How do I get the object if it exists, or None if it does not exist in Django?
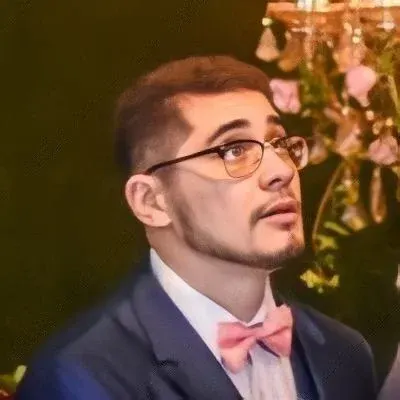
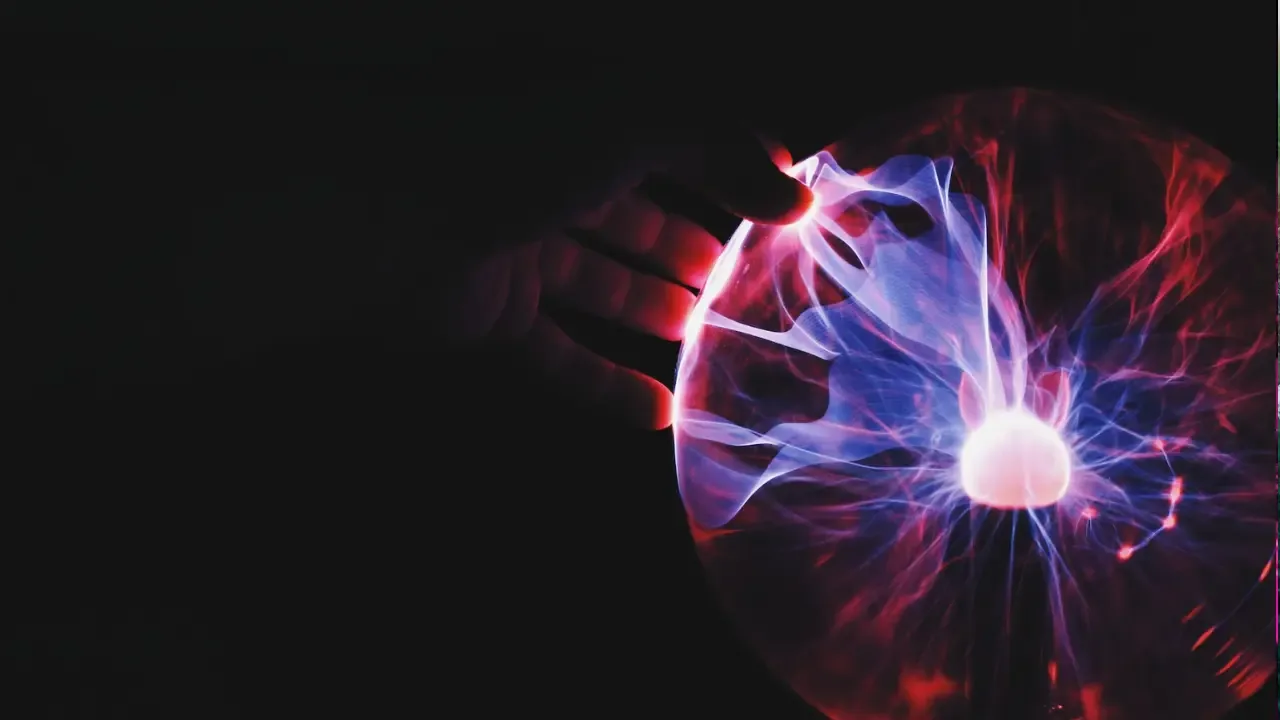
π Title: How to Get the Object You Want (or None) in Django!
Hey there, tech enthusiasts! Are you a Django developer struggling to handle model objects that may or may not exist? π€ Don't worry, we've got your back! In this blog post, we'll tackle a common issue faced by developers: getting an object if it exists, or None if it doesn't. Let's dive right in! π»π₯
The Dilemma: DoesNotExist or None?
