How do I filter ForeignKey choices in a Django ModelForm?
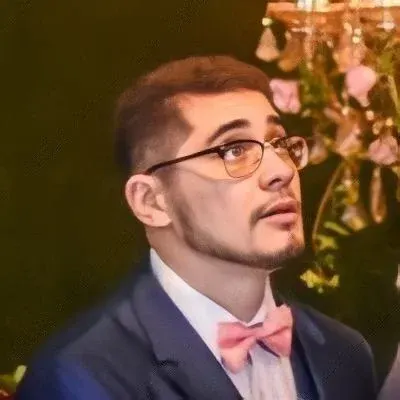
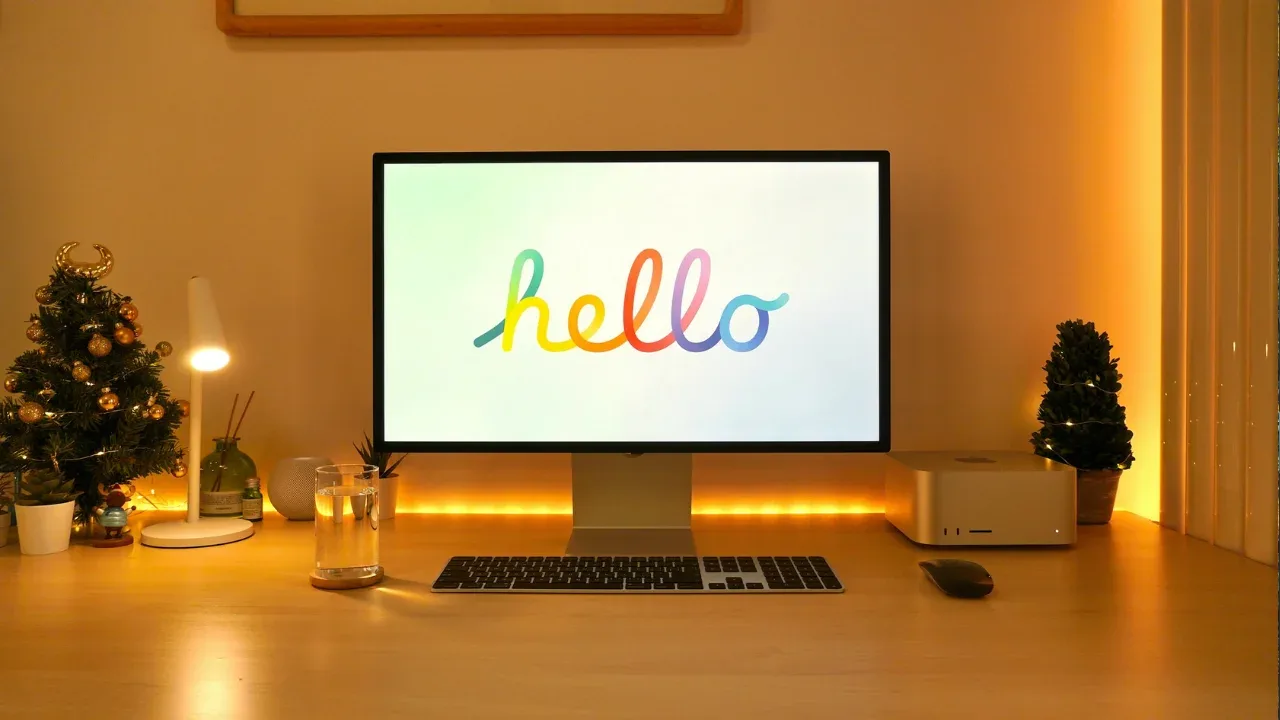
How to Filter ForeignKey Choices in a Django ModelForm 🧩
Are you struggling to filter the ForeignKey
choices in your Django ModelForm
? Don't worry, I've got you covered! In this guide, I'll walk you through common issues and provide easy solutions to help you limit the choices based on your needs. Let's dive in! 💻
The Scenario 📚
Let's start by understanding the context of your question. You have three models in your models.py
file: Company
, Rate
, and Client
. Each Client
belongs to a specific Company
and should have a base Rate
chosen from the parent Company's Rates
, not from another Company's Rates
. The goal is to create a form for adding a Client
that removes the Company
choices and limits the Rate
choices to that specific Company
.
The Initial Setup 🏗️
Your forms.py
and views.py
files are currently basic and do not include the required logic for filtering the choices. Let's modify them step by step to achieve the desired functionality.
In your forms.py
file, update the ClientForm
class as follows:
from models import *
from django.forms import ModelForm
class ClientForm(ModelForm):
class Meta:
model = Client
exclude = ['company'] # Remove the 'company' field from the form
Here, we exclude the company
field to remove it from the form since it has already been selected via the "Add Client" button on the Company
page.
