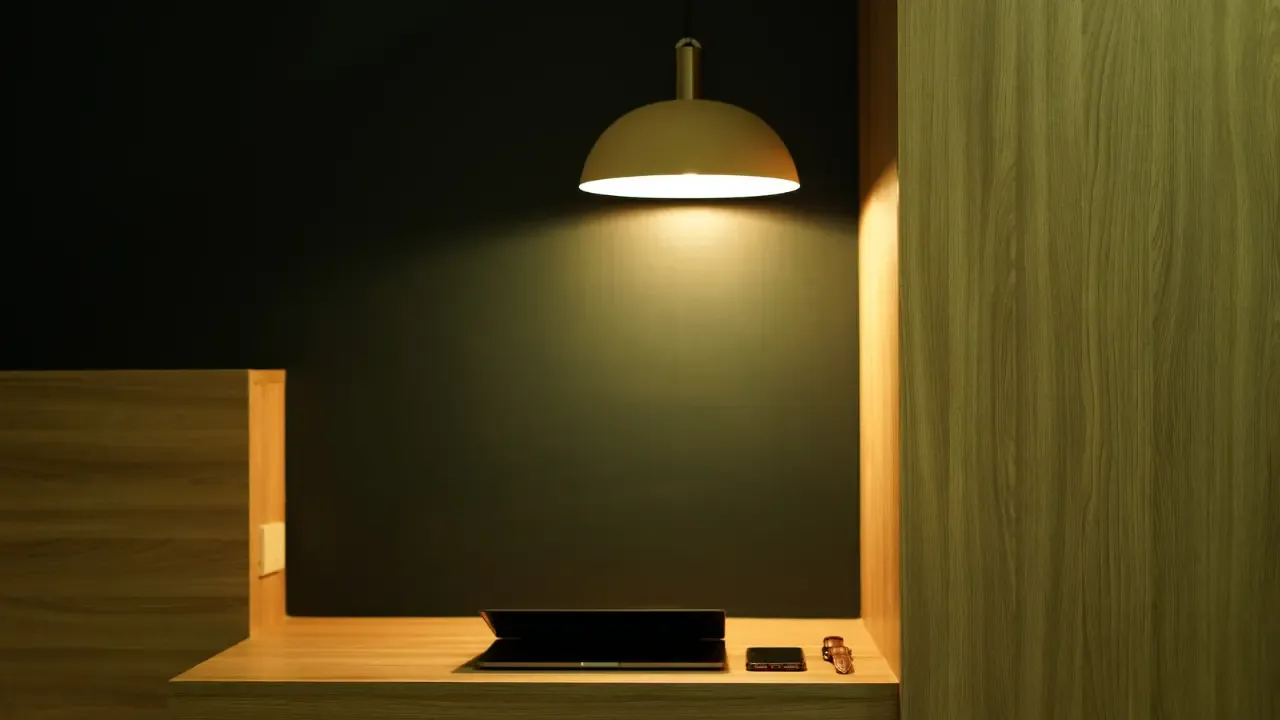
š Tech Blog: Iterating Through Two Lists in Parallel Made Easy!
š¤ Are you struggling to iterate through two lists at the same time and need a more efficient way? Look no further! š
š Let's begin by understanding the problem. You have two iterables, foo
and bar
, and you want to iterate over them together, accessing corresponding elements in pairs. For example:
foo = [1, 2, 3]
bar = [4, 5, 6]
for (f, b) in iterate_together(foo, bar):
print("f:", f, "| b:", b)
This should output:
f: 1 | b: 4
f: 2 | b: 5
f: 3 | b: 6
š The initial solution provided suggests using indices to access elements, but that can be cumbersome and not very Pythonic. Fortunately, there is a better way! š
š Solution: The zip()
Function š
The zip()
function allows you to iterate over multiple iterables simultaneously. It takes elements from each iterable and pairs them up, creating tuples. Let's see it in action:
for (f, b) in zip(foo, bar):
print("f:", f, "| b:", b)
The zip(foo, bar)
essentially combines the elements from foo
and bar
into pairs, which are then unpacked into (f, b)
. This simplifies the code and makes it more readable, Pythonic, and efficient!
