How do I declare an array in Python?
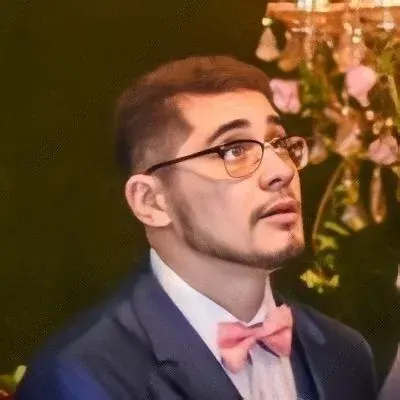
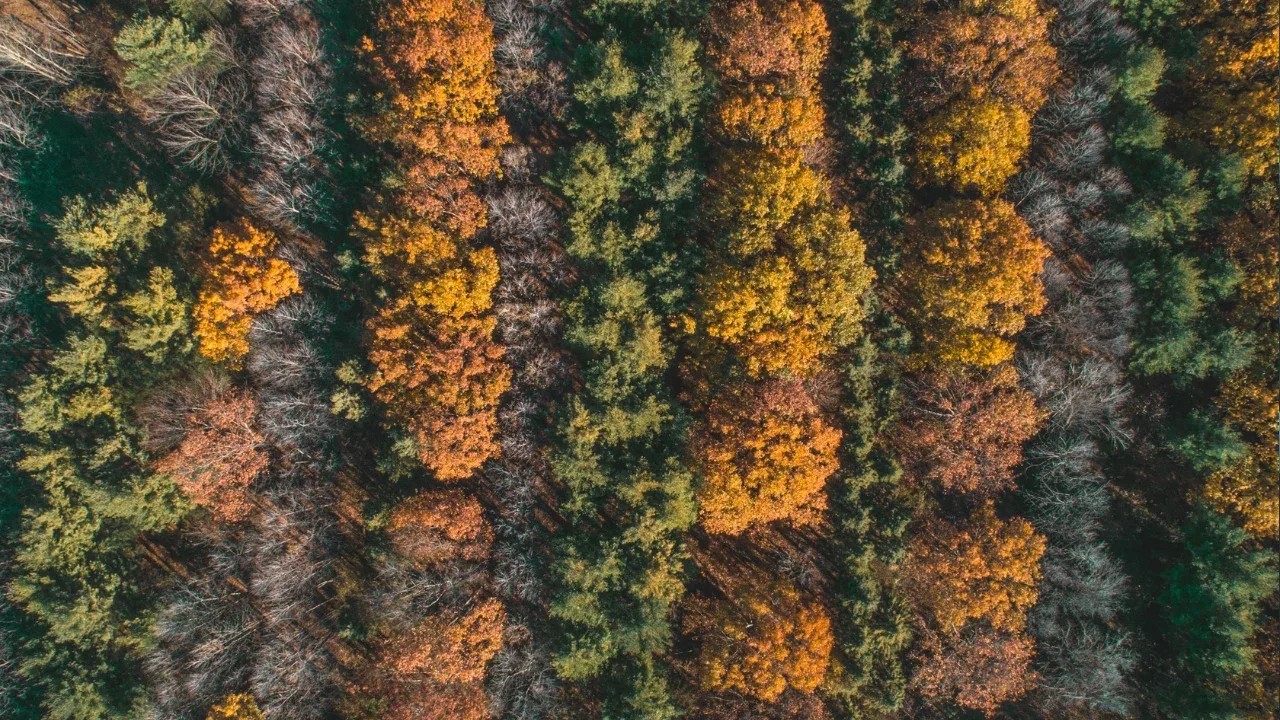
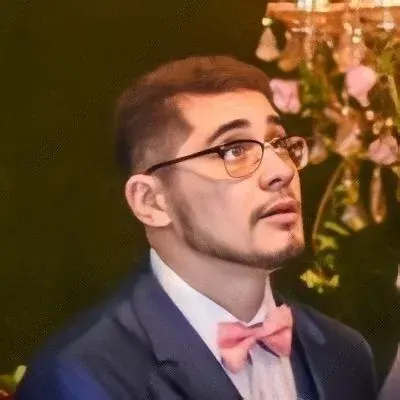
🐍 How to Declare an Array in Python: A Complete Guide 🐍
So, you're diving into the exciting world of Python and you want to know how to declare an array. You've come to the right place! In this guide, we'll walk you through everything you need to know about declaring arrays in Python. 🚀
What is an Array?
Before we jump into the how, let's start with the what. An array is a data structure that allows you to store multiple values of the same type in a single variable. Think of it as a big container that can hold many items.
Declaring an Array in Python
To declare an array in Python, we have several options. Let's explore each one:
Option 1: Using Square Brackets
The simplest and most common way to declare an array in Python is by using square brackets. Here's an example:
my_array = [1, 2, 3, 4, 5]
In this example, we've declared an array called my_array
containing five integers.
Option 2: Using the array Module
Python provides an array module that allows you to create arrays with a specific type. Here's how you can use it:
import array
my_array = array.array('i', [1, 2, 3, 4, 5])
In this example, we've imported the array
module and declared an array called my_array
containing five integers. The 'i'
argument specifies the type of the array (in this case, integer).
Option 3: Using the numpy Library
If you're working with large arrays or need advanced array manipulation capabilities, the numpy library is your best friend. Here's how you can use it:
import numpy as np
my_array = np.array([1, 2, 3, 4, 5])
In this example, we've imported the numpy library using the np
alias and declared an array called my_array
containing five integers.
Common Pitfalls and Troubleshooting
Issue 1: Forgetting to Import Modules
If you're using options 2 or 3 to declare an array, make sure you've imported the necessary modules (array
or numpy
). Forgetting this step can result in errors like NameError: name 'array' is not defined
.
Issue 2: Mixing Up Square Brackets and Parentheses
When declaring an array using the array module or the numpy library, make sure to use square brackets ([]
) to enclose the elements, not parentheses (()
). Mixing them up can lead to syntax errors.
Conclusion
Congratulations! 🎉 You now know how to declare an array in Python using different methods. Whether you choose the simple square bracket notation, the array module, or the powerful numpy library, you have the tools to handle arrays like a pro.
If you found this guide helpful, don't keep it to yourself! 📣 Share it with your friends who are also venturing into Python programming. And if you have any questions or insights to share, feel free to leave a comment below. Let's keep the Python love going!
Happy coding! 💻