How do I get the row count of a Pandas DataFrame?
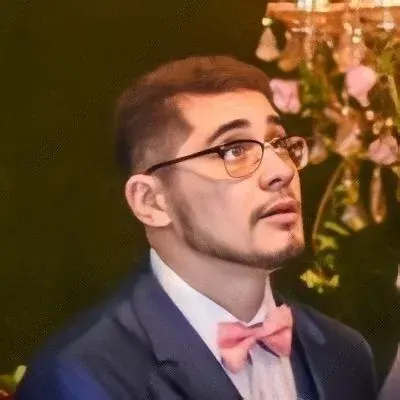
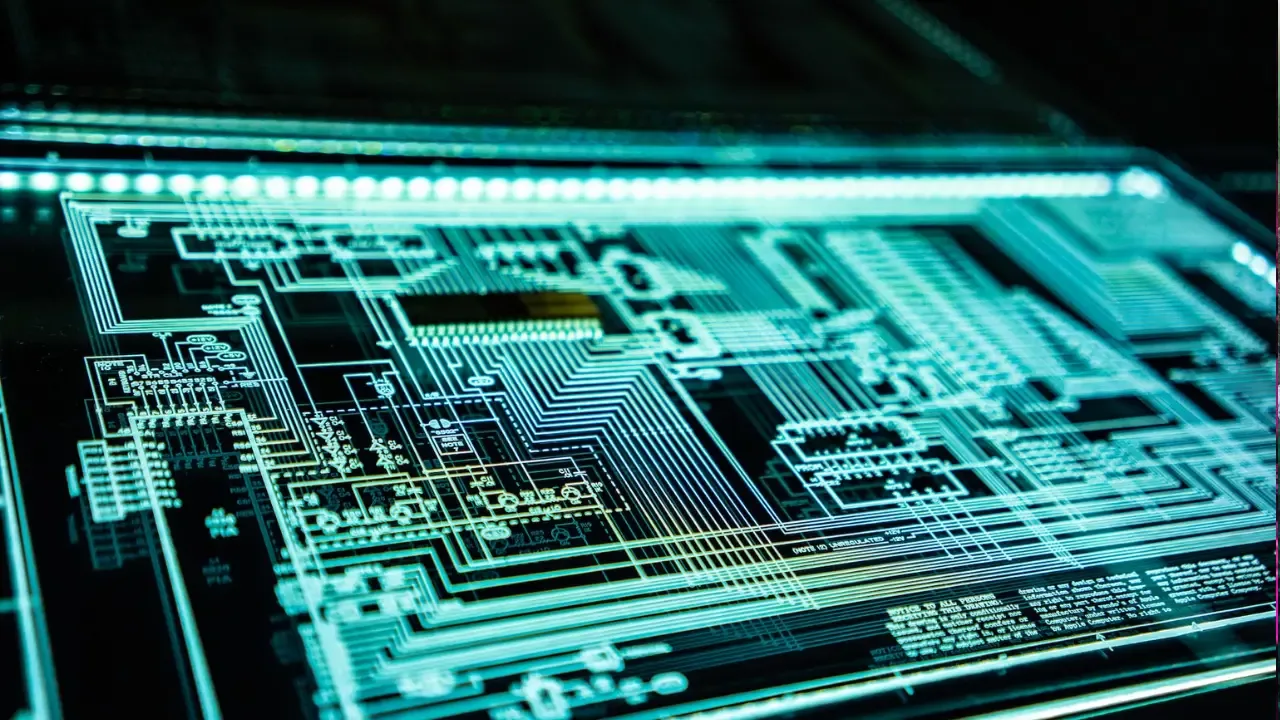
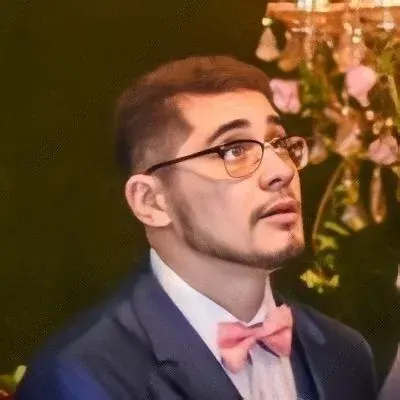
π Easy Guide to Getting Row Count of a Pandas DataFrame
So you have a Pandas DataFrame and you need to know how many rows it has, huh? Don't worry, you're not alone in this quest! π
The question here is: How do I get the row count of a Pandas DataFrame? π€ Don't worry, we'll walk you through it step by step. Let's dive in! πͺ
Problem:
You have a Pandas DataFrame called df
, and you want to know how many rows it contains.
Solution:
To get the row count of a Pandas DataFrame, you can use the shape
attribute or the len()
function. Let's explore both options.
Using the
shape
attribute:num_rows = df.shape[0]
The
shape
attribute returns a tuple with the dimensions of the DataFrame. The number of rows is represented by the first value in the tuple, which is accessed using[0]
.πBoom! You've got the row count! Easy, right? π
Using the
len()
function:num_rows = len(df)
The
len()
function returns the number of items in an object, and in the case of a Pandas DataFrame, it counts the number of rows.β¨VoilΓ ! Another way to get the row count with just one line of code! π
Example:
Let's say you have a DataFrame called sales_data
and you want to know how many rows it contains. Here's how you can do it:
import pandas as pd
# Creating a sample DataFrame
sales_data = pd.DataFrame({'Product': ['A', 'B', 'C', 'D'],
'Quantity': [10, 5, 14, 8],
'Price': [15.99, 9.99, 12.99, 7.99]})
# Getting the row count
num_rows = sales_data.shape[0]
print(f"The DataFrame has {num_rows} rows.")
Output:
The DataFrame has 4 rows.
Call-to-Action:
Now that you've learned how to effortlessly retrieve the row count of a Pandas DataFrame, why not apply this knowledge to your own data analysis projects? π Share with us how you plan to use this information or if you have any additional questions. We'd love to hear from you! ππ¬
Remember, understanding the basic properties of your DataFrame, such as the row count, will help you unlock incredible insights from your data. So go ahead, unleash the power of Pandas! πΌπͺ
Happy coding! π»β¨