How do I get a list of all the duplicate items using pandas in python?
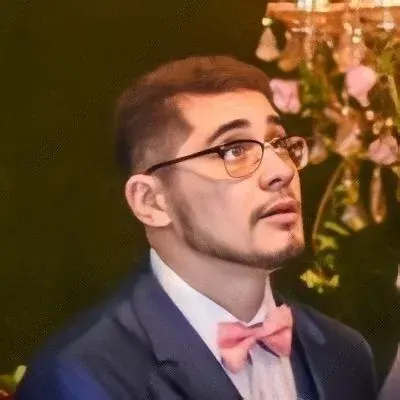
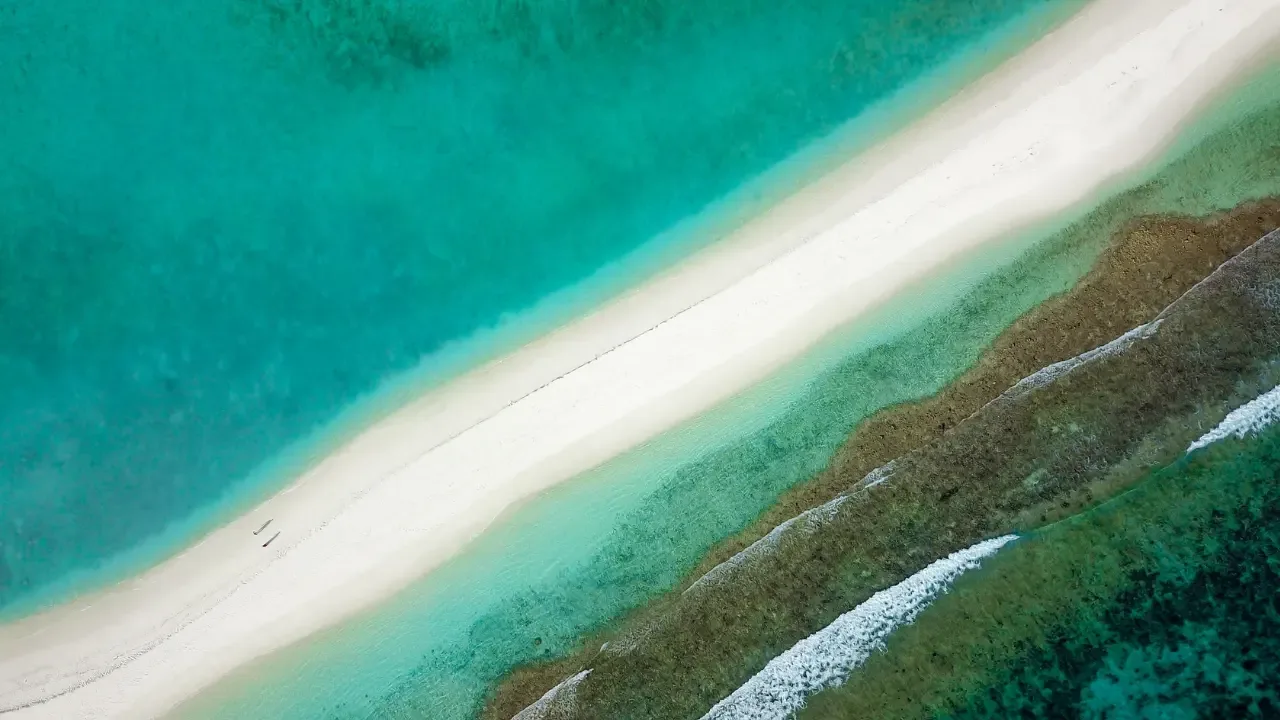
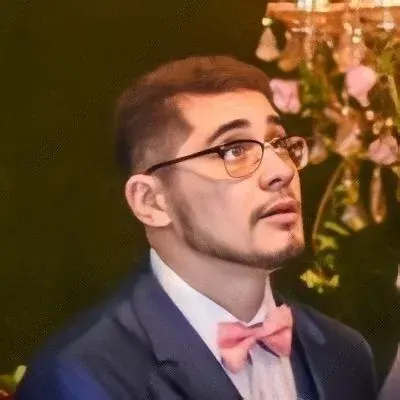
How to Get a List of All Duplicate Items Using Pandas in Python
Do you have a list of items and suspect there are some duplicates? Do you need to compare these duplicates manually? If you're using pandas in Python, you might have come across the duplicated
method, which only returns the first duplicate item. But what if you want to get all the duplicates and not just the first one?
In this guide, we'll explore how to use pandas in Python to get a list of all duplicate items. We'll address the common issue of the duplicated
method returning only the first duplicate and provide a easy solution to obtain all the duplicates. So, let's dive in!
