How do I integrate Ajax with Django applications?
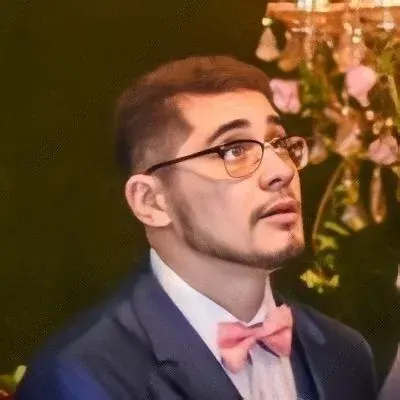
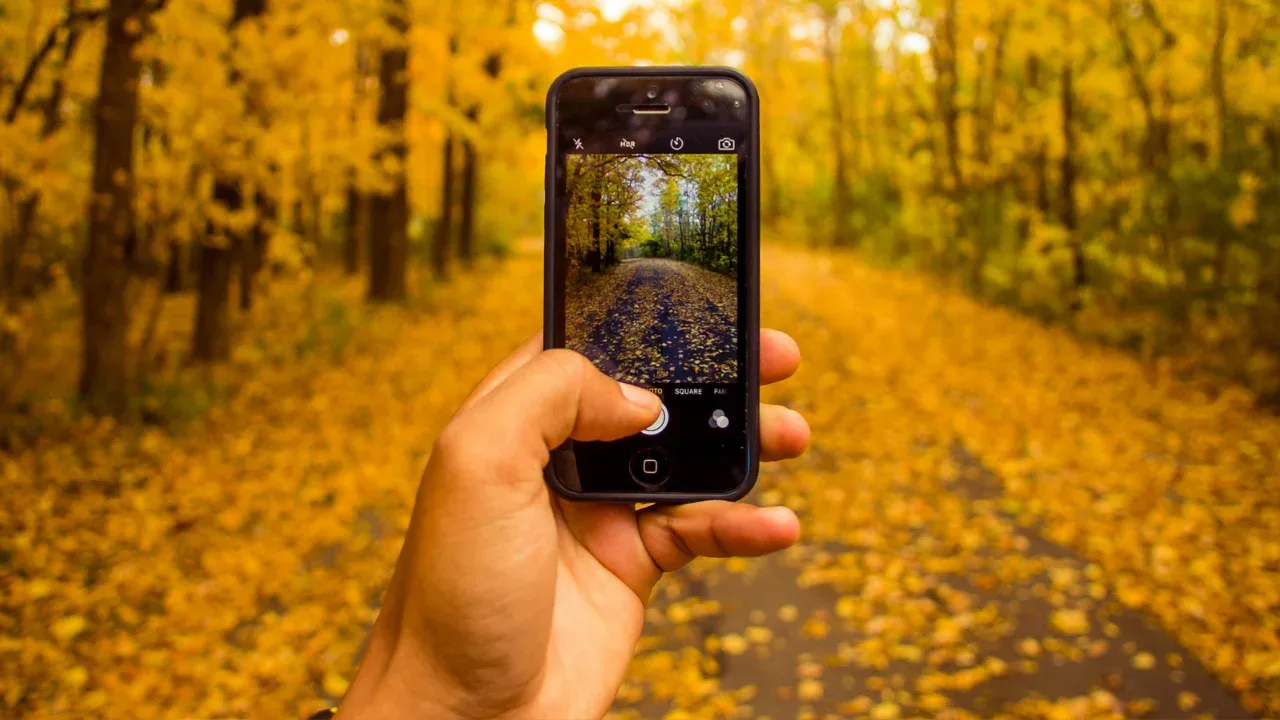
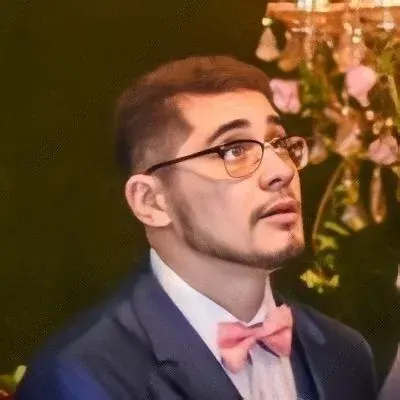
🌐 How to Integrate Ajax with Django Applications: A Beginner's Guide
Are you new to Django and Ajax, and struggling to understand how to integrate the two? Don't worry, we've got you covered! In this guide, we'll provide a quick explanation of how to combine Django and Ajax in your applications. We'll address common issues, provide easy solutions, and help you get started on the right track. Let's dive in! 💪
🤔 Understanding the Basics
Before we jump into integrating Ajax with Django, let's briefly understand what each of them is and how they work together.
Django: Django is a powerful and popular Python web framework that allows you to build web applications quickly and efficiently. It follows the Model-View-Controller (MVC) architecture, which helps in separating the different components of your application.
Ajax: Ajax stands for "Asynchronous JavaScript and XML", but don't let the jargon scare you! In simple terms, Ajax allows you to send and retrieve data from the server without refreshing the entire web page. It provides a seamless user experience by updating only specific parts of the page, resulting in faster and more interactive applications.
🔄 Integrating Ajax with Django
To integrate Ajax with your Django application, you need to follow a few steps:
Include the jQuery library: Ajax relies on jQuery, a popular JavaScript library, for making asynchronous requests. Make sure to include jQuery in your project by adding the following line within the
<head>
section of your HTML template:<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Including jQuery will allow you to use its powerful Ajax functions easily.
Write the Ajax code: Once you have jQuery included, you can write your Ajax code within a
<script>
section in your HTML template or in a separate JavaScript file. Here's a basic example that demonstrates how to send an Ajax request and handle the response:$.ajax({ url: "/your-ajax-endpoint-url/", type: "GET", dataType: "json", success: function(response) { // Handle the response data here }, error: function(xhr, status, error) { // Handle any errors here } });
In this example, we're using the
ajax
function provided by jQuery. You can customize the URL, request type (GET
,POST
, etc.), and specify the expected data type (json
,html
, etc.). The success and error functions handle the response accordingly.Implement the Django view: Now that we have the Ajax code in place, we need to implement the corresponding Django view that handles the Ajax request and sends back the response. Here's a simple example:
from django.http import JsonResponse def your_ajax_endpoint(request): # Process your logic and prepare the response data data = { "message": "Hello, Ajax!", "number": 42 } # Return the response as JSON return JsonResponse(data)
In this example, we're using Django's
JsonResponse
class to send the response data as JSON. You can customize the data based on your specific needs.Map the URL: Finally, you need to map the URL for your Ajax endpoint in Django's URL configuration. Open your
urls.py
file and add the following line:from django.urls import path from .views import your_ajax_endpoint urlpatterns = [ # Other URL patterns path('your-ajax-endpoint-url/', your_ajax_endpoint, name='your_ajax_endpoint'), ]
Replace
'your-ajax-endpoint-url/'
with the desired URL for your Ajax endpoint. This maps the URL to the corresponding view function we defined earlier.
🎉 You're All Set!
Congratulations! Now you know how to integrate Ajax with your Django applications. By following the steps outlined above, you'll be able to make asynchronous requests, handle responses, and create dynamic and interactive web applications.
Remember, this is just the tip of the iceberg when it comes to Django and Ajax. There are countless possibilities and features you can explore to enhance your applications further. So keep learning, experimenting, and building awesome things!
If you have any questions or face any difficulties along the way, don't hesitate to reach out to us. We're always here to help! 😊
Have you integrated Ajax and Django before? Share your experiences or any tips you have in the comments below! Let's learn and grow together. Happy coding! 🚀