How do I get the full path of the current file"s directory?
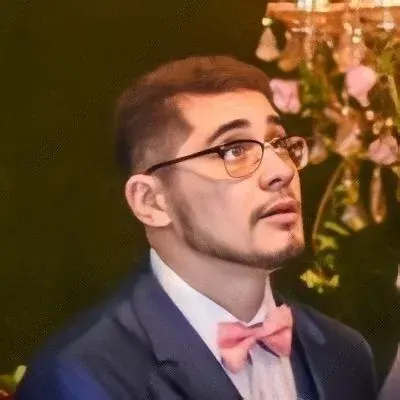
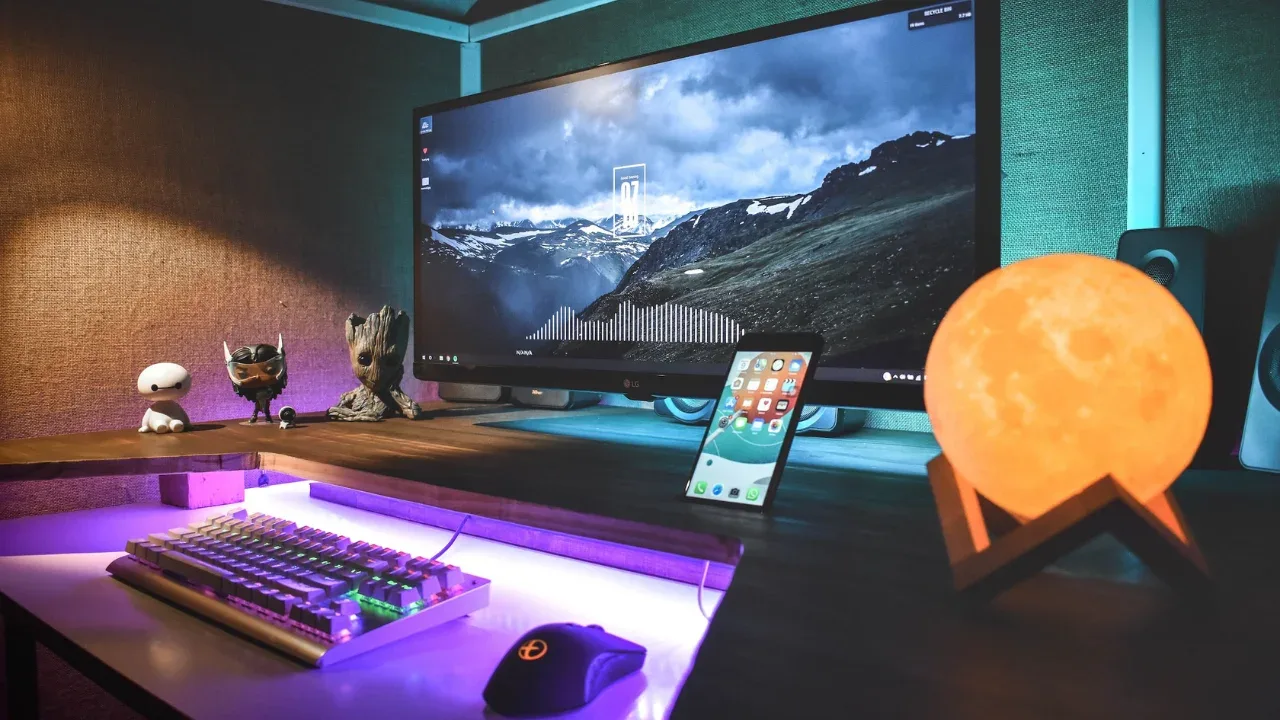
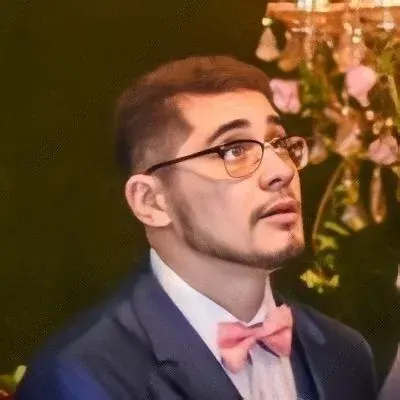
Getting the Full Path of the Current File's Directory 📂
Have you ever found yourself in a situation where you wanted to retrieve the full path of the directory containing your current file? 🤔 It may seem like a simple task, but it can be quite challenging if you don't know where to start. Don't worry, though, because we're here to help you out! 💪
In this guide, we'll address the common issues developers face when trying to obtain the full path of the current file's directory. We'll explore an easy solution using Python's os.path
module and provide you with a handy code snippet. So, let's dive right in! 🚀
The Problem: Getting the Current File's Absolute Path
The first approach that comes to mind is using the os.path.abspath(__file__)
method. Let's take a look at the code snippet you tried:
>>> os.path.abspath(__file__)
'C:\\python27\\test.py'
While this method returns the absolute file path, it doesn't give you the directory path as desired. You want 'C:\\python27\\'
, but the code snippet only provides 'C:\\python27\\test.py'
. So, how can we get the desired result? 🤷♀️
The Solution: Extracting the Directory Path 🗂
To obtain the directory path, we can utilize the os.path.dirname()
method. This method extracts the directory name from the given path. Let's modify the previous code snippet:
>>> os.path.dirname(os.path.abspath(__file__))
'C:\\python27'
By combining the os.path.abspath(__file__)
method with os.path.dirname()
, we get the desired result of 'C:\\python27\\'
. 🎉
Putting It All Together: A Handy Code Snippet 💡
To make your life easier, let's encapsulate the solution into a reusable code snippet. This way, you can simply copy and paste it whenever you need to retrieve the directory path.
import os
# Get the directory path
current_directory = os.path.dirname(os.path.abspath(__file__))
# Print the directory path
print(current_directory)
With this code snippet, you'll be able to obtain the full path of the current file's directory whenever you need it. No more scratching your head trying to figure it out! 😄
Take It a Step Further: Enhancing Your Workflow ✨
Now that you have the solution to your original question, why stop there? You can further enhance your development workflow using the obtained directory path. Here are a few ideas:
File Manipulation: Use the directory path to perform operations on files within the same directory, such as reading or writing files.
Module Importing: If you're working with modules or packages located in the same directory, you can add the directory path to
sys.path
and import them easily.Relative Path Resolution: Combine the directory path with a relative path to access files or directories in a structured manner.
Your Turn: Share Your Thoughts! 💬
Now that you know how to get the full path of the current file's directory and have some ideas on how to leverage it, we want to hear from you! Have you ever struggled with this problem? How did you solve it? Share your thoughts, experiences, and any additional tips or tricks in the comments below. Let's learn from each other! 👇
Happy coding! 💻