Next, let's update your views.py
file. In the addclient
function, add logic to filter the Rate
choices based on the selected Company
. Here's the modified code:
from django.shortcuts import render_to_response, get_object_or_404
from models import *
from forms import *
def addclient(request, company_id):
the_company = get_object_or_404(Company, id=company_id)
if request.POST:
form = ClientForm(request.POST)
if form.is_valid():
client = form.save(commit=False)
client.company = the_company
client.save()
return HttpResponseRedirect(the_company.get_clients_url())
else:
form = ClientForm()
# Filter the Rate choices based on the selected Company
form.fields['base_rate'].queryset = Rate.objects.filter(company=the_company)
return render_to_response('addclient.html', {'form': form, 'the_company': the_company})
In this code, we set the queryset
of the base_rate
field in the form to filter the Rate
choices based on the selected Company
. Now, you're ready to go! 🎉
The Result 🌟
With the modifications made to your code, the Company
choices will be removed from the form, and the Rate
choices will be filtered based on the selected Company
. You have successfully tackled the challenge of filtering ForeignKey
choices in your Django ModelForm
.
Further Improvements 🚀
You've achieved your goal with the current setup, but there are always opportunities to improve code readability and maintainability. Here are a couple of suggestions:
Consider using better naming conventions. Instead of
ClientForm
andaddclient
, you can useAddClientForm
andadd_client
, respectively. This enhances code clarity and follows Python's recommended naming style.Look into Django's Class-Based Views (CBVs). CBVs provide a more structured approach to handling views and can make your code more organized and reusable.
Feel free to explore these improvements and adapt them to your project's needs!
Conclusion 🏁
Filtering ForeignKey
choices in a Django ModelForm
is a common requirement, and with the steps outlined in this guide, you can easily achieve it. By excluding the company
field and filtering the Rate
choices based on the selected Company
, you can create a customized form that fits your needs. Happy coding! 😃
Do you have any other Django-related questions or challenges? Let me know in the comments below! Let's keep the conversation going! 🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
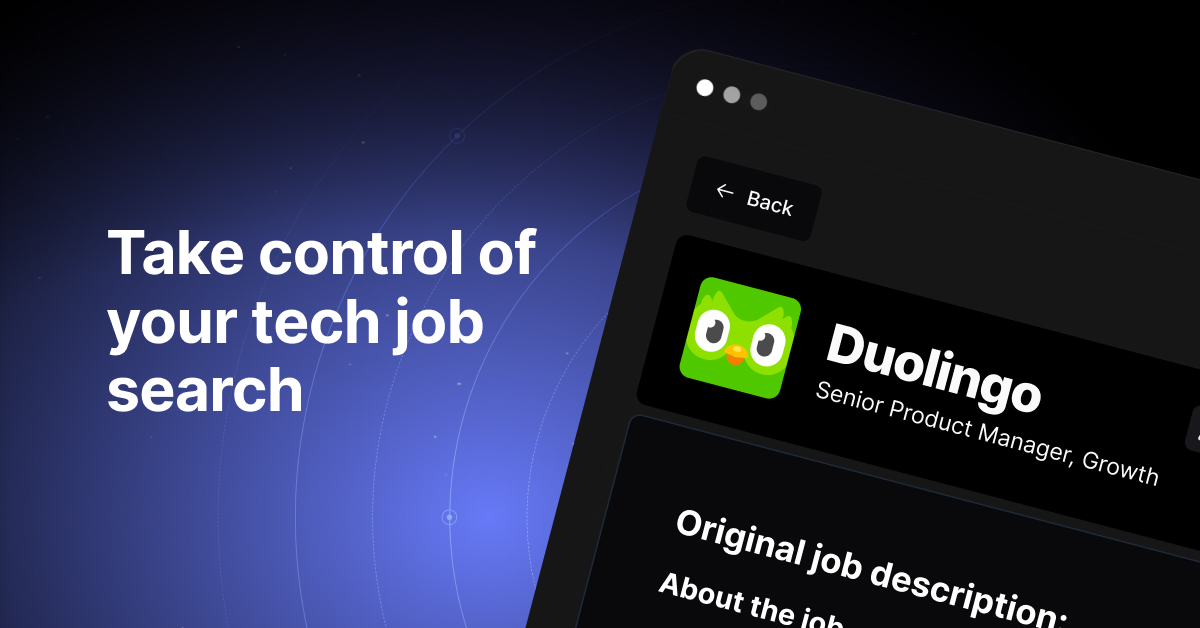