How do I create test and train samples from one dataframe with pandas?
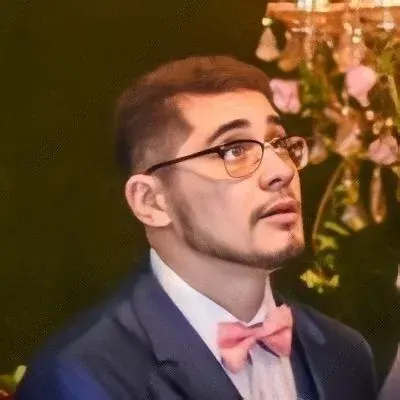
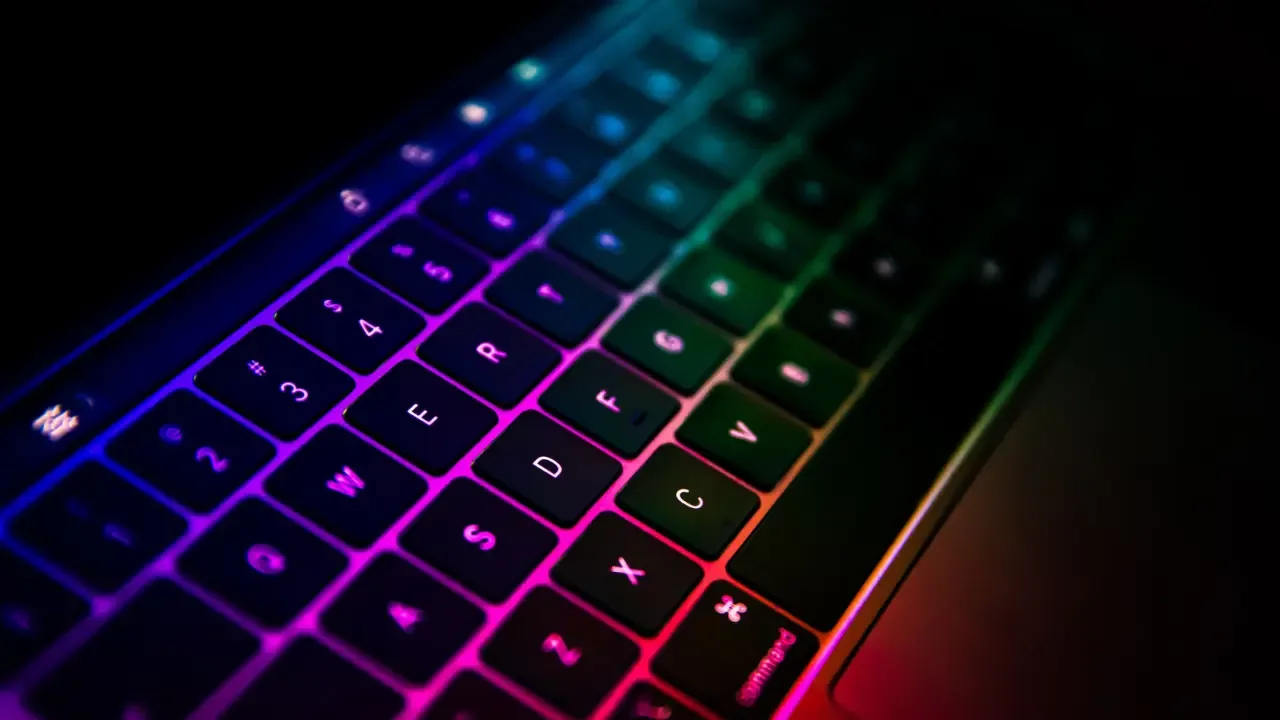
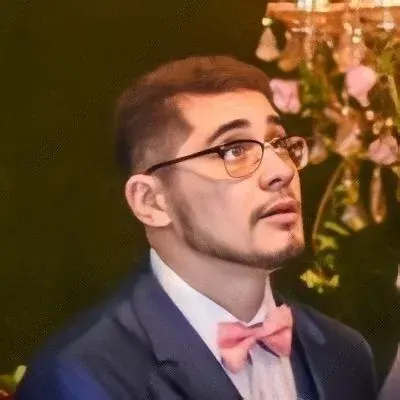
📝 How to Easily Split a DataFrame into Test and Train Samples with Pandas 💻📊
Have you ever wondered how to divide your large dataframe into random samples for training and testing purposes? 🤔 Don't worry, we've got you covered! In this blog post, we'll show you an easy and efficient way to create test and train samples using pandas. Let's dive right in! 🏊♂️💦
The Dilemma 💭
So, you have a fairly large dataset in the form of a dataframe, and you want to split it into two random samples - one for training and one for testing. It's a common scenario, especially when you're building machine learning models, where you need to assess the performance and accuracy of your model using unseen data.
The Solution 💡
To split your dataframe into test and train samples, we can utilize the power of pandas and a little bit of randomness! Here's how you can do it step-by-step:
import pandas as pd
from sklearn.model_selection import train_test_split
# Load your data into a pandas dataframe
df = pd.read_csv('your_dataset.csv')
# Split the data into features (X) and target variable (y)
X = df.drop('target_variable', axis=1)
y = df['target_variable']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Let's break down what's happening in the code:
First, we import the necessary libraries - pandas and
train_test_split
fromsklearn.model_selection
.Next, we load our dataset into a pandas dataframe using the
read_csv()
function. Replace'your_dataset.csv'
with the path or name of your dataset file.Then, we split the dataframe into features (X) and the target variable (y). In the
drop()
function, replace'target_variable'
with the column name of your target variable.Finally, we utilize the
train_test_split()
function to split the data into training and testing sets. We pass in the features (X) and target variable (y) along with the desired test size (e.g.,test_size=0.2
for an 80-20 split) and a random state for reproducibility.
And voila! 🎉 You now have two separate dataframes - X_train
and X_test
for features, as well as y_train
and y_test
for the target variable.
Take it to the Next Level 🚀
Now that you know how to split your dataframe into test and train samples, the possibilities are endless! You can use these samples to train, validate, and evaluate machine learning models, ensuring the accuracy and reliability of your predictions.
So why not give it a try? 🤓 Load your dataset, follow the steps we've provided, and explore the exciting world of machine learning!
Don't hesitate to share your results and experiences with us in the comments below. We'd love to hear how this technique has helped you in your data science journey.
Until next time, happy coding! 💻😄