How do I import other Python files?
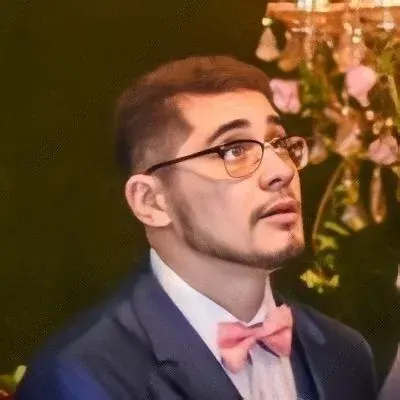
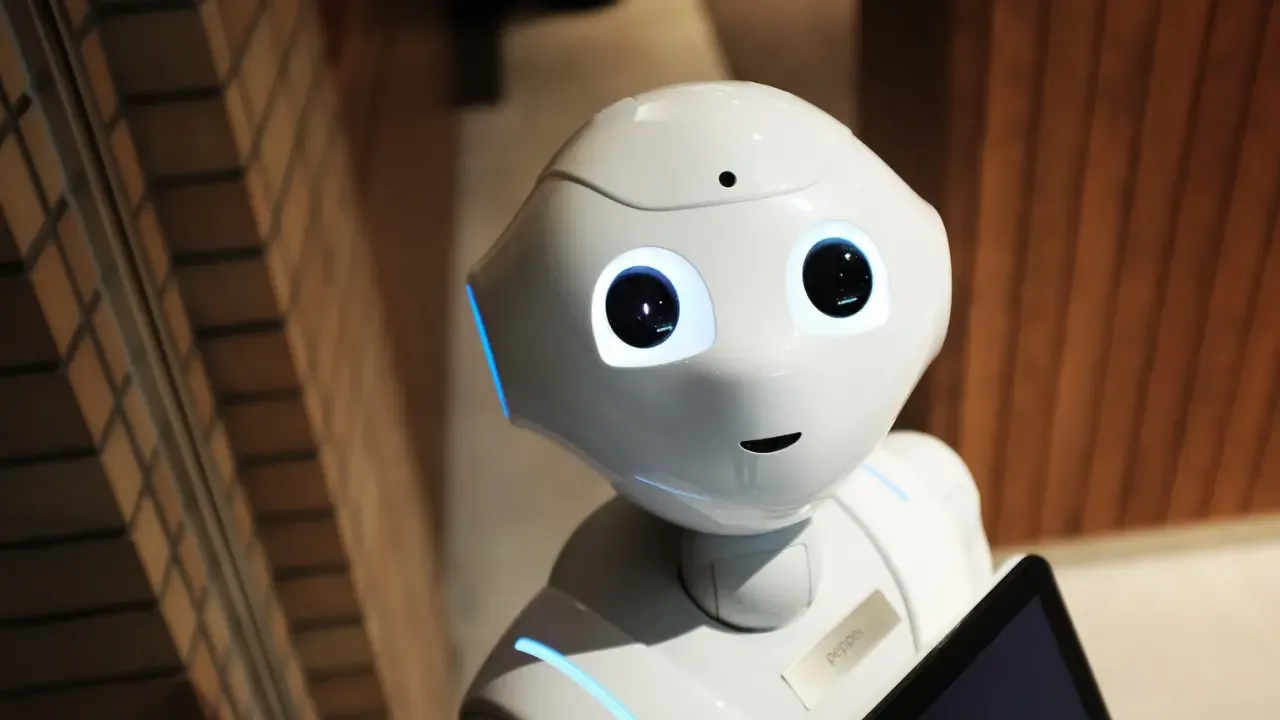
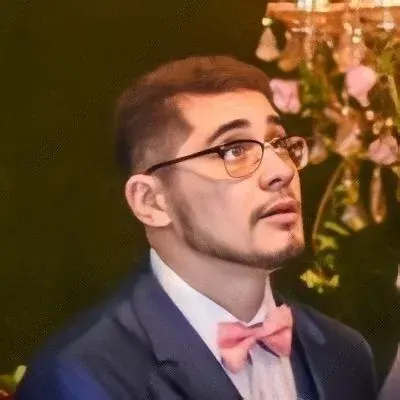
🐍 How to Import Other Python Files: A Complete Guide 📚
So, you want to import other Python files and are feeling a bit puzzled? Don't worry, you're not alone! Many Python beginners encounter this question and struggle with it. But fear not, because in this blog post, I'm going to break it down for you step by step! By the end, you'll be importing files like a pro. Let's get started! 💪
Importing a File
To import a file in Python, you simply use the import
statement followed by the name of the file without the .py
extension:
import file
So if your file is named file.py
, this line of code will import it. Easy peasy! 🎉
Importing a Folder
Sometimes, you may want to import an entire folder of Python files. This can be achieved by creating a special file called __init__.py
inside the folder. Once you've done that, you can import the entire folder using the folder's name:
import folder
Keep in mind that the __init__.py
file can be empty, but it is required for Python to treat the folder as a package.
Importing a File Dynamically at Runtime
Now, let's level up our Python skills! 🚀 What if you want to import a file based on user input or a specific condition at runtime? Python has got you covered with the importlib
module. Here's how you can do it:
import importlib
file_name = input("Enter the name of the file to import: ")
imported_module = importlib.import_module(file_name)
In this example, we ask the user to input the name of the file they want to import, and importlib.import_module()
does the magic for us.
Importing a Specific Part of a File
Imagine this scenario: you have a Python file with multiple functions or classes, but you only want to import and use a specific function. Python provides a way to do this using the from
statement. Here's an example:
from file import specific_function
This line of code imports only the specific_function
from the file.py
module. Now you can use that function directly in your code without having to reference the module name.
Wrapping Up and Taking Action
Congratulations! 🎉🎉 You've now learned the ins and outs of importing other Python files. No more struggling, you're in control! 😉 Feel free to experiment and apply what you've learned in your own projects.
Now it's your turn to take action! Try importing different files, folders, or specific parts of files. Play around and have fun with it! And don't forget to share your experience and any cool findings you come across in the comments below. Let's learn and grow together as Pythonistas! 💪🐍
👉✍️ Share your thoughts and experiences in the comments! Have you encountered any challenges while importing files? Did this guide help you? Let's have a chat and help each other out!
Remember, importing files is an important concept in Python, so it's crucial to understand it well. As always, keep exploring and stay curious. Happy coding! 🙌