how do I insert a column at a specific column index in pandas?
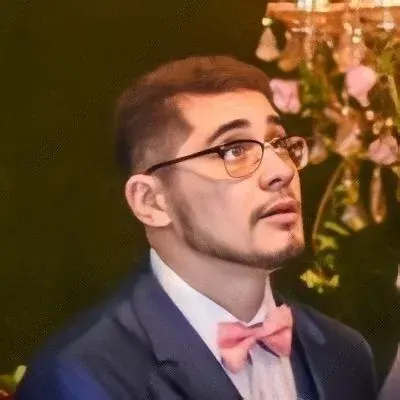
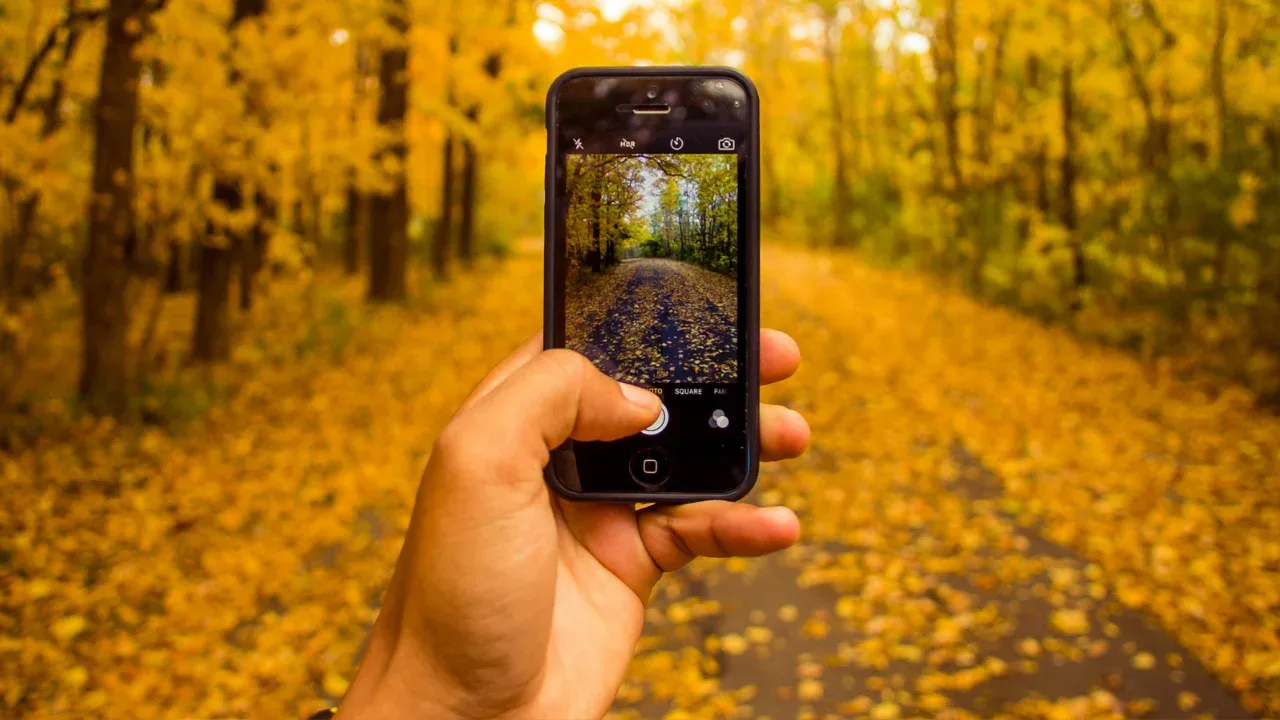
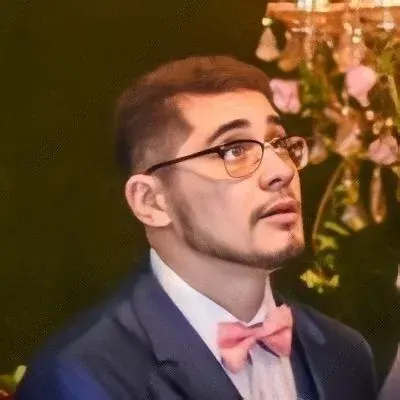
How to Insert a Column at a Specific Column Index in Pandas?
So, you're working with Pandas and you want to insert a column at a specific column index. You know how to append a column and make it the last one, but what if you want to place it at the beginning or any other position? Don't worry, I've got your back! 🤓
Let's start by addressing the common problem:
The Issue
By default, when you add a new column to a Pandas DataFrame, it gets appended to the end of the DataFrame. So, if you want to insert a column at a specific column index, you need to find an alternative solution.
The Solution
To insert a column at a specific column index, you can use the Pandas insert()
function. This function allows you to specify the index where the new column should be placed. Let's see how it works with an example:
import pandas as pd
df = pd.DataFrame({'l': ['a', 'b', 'c', 'd'], 'v': [1, 2, 1, 2]})
df.insert(0, 'n', 0)
In the example above, we have a DataFrame df
with columns 'l' and 'v'. We want to insert a new column 'n' at index 0, which means it will be placed as the first column of the DataFrame.
Explaining the Code
Let's break down the code to understand how it works:
We import the Pandas library using the
import
statement.We create a DataFrame
df
with two columns 'l' and 'v'.We use the
insert()
function ondf
to insert a new column named 'n' at index 0.The first argument of the
insert()
function is the index where the new column should be placed, which in this case is 0.The second argument is the name of the new column, which is 'n'.
Finally, we specify the values for the new column. In this example, we set all values to 0.
Verifying the Result
To make sure the column has been inserted correctly, you can print the DataFrame using the print()
function:
print(df)
The output should be:
n l v
0 0 a 1
1 0 b 2
2 0 c 1
3 0 d 2
As you can see, the new column 'n' has been inserted at index 0 and placed as the first column of the DataFrame.
Conclusion and Call-to-action
Now you know how to insert a column at a specific column index in Pandas using the insert()
function. This can be very useful in scenarios where you need to control the order of your columns or insert a new column at a specific position.
I hope this guide was helpful to you! If you have any questions or other Pandas-related topics you'd like to learn about, feel free to leave a comment below. Happy data manipulation! 📊