The Problem
Let's start by understanding the problem. We have a dataset with multiple columns, and we want to find all the duplicate items in a specific column. For example, let's say we have a dataset that looks like this:
ID,ENROLLMENT_DATE,TRAINER_MANAGING,TRAINER_OPERATOR,FIRST_VISIT_DATE
1536D,12-Feb-12,"06DA1B3-Lebanon NH",,15-Feb-12
F15D,18-May-12,"06405B2-Lebanon NH",,25-Jul-12
8096,8-Aug-12,"0643D38-Hanover NH","0643D38-Hanover NH",25-Jun-12
A036,1-Apr-12,"06CB8CF-Hanover NH","06CB8CF-Hanover NH",9-Aug-12
8944,19-Feb-12,"06D26AD-Hanover NH",,4-Feb-12
1004E,8-Jun-12,"06388B2-Lebanon NH",,24-Dec-11
11795,3-Jul-12,"0649597-White River VT","0649597-White River VT",30-Mar-12
30D7,11-Nov-12,"06D95A3-Hanover NH","06D95A3-Hanover NH",30-Nov-11
3AE2,21-Feb-12,"06405B2-Lebanon NH",,26-Oct-12
B0FE,17-Feb-12,"06D1B9D-Hartland VT",,16-Feb-12
127A1,11-Dec-11,"064456E-Hanover NH","064456E-Hanover NH",11-Nov-12
161FF,20-Feb-12,"0643D38-Hanover NH","0643D38-Hanover NH",3-Jul-12
A036,30-Nov-11,"063B208-Randolph VT","063B208-Randolph VT",
475B,25-Sep-12,"06D26AD-Hanover NH",,5-Nov-12
151A3,7-Mar-12,"06388B2-Lebanon NH",,16-Nov-12
CA62,3-Jan-12,,,
D31B,18-Dec-11,"06405B2-Lebanon NH",,9-Jan-12
20F5,8-Jul-12,"0669C50-Randolph VT",,3-Feb-12
8096,19-Dec-11,"0649597-White River VT","0649597-White River VT",9-Apr-12
14E48,1-Aug-12,"06D3206-Hanover NH",,
177F8,20-Aug-12,"063B208-Randolph VT","063B208-Randolph VT",5-May-12
553E,11-Oct-12,"06D95A3-Hanover NH","06D95A3-Hanover NH",8-Mar-12
12D5F,18-Jul-12,"0649597-White River VT","0649597-White River VT",2-Nov-12
C6DC,13-Apr-12,"06388B2-Lebanon NH",,
11795,27-Feb-12,"0643D38-Hanover NH","0643D38-Hanover NH",19-Jun-12
17B43,11-Aug-12,,,22-Oct-12
A036,11-Aug-12,"06D3206-Hanover NH",,19-Jun-12
Our goal is to identify all the duplicate entries in the ID
column.
The Existing Code
To solve this problem, the user originally tried using the following code:
df_bigdata_duplicates = df_bigdata[df_bigdata.duplicated(cols='ID')]
However, this code only returns the first duplicate item. In our dataset example, it would only return the first A036
entry and the first 11795
entry.
The Solution
To obtain all the duplicate items in the ID
column, we can use the duplicated
method differently. Here's the updated code:
df_bigdata_duplicates = df_bigdata[df_bigdata.duplicated(subset='ID', keep=False)]
Let's break this down:
subset='ID'
: This specifies that we only want to consider theID
column for duplicates.keep=False
: By setting this parameter toFalse
, we keep all duplicate items instead of keeping the first occurrence and marking the rest as duplicates.
With this updated code, we will get all the duplicate entries for the ID
column, including the three A036
entries and the two 11795
entries in our dataset example.
Conclusion
Finding duplicate items in a dataset can be essential for data analysis and quality control. Pandas provides a simple yet powerful method, duplicated
, to identify duplicates. By understanding the parameters and options of this method, we can easily obtain all the duplicates instead of just the first duplicate item.
Next time you encounter a duplicate item problem while working with pandas in Python, remember to use the duplicated
method with the subset
and keep
parameters to get the complete list of duplicates.
I hope this guide was helpful in solving your duplicate item issue. If you have any questions or other pandas-related problems, feel free to leave a comment below. Let's harness the power of pandas together!
π Happy coding! πΌπ»
π£ Call to Action:
If you found this guide helpful, why not share it with your friends and colleagues who might be struggling with duplicate items in pandas? Spread the knowledge and help others overcome this common issue!
Don't forget to subscribe to our newsletter to receive more amazing pandas tips and tricks straight to your inbox. Stay up-to-date with the latest data science and programming trends and level up your skills.
Keep exploring, keep learning, and keep embracing the awesomeness of pandas! π©βπ»π¨βπ»π
Disclaimer: The dataset used in this example is fictional and for demonstration purposes only.