So, you're using Django's model manager to retrieve an object, but if there's no match, it throws a pesky DoesNotExist
error. π« Fear not, we'll show you a way to gracefully handle this situation, allowing your variable to be set to None
instead.
go = Content.objects.get(name="baby")
Solution: Using the try-except
Block
To avoid the dreaded DoesNotExist
error, we can utilize a try-except
block. Here's how it works:
try:
go = Content.objects.get(name="baby")
except Content.DoesNotExist:
go = None
Let's break it down:
We enclose the
get()
method inside atry
block.If
get()
raises aDoesNotExist
error, the code inside theexcept
block will execute.Inside the
except
block, we assigngo
toNone
.
This clever solution ensures that your code remains elegant and avoids unnecessary disruptions. πβ¨
Putting it into Practice
Let's imagine you have a Django application that tracks cute animal videos. You want to retrieve a specific video by its name, but also want to handle the case when the video doesn't exist. Let's see how our solution can be implemented:
def get_video(name):
try:
video = Video.objects.get(name=name)
except Video.DoesNotExist:
video = None
return video
# Usage:
cute_video = get_video("baby panda")
if cute_video is None:
print("No video found!")
else:
print(f"Found a cute video: {cute_video}")
By encapsulating the object retrieval inside the try-except
block, you can safely handle situations where no matching object is found. πΌπΉ
Share Your Thoughts!
Now that you've learned how to gracefully handle the absence of objects in Django, why not share your experience with us in the comments section below? We'd love to hear about any challenges you've faced or alternative solutions you've discovered. Let's learn from each other! ππ¬
If you found this guide helpful, don't keep it to yourself! Share it with fellow Django developers who might be struggling with the same issue. Together, we can make coding easier and more enjoyable for everyone! Share the knowledge! π’π‘
Happy coding! π»π
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
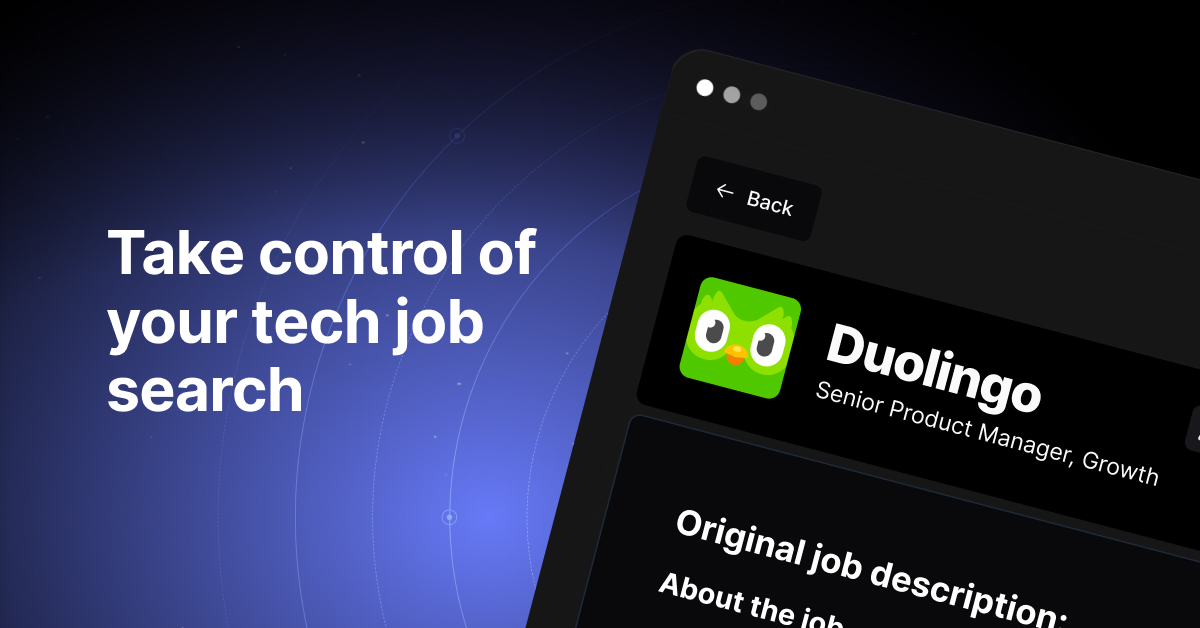