How do I get user IP address in Django?
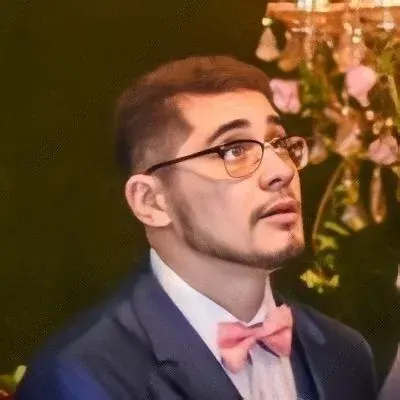
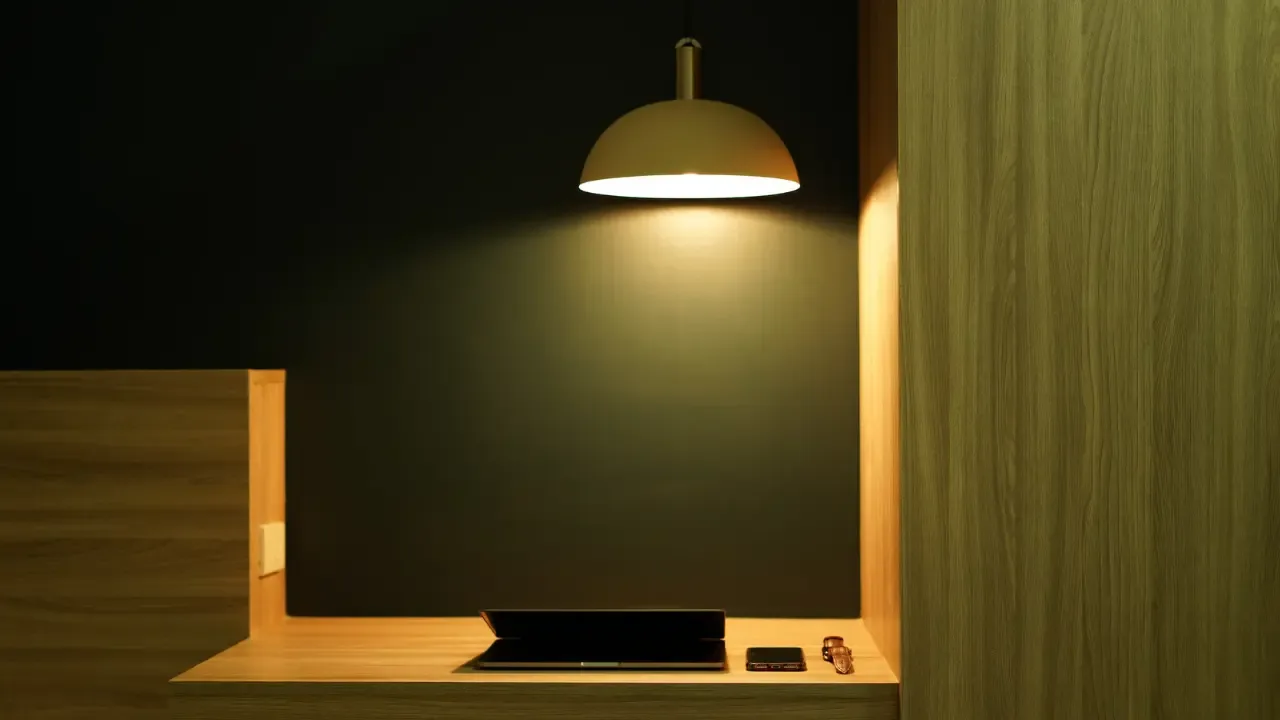
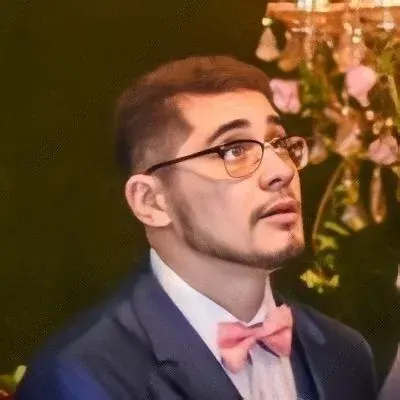
How to Get User IP Address in Django?
So you want to know how to get the user's IP address in Django? It seems like a simple task, but sometimes it can be a bit tricky. Don't worry, I've got you covered! In this blog post, I'll guide you through common issues and provide easy solutions to help you get the user's IP address in Django.
The Error and its Meaning
Before we delve into the solution, let's understand the error message you encountered. Based on the error message, it seems that the 'REMOTE_ADDR'
key is missing from the request.META
dictionary. This error usually occurs when the web server you are using doesn't provide the 'REMOTE_ADDR'
key by default. But fret not, we have other ways to obtain the user's IP address!
Alternative Methods to Get User IP Address
Method 1: Using request.META.get('HTTP_X_FORWARDED_FOR', '')
The request.META.get('HTTP_X_FORWARDED_FOR', '')
method can be used as a fallback solution when 'REMOTE_ADDR'
is not available. This method retrieves the IP address from the 'HTTP_X_FORWARDED_FOR'
header, which is commonly set by proxy servers. Here's an example of how to use it:
def home(request):
client_ip = request.META.get('HTTP_X_FORWARDED_FOR', '')
# Rest of your code
Method 2: Using get_host()
The Django HttpRequest
object provides a get_host()
method, which can be used to get the user's IP address when 'REMOTE_ADDR'
is not present. Here's how you can use it:
def home(request):
client_ip = request.get_host().split(':')[0]
# Rest of your code
Handling Common Issues
Issue: IP Address Returns Multiple IP Addresses
In some cases, the IP address may contain multiple values. To handle this situation, you can split the IP address and extract the first value. Here's an example:
def home(request):
client_ip = request.META.get('HTTP_X_FORWARDED_FOR', '').split(',')[0].strip()
# Rest of your code
Issue: Getting the Real User IP Behind a Proxy
If you are using Django behind a proxy server, you may need to configure it to trust the X-Forwarded-For
header. This header contains the real IP address of the user when requests are made through a proxy. To enable this, add the following line to your Django settings:
USE_X_FORWARDED_HOST = True
This will ensure that Django retrieves the user's IP address correctly.
Get Ready to Obtain the User's IP Address!
Now that you have learned alternative methods and resolved common issues, it's time to implement the solution that best suits your needs. Remember, different setups may require different methods, so choose the one that works best for you.
Don't let the missing 'REMOTE_ADDR'
key discourage you! With the information provided in this blog post, you'll be well-equipped to retrieve the user's IP address in Django.
Did this guide help you solve your problem? Let me know in the comments below! If you have any other questions or need further assistance, feel free to reach out. Happy coding! 😊🚀