How do I filter query objects by date range in Django?
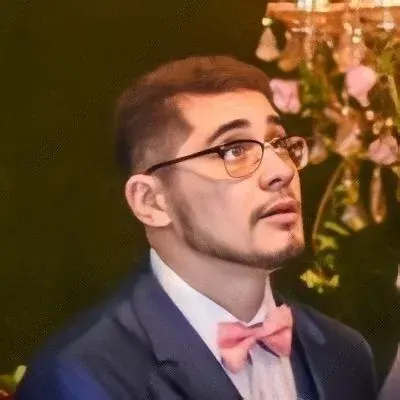
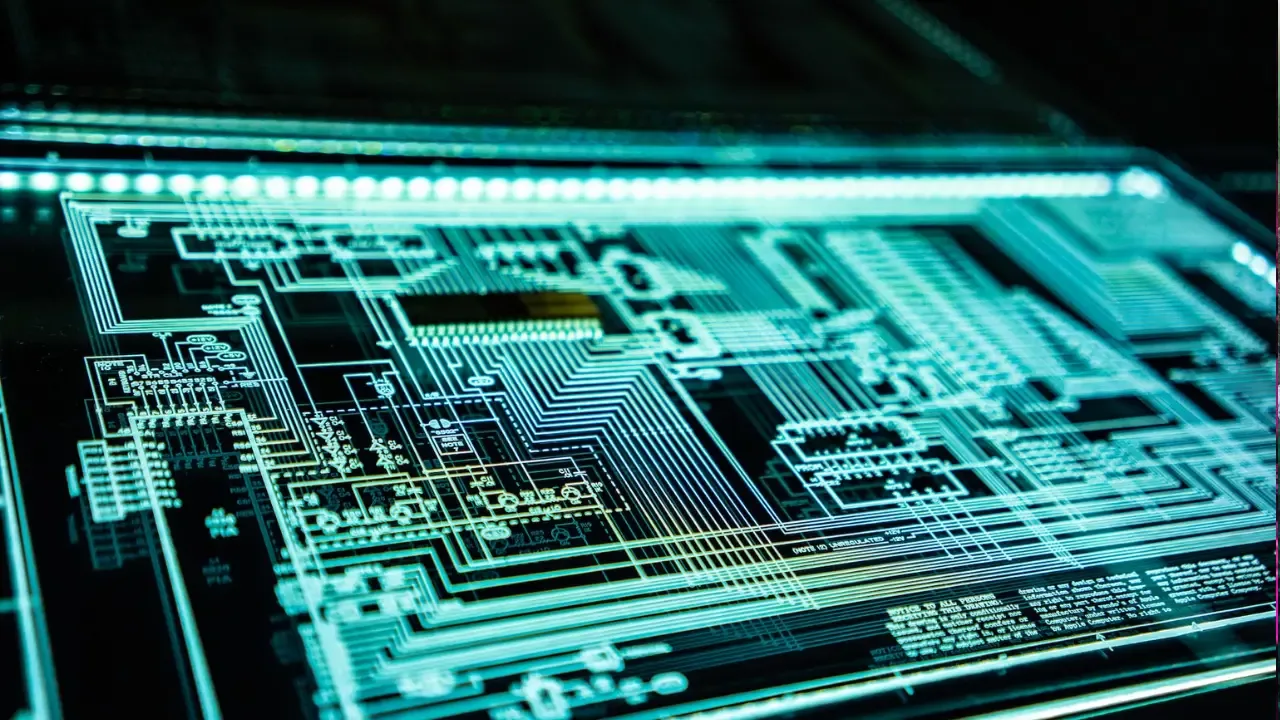
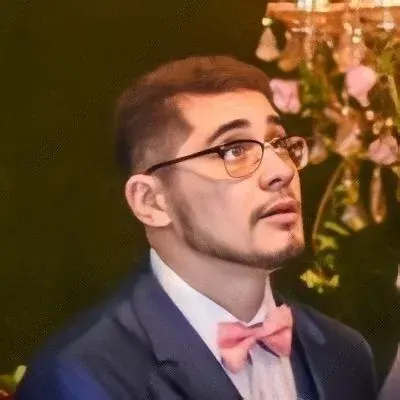
Filtering Query Objects by Date Range in Django: The Ultimate Guide
⨠Welcome to our tech blog ⨠Today, we're diving into the wonderful world of Django, where we'll explore how to filter query objects by date range. šš”
The Challenge: Filtering Objects by Date Range
Imagine you have a Django model called Sample
with a date
field, and you want to filter all the objects that fall within a specific date range. š
class Sample(models.Model):
date = fields.DateField(auto_now=False)
To solve this challenge, we'll explore a few easy-to-follow solutions. Let's get started! š
Solution 1: Using the __range
Lookup
The __range
lookup is a powerful Django feature that allows us to filter query objects within a specified date range. Here's how you can use it:
from django.db.models import Q
from datetime import date
start_date = date(2011, 1, 1)
end_date = date(2011, 1, 31)
filtered_objects = Sample.objects.filter(date__range=(start_date, end_date))
In this example, we import the necessary modules and define the start and end dates for our desired date range. Then, we use the __range
lookup with the filter()
method to retrieve all the Sample
objects that fall within that range. Easy-peasy, right? š
Solution 2: Using the gte
and lte
Lookups
Alternatively, you can accomplish the same result by using the __gte
(greater than or equal to) and __lte
(less than or equal to) lookups:
from datetime import date
start_date = date(2011, 1, 1)
end_date = date(2011, 1, 31)
filtered_objects = Sample.objects.filter(date__gte=start_date, date__lte=end_date)
These lookups allow you to filter objects where the date is greater than or equal to the start date and less than or equal to the end date. Super simple, right? š
Solution 3: Combining Multiple Conditions
Sometimes, you might need to filter objects using more complex conditions. In those cases, you can combine multiple Q objects using the |
(OR) operator or the &
(AND) operator. Here's an example:
from django.db.models import Q
from datetime import date
start_date = date(2011, 1, 1)
end_date = date(2011, 1, 31)
filtered_objects = Sample.objects.filter(Q(date__gte=start_date) & Q(date__lte=end_date))
In this case, the Q
object allows us to define two separate conditions: one for the start date and one for the end date. By combining them with the &
operator, we can filter objects that satisfy both conditions. Pretty neat, huh? š
Call-to-Action: Share Your Experience!
Congratulations! You've become a Django date range filtering expert. š
Now, we want to hear from you! How do you usually filter objects by date range in Django? Do you have any questions or other challenging scenarios? Leave a comment below, and let's start a conversation! šš¤
Remember to share this blog post with your fellow Django enthusiasts, so they can benefit from this handy guide, too. Happy coding! š»š