How do I execute a program or call a system command?
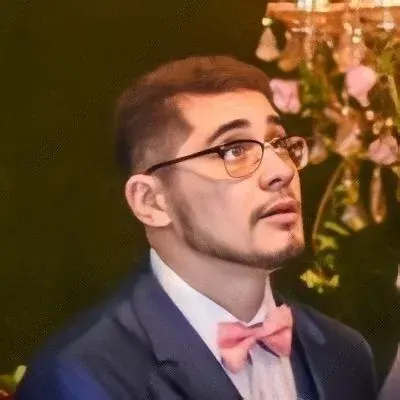
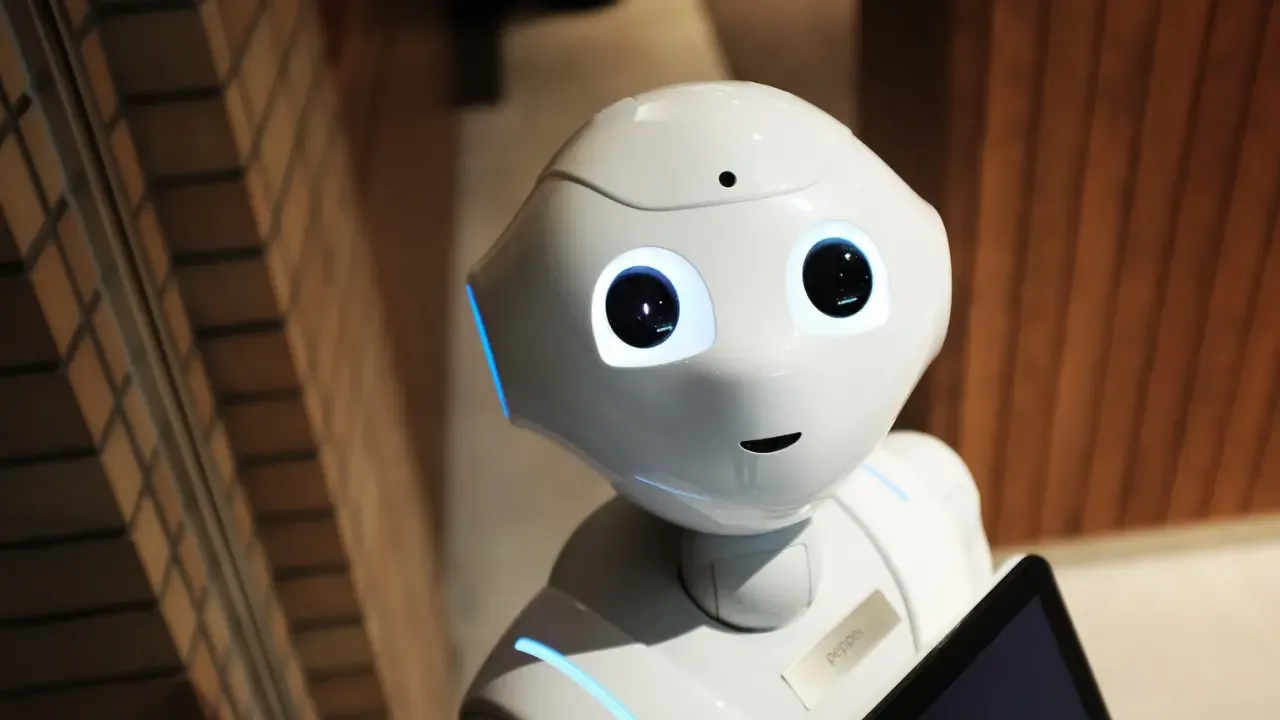
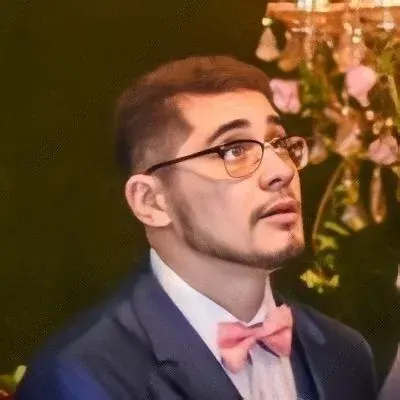
How to Execute a Program or Call a System Command with Python 🐍
So, you find yourself needing to execute a program or call a system command within your Python code. Whether you want to automate a task, interact with the operating system, or simply want to get things done more efficiently, Python provides us with some handy tools to achieve that. In this post, we'll explore different methods to execute programs or call system commands using Python 🚀.
What's the problem? 🤔
The question at hand is: "How do I call an external command within Python as if I had typed it in a shell or command prompt?" Well, you're in luck, friend! Python has got your back! 😎
Solution 1: Using the os
Module 🥳
Python's built-in os
module provides functions for interacting with the operating system. One of the methods it offers is os.system()
, which allows us to execute system commands from our Python script.
Here's a simple example that demonstrates how to execute a command using os.system()
:
import os
command = "echo Hello, World!"
os.system(command)
In this example, we're using the echo
command to print "Hello, World!" to the console. You can replace the command
variable with any system command you'd like to execute.
Solution 2: Using the subprocess
Module 🤓
While os.system()
is easy and convenient, the subprocess
module offers a more powerful and flexible way to execute programs or system commands from Python.
Here's an example showcasing how to use subprocess.run()
to execute a command:
import subprocess
command = "echo Hello, World!"
subprocess.run(command, shell=True)
The subprocess.run()
function provides more control over the execution, allowing us to capture the output, set environment variables, and even specify input data. Just remember to set shell=True
when using shell built-ins or shell syntax.
Common Issues and Tips 💡
Issue 1: Command not found
If you encounter a "Command not found" error when executing a command, it might be because the command is not in the system's PATH environment variable. To fix this, you can provide the full path of the command or add the directory containing the command to the PATH.
Issue 2: Capturing output
By default, both os.system()
and subprocess.run()
execute the command without capturing its output. If you want to capture the output, you can assign it to a variable like this:
import subprocess
command = "echo Hello, World!"
result = subprocess.run(command, shell=True, capture_output=True)
output = result.stdout.decode().strip()
print(output)
Issue 3: Handling command arguments
If your command requires arguments, you need to provide them as a list instead of a single string. For example:
import subprocess
command = ["ls", "-l", "-a"]
subprocess.run(command)
Conclusion and Call-to-Action 🙌
You're now equipped with the knowledge to execute programs or call system commands with Python. Whether you choose the simplicity of os.system()
or the power of subprocess.run()
, it's up to you and your specific requirements.
So, go ahead and automate those tasks, interact with the operating system, and unleash the full potential of Python! 💪
Hope you found this guide helpful! If you have any questions or want to share your experiences, feel free to leave a comment below. Happy coding! 😄🚀