šÆ Take it further: š
⨠Task 1: Merging Lists into a List of Tuples
If you want to merge foo
and bar
into a list of tuples, you can use the zip()
function with a list comprehension:
merged = [(f, b) for (f, b) in zip(foo, bar)]
print(merged)
Output:
[(1, 4), (2, 5), (3, 6)]
⨠Task 2: Creating a Dictionary from Separate Lists of Keys and Values
To create a dictionary from separate lists of keys and values:
keys = [1, 2, 3]
values = [4, 5, 6]
dictionary = dict(zip(keys, values))
print(dictionary)
Output:
{1: 4, 2: 5, 3: 6}
⨠Task 3: Constructing a Dictionary with Zip and Dict Comprehension
To construct a dictionary with zip()
and a dictionary comprehension:
keys = [1, 2, 3]
values = [4, 5, 6]
dictionary = {k: v for (k, v) in zip(keys, values)}
print(dictionary)
Output:
{1: 4, 2: 5, 3: 6}
š Congratulations! You now know how to iterate through two lists in parallel, merge lists into tuples, and create dictionaries effortlessly!
š We hope this guide helped you to overcome your iteration struggles! If you found this blog post helpful, don't forget to share it with your friends! And if you have any further questions or suggestions, feel free to leave a comment below. Happy coding! šš
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
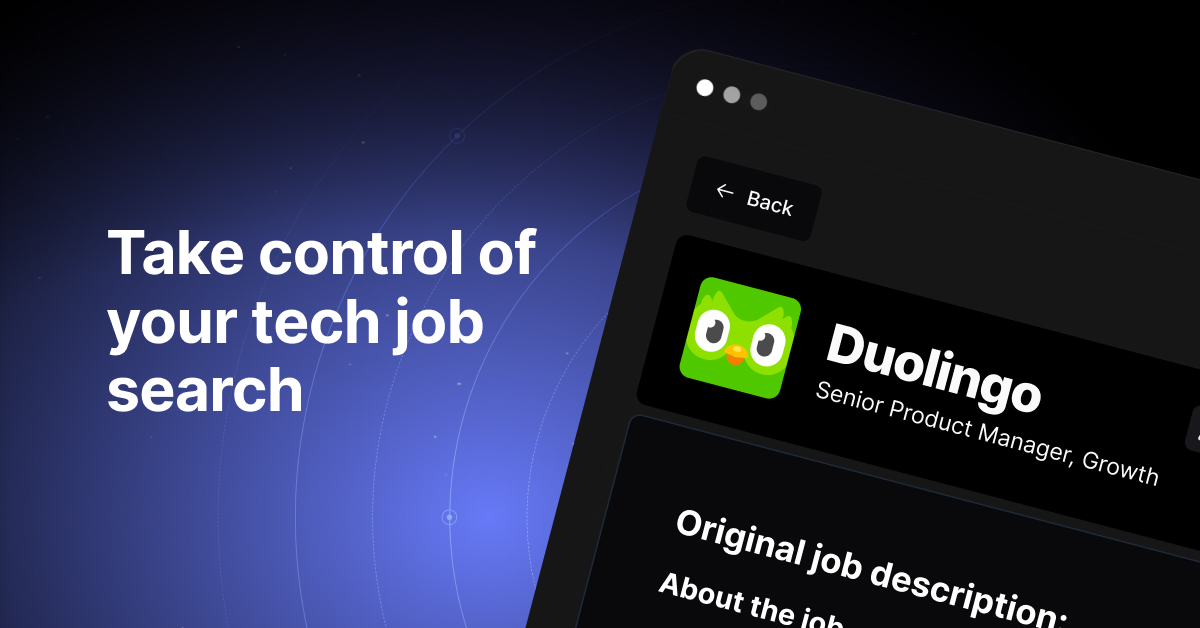