How do I format a string using a dictionary in python-3.x?
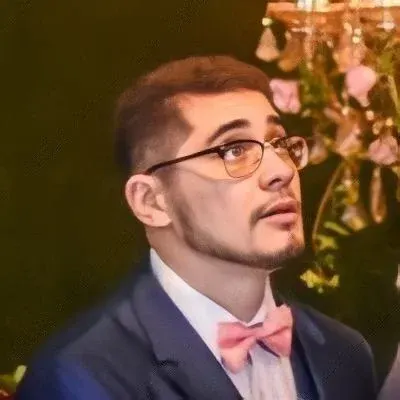

How to Format a String Using a Dictionary in Python 3.x
Are you a fan of using dictionaries to format strings in Python? It's a great way to improve readability and take advantage of existing dictionaries. However, if you've recently upgraded to Python 3.x, you might have noticed that the syntax for formatting strings with dictionaries has changed. But don't worry, we've got you covered! In this blog post, we'll explore the new syntax and provide you with easy solutions to format strings using dictionaries in Python 3.x.
The Old Syntax
In Python 2.x, you could format strings using dictionaries like this:
class MyClass:
def __init__(self):
self.title = 'Title'
a = MyClass()
print 'The title is %(title)s' % a.__dict__
path = '/path/to/a/file'
print 'You put your file here: %(path)s' % locals()
The output of this code would be:
The title is Title
You put your file here: /path/to/a/file
As you can see, we used the %
operator to format the string, and the dictionary values were inserted using the placeholder %(<key>)s
.
The New Syntax
In Python 3.x, the syntax for formatting strings with dictionaries has changed to use the str.format()
method. Let's take a look at the updated code:
geopoint = {'latitude': 41.123, 'longitude': 71.091}
print('{latitude} {longitude}'.format(**geopoint))
Notice that we used {<key>}
as the placeholder instead of %(<key>)s
. Additionally, we used the unpacking operator **
to pass the dictionary values to the format()
method.
The output of this code would be:
41.123 71.091
Awesome, our string is formatted correctly! But what if we don't have a dictionary and only have separate variables? No worries, Python 3.x has a solution for that too. Take a look at this code:
latitude = 41.123
longitude = 71.091
print('{latitude} {longitude}'.format(latitude=latitude, longitude=longitude))
The output would be the same as before:
41.123 71.091
As you can see, we assigned the values of latitude
and longitude
directly inside the format()
method using the key=value
pairs.
💡 Pro Tip: Using F-Strings
Starting from Python 3.6, there's an even cooler way to format strings using dictionaries or variables. It's called f-strings, and it provides a concise and readable way to format strings. Here's an example:
geopoint = {'latitude': 41.123, 'longitude': 71.091}
print(f"{geopoint['latitude']} {geopoint['longitude']}")
The output is still the same:
41.123 71.091
F-strings allow you to directly embed expressions inside curly braces, making your code more readable and compact.
Conclusion
Formatting strings using dictionaries in Python 3.x is slightly different from Python 2.x. By using the str.format()
method and the correct placeholder syntax, you can easily format strings with dictionaries or variables. And if you're using Python 3.6 or later, don't forget to check out f-strings for a more concise and readable formatting option.
So go ahead and give it a try! Upgrade your code to use the new syntax and experience the power of Python 3.x string formatting. And if you have any questions or other cool tips to share, feel free to leave a comment below.
Happy coding! 👩💻🔥
📚 Further Reading
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
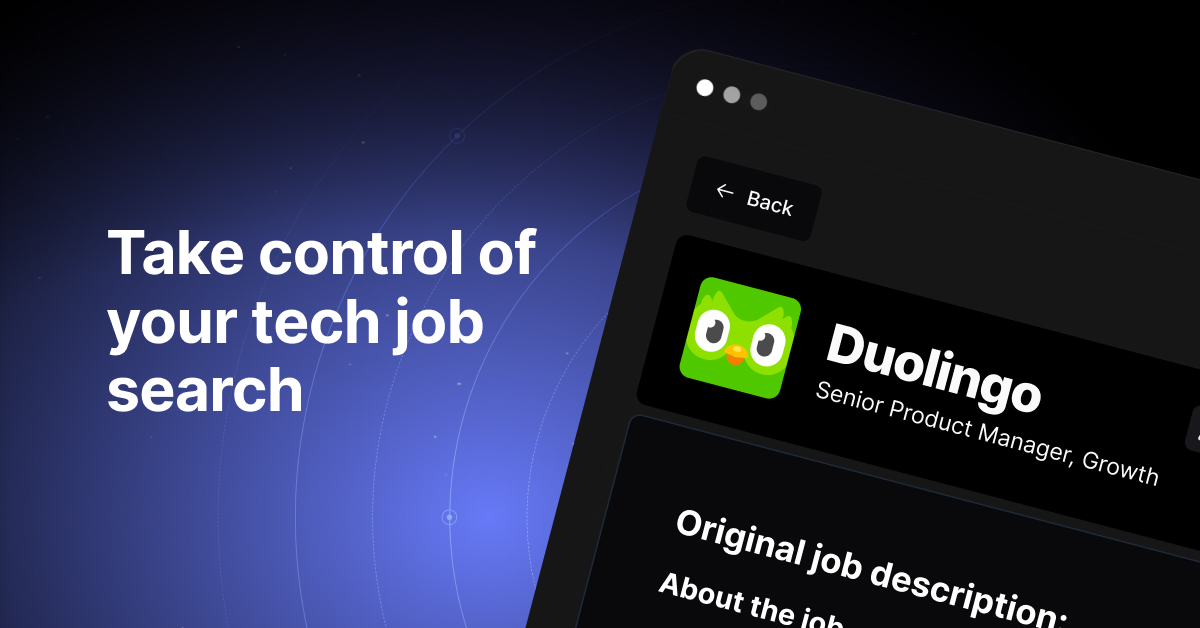