How do I create multiline comments in Python?
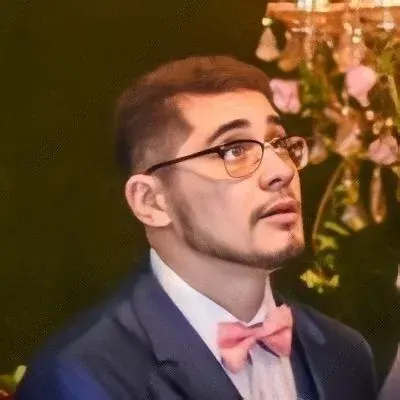
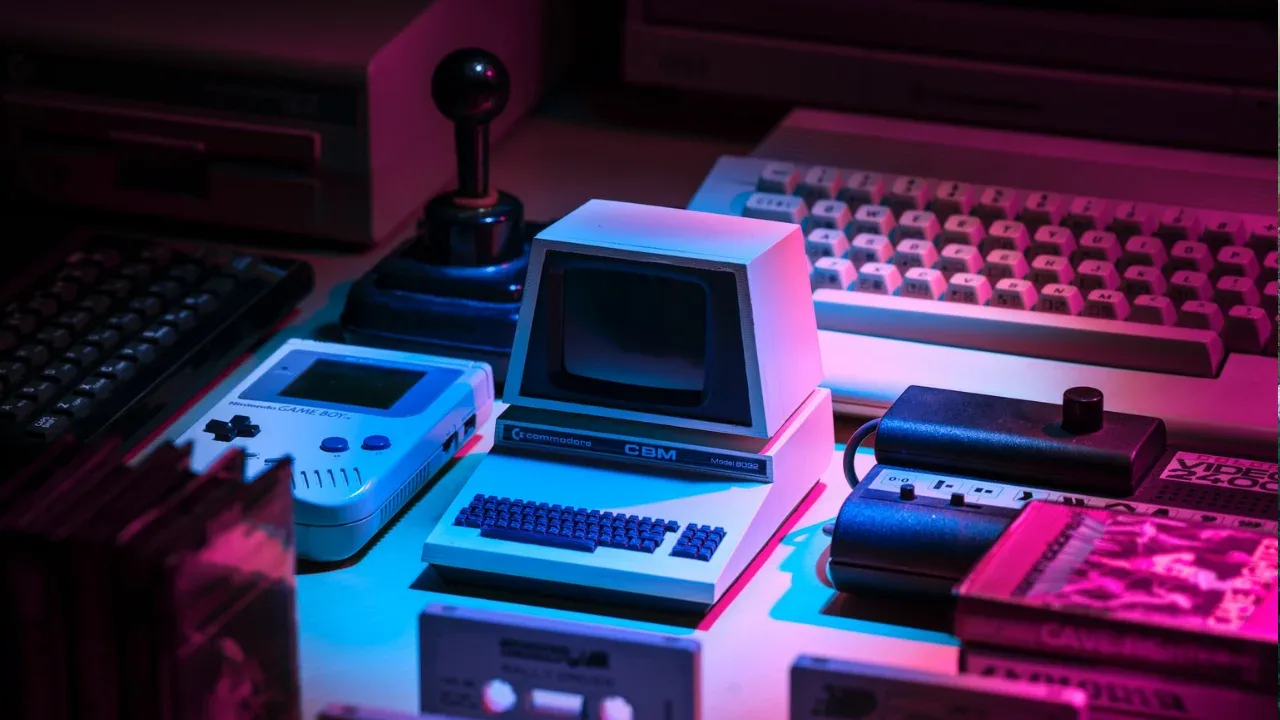
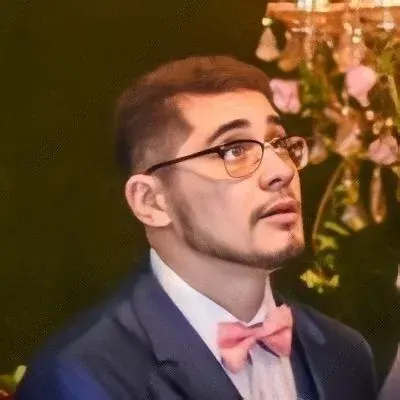
👋 Welcome to my tech blog! Today, we are going to tackle a common question that many Python beginners have: How do I create multiline comments in Python? 🐍
The Multiline Comment Challenge
So you've started your Python journey and you want to add comments to your code to explain what it does. Makes sense! But how do you add long, multiline comments that span several lines without the hassle?
The Solution 💡
In Python, we don't have a built-in syntax specifically for multiline comments like other languages do. However, fear not! There are clever workarounds available to achieve the same effect. Let me share some with you:
Method 1: Using Triple Double Quotes
One common way to create multiline comments in Python is by using triple double quotes. This method is quite straightforward:
"""
This is a multiline comment.
It can span several lines.
"""
By wrapping your comment between three sets of double quotes, Python treats it as a string literal. These triple double quotes will be ignored by the interpreter and effectively act as a multiline comment.
However, please note that this method has a crucial drawback - the comment is not completely ignored by the interpreter. It is still compiled into bytecode and can consume some memory. So, while it's useful for documenting your code, it's not recommended for large comments in performance-critical applications.
Method 2: Single Line Comments Joined by Backslash
Another way to tackle this challenge is to use single-line comments joined by a backslash. Here's how it looks:
# This is a multiline comment \
# that spans several lines
By adding a backslash at the end of each line, Python will treat the comment as a single continuous line. This technique is commonly used when you want to break long comments into multiple lines for better readability.
Method 3: Combining Single Line Comments
In situations where you just need to quickly add a multiline comment without worrying about formatting, you can use multiple single-line comments together:
# This is a multiline comment
# that spans several lines
# without using any joining characters
While this method may not be as visually appealing, it gets the job done when you're in a hurry.
The Call-to-Action 🚀
Now that you know how to create multiline comments in Python, go ahead and start commenting your code with ease! 🎉
Remember, adding helpful comments to your code improves readability and makes it easier for others (including your future self!) to understand and maintain it. So, let those comments shine and spread the love for clean, well-documented code!
If you have any questions or other Python topics you'd like me to cover, feel free to leave a comment below. Happy coding! 😄💻